concept sub in category ruby
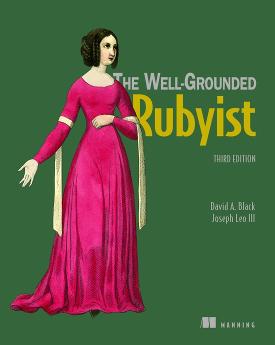
This is an excerpt from Manning's book The Well-Grounded Rubyist, Third Edition.
Dangerous can mean whatever the person writing the method wants it to mean. In the case of the built-in classes, it usually means this method, unlike its nonbang equivalent, permanently modifies its receiver. It mutates the state of the caller. (We’ll dive much more deeply into mutable versus immutable code in chapter 16.) It doesn’t always mean this though: exit! is a dangerous alternative to exit, in the sense that it doesn’t run any finalizers on the way out of the program. The danger in sub! (a method that substitutes a replacement string for a matched pattern in a string) is partly that it changes its receiver and partly that it returns nil if no change has taken place—unlike sub, which always returns a copy of the original string with the replacement (or no replacement) made.
sub and gsub (along with their bang, in-place equivalents) are the most common tools for changing the contents of strings in Ruby. The difference between them is that gsub (global substitution) makes changes throughout a string, whereas sub makes at most one substitution.
gsub is like sub, except it keeps substituting as long as the pattern matches anywhere in the string. For example, here’s how you can replace the first letter of every word in a string with the corresponding capital letter:
>> "capitalize every word".gsub(/\b\w/) {|s| s.upcase } => "Capitalize Every Word"As with sub, gsub gives you access to the $n parenthetical-capture variables in the code block.