concept AsyncStorage in category react native
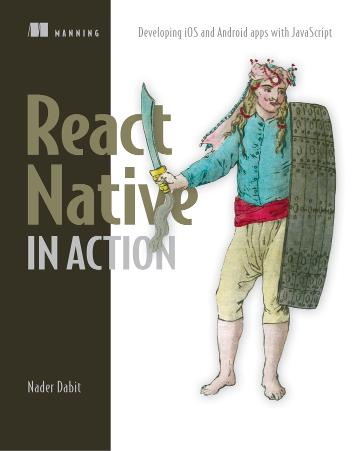
This is an excerpt from Manning's book React Native in Action.
You’re finished and should be able to run the app. Play around with the app, add cities and locations, and then refresh it. Notice that all the cities disappear when you refresh. This is because you’re only storing the data in memory. Let’s use
AsyncStorage
to persist the state, so if the user closes or refreshes the app, their data remains available.
Next up is AsyncStorage. AsyncStorage is a great way to persist and store data: it’s asynchronous, meaning you can retrieve data using a promise or
async await
, and it uses a key-value system to store and retrieve data.When you use an application and then close it, its state will be reset the next time you open it. One of the main benefits of AsyncStorage is that it lets you store the data directly to the user’s device and retrieve it whenever you need it!
9.3.2 Example of using AsyncStorage
In this example, you’ll take a user object and store it into the AsyncStorage in
componentDidMount
. You’ll then use a button to extract the data from AsyncStorage, populate the state with the data, and render it to the view.Listing 9.3 Persisting and retrieving data using AsyncStorage
import React, { Component } from 'react' import { TouchableHighlight, AsyncStorage, View, Text, StyleSheet } from 'react-native' #1 let styles = {} const person = { #2 name: 'James Garfield', age: 50, occupation: 'President of the United States' } const key = 'president' #3 export default class App extends Component { constructor () { super() this.state = { person: {} #4 } this.getPerson= this.getPerson.bind(this) } componentDidMount () { AsyncStorage.setItem(key, JSON.stringify(person)) #5 .then(() => console.log('item stored...')) .catch((err) => console.log('err: ', err)) } getPerson () { #6 AsyncStorage.getItem(key) #7 .then((res) => this.setState({ person: JSON.parse(res) })) #8 .catch((err) => console.log('err: ', err)) } render () { const { person } = this.state return ( <View style={styles.container}> <Text style={{textAlign: 'center'}}>Testing AsyncStorage</Text> <TouchableHighlight onPress={this.getPerson} style={styles.button}> #9 <Text>Get President</Text> </TouchableHighlight> <Text>{person.name}</Text> <Text>{person.age}</Text> <Text>{person.occupation}</Text> </View> ) } } styles = StyleSheet.create({ container: { justifyContent: 'center', flex: 1, margin: 20 }, button: { justifyContent: 'center', marginTop: 20, marginBottom: 20, alignItems: 'center', height: 55, backgroundColor: '#dddddd' } }) #1 Imports AsyncStorage from React Native #2 Creates a person object and stores the information in it #3 Creates a key you’ll use to add and remove data from AsyncStorage #4 Creates a person object in the state #5 Calls AsyncStorage.setItem, passing in the key as well as the person. Calls JSON.stringify because the value stored in AsyncStorage needs to be a string; JSON.stringify turns objects and arrays into strings. #6 Creates the getPerson method #7 Calls AsyncStorage.getItem, passing in the key created earlier. You receive a callback function with the data retrieved from AsyncStorage. #8 Calls JSON.parse, which turns the returned data back into a JavaScript object; and populates the state #9 Wires up getPerson to a TouchableHighlight in the view. When the TouchableHighlight is pressed, the data from AsyncStorage is rendered to the View.As you can see, promises are used to set and return the values from AsyncStorage. There’s also another way to do this: let’s look at
async await
.