concept border radius in category react native
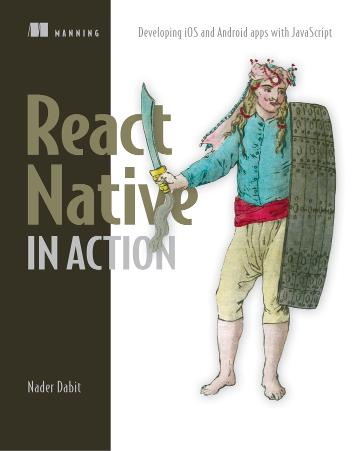
This is an excerpt from Manning's book React Native in Action.
For the color and width, there are individual properties for each side of the border:
borderTopColor
,borderRightColor
,borderBottomColor
,borderLeftColor
,borderTopWidth
,borderRightWidth
,borderBottomWidth
, andborderLeftWidth
. For the border radius, there are properties for each corner:borderTopRightRadius
,borderBottomRightRadius
,borderBottomLeftRadius
, andborderTopLeftRadius
. But there’s only oneborderStyle
.
Figure 4.6 Examples of various border radius combinations. Example 1: a square with four rounded corners. Example 2: a square with the right two corners rounded, making a D shape. Example 3: a square with the opposite corners rounded, which looks like a leaf. Example 4: a square with a border radius equal to half the length of a side, which results in a circle.
![]()
Listing 4.9 Setting various border radius combinations
import React, { Component } from 'react'; import { StyleSheet, Text, View} from 'react-native'; export default class App extends Component<{}> { render() { return ( <View style={styles.container}> <Example style={{borderRadius: 20}}> #1 <CenteredText> Example 1:{"\n"}4 Rounded Corners #2 </CenteredText> </Example> <Example style={{borderTopRightRadius: 60, borderBottomRightRadius: 60}}> #3 <CenteredText> Example 2:{"\n"}D Shape </CenteredText> </Example> <Example style={{borderTopLeftRadius: 30, borderBottomRightRadius: 30}}> #4 <CenteredText> Example 3:{"\n"}Leaf Shape </CenteredText> </Example> <Example style={{borderRadius: 60}}> #5 <CenteredText> Example 4:{"\n"}Circle </CenteredText> </Example> </View> ); } } const Example = (props) => ( <View style={[styles.example,props.style]}> {props.children} </View> ); const CenteredText = (props) => ( #6 <Text style={[styles.centeredText, props.style]}> {props.children} </Text> ); const styles = StyleSheet.create({ container: { #7 flex: 1, flexDirection: 'row', flexWrap: 'wrap', marginTop: 75 }, example: { width: 120, height: 120, marginLeft: 20, marginBottom: 20, backgroundColor: 'grey', borderWidth: 2, justifyContent: 'center' }, centeredText: { #8 textAlign: 'center', margin: 10 } }); #1 Example 1: a square with four rounded corners #2 This is JavaScript, so you can specify a hard return inline with the text by using {"\n"}. #3 Example 2: a square with the right two corners rounded #4 Example 3: a square with the opposite corners rounded #5 Example 4: a square with a border radius equal to half the length of a side #6 Reusable component for rendering the centered text elements #7 React Native uses flexbox to control layout. #8 Style that centers the text within the text components