concept color in category react
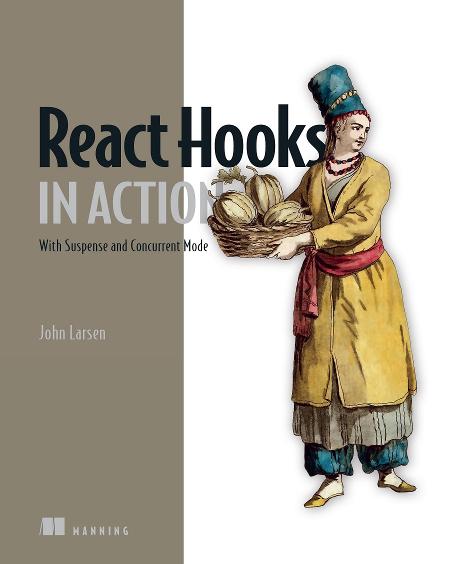
This is an excerpt from Manning's book React Hooks in Action MEAP 04.
Clicking a color in the list (one of the circles) highlights that selection and updates the text and the color bar. You can see the component in action on codesandbox (https://hgt0x.csb.app/).
Figure 7.1 The Colors component. When a user selects a color, the menu, text and color bar all update.
![]()
It’s a simple example but illustrates the basic concepts nicely. Also, we return to the
Colors
component in chapter 9 when we use the Context API and the useContext hook to share the selected color and the color-selection updater function.
Both the
ColorChoiceText
component and theColorSample
component display the currently selected color.ColorChoiceText
includes it in its message andColorSample
uses it to set the background color. They receive the color value from theColors
component, as shown in figure 7.2.Figure 7.2 The Colors component passes the current color state value to the child components.
![]()
Colors
is the closest shared parent of the child components that share the state, so we manage the state withinColors
. Figure 7.3 shows theColorChoiceText
component displaying a message that includes the selected color. The component simply uses the color value as part of its UI; it doesn’t need to update the value.Figure 7.3 The ColorChoiceText component includes the selected color in its message.
![]()
The
ColorChoiceText
component’s code is in listing 7.2. When React calls the component, it passes it, as the component’s first argument, an object containing all of the props set by the parent. The code here destructures the props, assigning thecolor
prop to a local variable of the same name.
The destructuring syntax includes a default value for
colors
:{colors = [], color, setColor}The
ColorPicker
component iterates over thecolors
array to create a list item for each available color. Using an empty array as a default value causes the component to return an empty unordered list if the parent component doesn’t set thecolors
prop.More interesting (for a book about React hooks) are the
color
andsetColor
props. These props have come from a call touseState
in the parent.const [color, setColor] = useState(availableColors[0]);The
ColorPicker
doesn’t care where they’ve come from, it just expects acolor
prop to hold the current color and asetColor
prop to be a function it can call to set the color somewhere.ColorPicker
uses thesetColor
updater function in theonClick
handler for each list item. By calling thesetColor
function, the child component,ColorPicker
, is able to set the state for the parent component,Colors
. The parent then re-renders, updating all of its children with the newly selected color.We created the
Colors
component from scratch, knowing we needed shared state to pass down to child components. Sometimes we work with existing components and, as a project develops, realize they hold state that other siblings may also need. The next sections look at a couple of ways of lifting state up from children to parents to make it more widely available.