concept QuTiP in category quantum computing
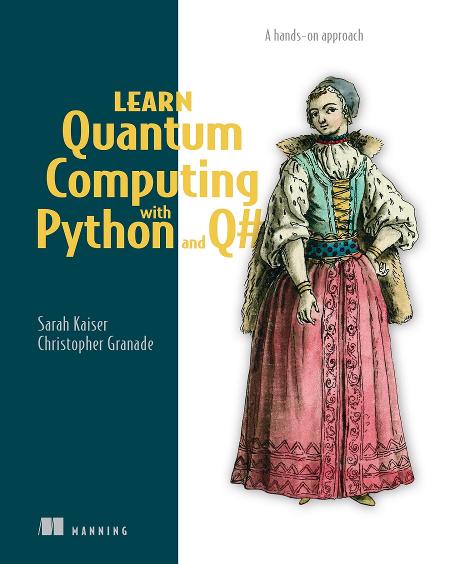
This is an excerpt from Manning's book Learn Quantum Computing with Python and Q# MEAP V08.
We will dive into a new Python package called QuTiP that will allow us to program quantum systems faster and has some cool built-in features for simulating quantum mechanics. Then you will learn how to leverage QuTiP and program a simulator for multiple qubits and see how that changes (or doesn’t!) the 3 main tasks for our qubits: state preparations, operations, and measurement.
Where QuTiP really helps out, though, is that it provides us with a lot of nice shorthand for the kinds of objects we need to work with in quantum computing. For instance, we could have also made ket0 in the above sample by using the QuTiP basis function, see .
Listing 4.11. Using built-in features of QuTiP to easily create the |0⟩ and |1⟩ states.
>>> import qutip as qt >>> ket0 = qt.basis(2, 0) #1 >>> ket0 Quantum object: dims = [[2], [1]], shape = (2, 1), type = ket #2 Qobj data = [[1.] [0.]] >>> ket1 = qt.basis(2, 1) #3 >>> ket1 Quantum object: dims = [[2], [1]], shape = (2, 1), type = ket Qobj data = [[0.] [1.]]
QuTiP also provides a function ry to represent rotating by whatever angle we like instead of 180° like the x operation. We have actually already seen the operation that ry represents in Chapter 2, when we considered rotating |0⟩ by an arbitrary angle 𝜃. See for a refresher on the operation we now know as ry.
Figure 4.6. A visualization of QuTiP function ry which corresponds to a variable rotation of 𝜃 around the 𝑌 axis of our qubit (which points directly out of the page).
![]()
Now that we have a few more single-qubit operations down, how can we easily simulate multi-qubit operations in QuTiP? We can use QuTiP’s tensor function to quickly get up and running with tensor products to make our multi-qubit registers and operations, as we show in listing 4.12.
Listing 4.12. Tensor products in QuTiP
>>> import qutip as qt >>> psi = qt.basis(2, 0) #1 >>> phi = qt.basis(2, 1) #2 >>> qt.tensor(psi, phi) Quantum object: dims = [[2, 2], [1, 1]], shape = (4, 1), type = ket Qobj data = [[ 0.] #3 [ 1.] [ 0.] [ 0.]] >>> H = qt.hadamard_transform(1) #4 >>> I = qt.qeye(2) #5 >>> qt.tensor(H, I) #6 Quantum object: dims = [[2, 2], [2, 2]], shape = (4, 4), type = oper, isherm = True Qobj data = [[ 0.70710678 0. 0.70710678 0. ] [ 0. 0.70710678 0. 0.70710678] [ 0.70710678 0. -0.70710678 0. ] [ 0. 0.70710678 0. -0.70710678]] >>> ( #7 ... qt.tensor(H, I) * qt.tensor(psi, phi) - ... qt.tensor(H * psi, I * phi) ... ) Quantum object: dims = [[2, 2], [1, 1]], shape = (4, 1), type = ket Qobj data = [[ 0.] [ 0.] [ 0.] [ 0.]]