concept shadow in category react native
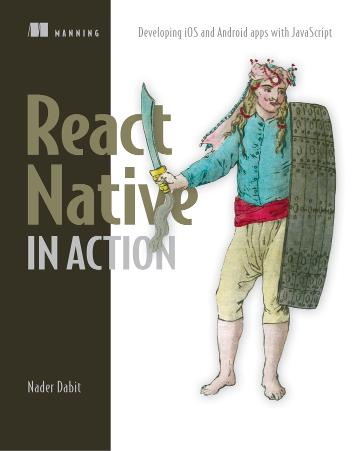
This is an excerpt from Manning's book React Native in Action.
Adding shadows to text
Listing 4.20 Completed Profile Card example
import React, { Component } from 'react'; import { Image, StyleSheet, Text, View} from 'react-native'; export default class App extends Component<{}> { render() { return ( <View style={styles.container}> <View style={styles.cardContainer}> <View style={styles.cardImageContainer}> <Image style={styles.cardImage} source={require('./user.png')}/> </View> <View> <Text style={styles.cardName}> John Doe </Text> </View> <View style={styles.cardOccupationContainer}> <Text style={styles.cardOccupation}> React Native Developer </Text> </View> <View> <Text style={styles.cardDescription}> John is a really great JavaScript developer. He loves using JS to build React Native applications for iOS and Android. </Text> </View> </View> </View> ); } } const profileCardColor = 'dodgerblue'; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center' }, cardContainer: { alignItems: 'center', borderColor: 'black', borderWidth: 3, borderStyle: 'solid', borderRadius: 20, backgroundColor: profileCardColor, width: 300, height: 400 }, cardImageContainer: { alignItems: 'center', backgroundColor: 'white', borderWidth: 3, borderColor: 'black', width: 120, height: 120, borderRadius: 60, marginTop: 30, paddingTop: 15 }, cardImage: { width: 80, height: 80 }, cardName: { color: 'white', fontWeight: 'bold', fontSize: 24, marginTop: 30, textShadowColor: 'black', #1 textShadowOffset: { #2 height: 2, width: 2 }, textShadowRadius: 3 #3 }, cardOccupationContainer: { borderColor: 'black', borderBottomWidth: 3 }, cardOccupation: { fontWeight: 'bold', marginTop: 10, marginBottom: 10, }, cardDescription: { fontStyle: 'italic', marginTop: 10, marginRight: 40, marginLeft: 40, marginBottom: 10 } }); #1 Sets the shadow color to black on the Title text component #2 Sets the shadow offset to be down and to the right #3 Sets the shadow radius
Figure 5.2 iOS-specific examples of how to apply ShadowPropTypesIOS styles to View components. Example 1 has a shadow applied but no opacity set, which causes the drop shadow to not be displayed. Example 2 has the same shadow effect but with opacity set to 1. Example 3 has a slightly larger shadow, and example 4 has the same size shadow with a shadow radius. Example 5 has the same shadow size, but opacity is changed from 1 to 0.2. Example 6 changes the color of the shadow. Example 7 shows the shadow applied in only one direction, and example 8 shows the shadow applied in the opposite direction.
![]()