concept screen in category react native
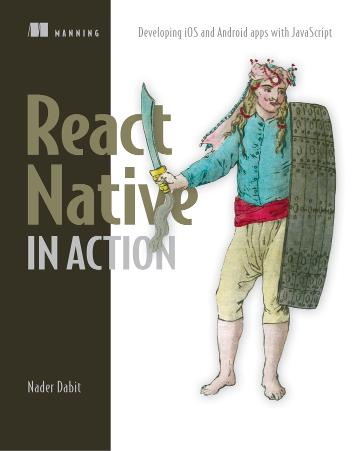
This is an excerpt from Manning's book React Native in Action.
The developer experience is a major win for React Native. If you’ve ever developed for the web, you’re aware of the browser’s snappy reload times. Web development has no compilation step: just refresh the screen, and your changes are there. This is a far cry from the long compile times of native development. One of the reasons Facebook decided to develop React Native was to overcome the lengthy compile times of the Facebook application when using native iOS and Android build tools. To make a small UI change or any other change, Facebook developers had to wait a long time while the program compiled to see the results. Long compilation times result in decreased productivity and increased developer cost. React Native solves this issue by giving you the quick reload times of the web, as well as Chrome and Safari debugging tools, making the debugging experience feel a lot like the web.
Size can be a confusing topic, but it’s important to keep in mind if you need to be absolutely precise when positioning components on the screen. Even if you’re not trying to produce a high-fidelity layout, it will be useful to understand the concepts in case you encounter small discrepancies in your layouts from one device to another.
You learned earlier in this section that the
flexDirection
property takes two values:column
(the default) androw
.column
lays out items vertically, androw
lays out items horizontally. What you haven’t seen is a situation in which items flow off the screen because they don’t fit.
flexWrap
takes two values:nowrap
andwrap
. The default value isnowrap
, meaning items will flow off the screen if they don’t fit. The items are clipped, and the user can’t see them. To work around this problem, use thewrap
value.In figure 5.21, the first example uses
nowrap
, and the squares flow off the screen. The row of squares is chopped off at the right edge. The second example useswrap
, and the squares wrap around and start a new row. Listing 5.10 shows the code.Figure 5.21 An example of two overflowing containers: one with flexWrap set to nowrap and the other with flexWrap set to wrap
![]()
Listing 5.10 Example of how
flexWrap
values affect layoutimport React, { Component } from 'react'; import { StyleSheet, Text, View} from 'react-native'; export default class App extends Component<{}> { render() { return ( <View style={styles.container}> <NoWrapContainer> #1 <Example>A nowrap</Example> <Example>1</Example> <Example>2</Example> <Example>3</Example> <Example>4</Example> </NoWrapContainer> <WrapContainer> #2 <Example>B wrap</Example> <Example>1</Example> <Example>2</Example> <Example>3</Example> <Example>4</Example> </WrapContainer> </View> ); } } const NoWrapContainer = (props) => ( <View style={[styles.noWrapContainer,props.style]}> #3 {props.children} </View> ); const WrapContainer = (props) => ( <View style={[styles.wrapContainer,props.style]}> #4 {props.children} </View> ); const Example = (props) => ( <View style={[styles.example,props.style]}> <Text> {props.children} </Text> </View> ); const styles = StyleSheet.create({ container: { marginTop: 150, flex: 1 }, noWrapContainer: { backgroundColor: '#ededed', flexDirection: 'row', #5 flexWrap: 'nowrap', borderWidth: 1, margin: 10 }, wrapContainer: { backgroundColor: '#ededed', flexDirection: 'row', #6 flexWrap: 'wrap', borderWidth: 1, margin: 10 }, example: { width: 100, height: 100, margin: 5, backgroundColor: '#666666' }, }); #1 flexWrap is set to nowrap: the squares overflow off the screen. #2 flexWrap is set to wrap: the row of squares wraps around to start a new line. #3 Uses the noWrapContainer style for the first example #4 Uses the wrapContainer style for the second example #5 Sets flexDirection to row and flexWrap to nowrap #6 Sets flexDirection to row and flexWrap to wrap !@%STYLE%@! {"css":"{\"css\": \"font-weight: bold;\"}","target":"[[{\"line\":27,\"ch\":18},{\"line\":27,\"ch\":40}],[{\"line\":33,\"ch\":18},{\"line\":33,\"ch\":38}],[{\"line\":53,\"ch\":8},{\"line\":53,\"ch\":29}],[{\"line\":60,\"ch\":8},{\"line\":60,\"ch\":29}],[{\"line\":54,\"ch\":8},{\"line\":54,\"ch\":27}],[{\"line\":53,\"ch\":8},{\"line\":53,\"ch\":29}],[{\"line\":60,\"ch\":8},{\"line\":60,\"ch\":29}],[{\"line\":61,\"ch\":8},{\"line\":61,\"ch\":25}]]"} !@%STYLE%@!