concept observable in category rxjs
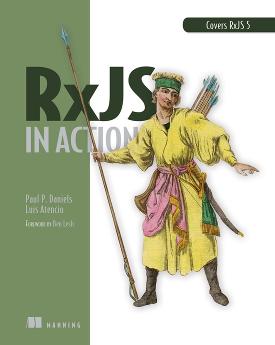
This is an excerpt from Manning's book RxJS in Action.
Producers are the sources of your data. A stream must always have a producer of data, which will be the starting point for any logic that you’ll perform in RxJS. In practice, a producer is created from something that generates events independently (anything from a single value, an array, mouse clicks, to a stream of bytes read from a file). The observer pattern defines producers as the subject; in RxJS, we call them observables, as in something that’s able to be observed.
Observables are in charge of pushing notifications, so we refer to this behavior as fire-and-forget, which means that we’ll never expect the producer to be involved in the processing of events, only the emission of them.
A lazy subscription means that an observable will remain in a dormant state until it’s activated by an event that it finds interesting. Consider this example that again generates an infinite number of events separated by half a second:
const source$ = Rx.Observable.create(observer => { let i = 0; setInterval(() => { observer.next(i++); #1 }, 500); });The cost of allocating an observable instance is fixed, unlike an array, which is a dynamic object with potential unbounded growth. It must be this way; otherwise, it would be impossible to store each user’s clicks, key presses, or mouse moves. To activate source$, an observer must first subscribe to it via subscribe(). A call to subscribe will take the observable out of its dormant state and inform it that it can begin producing values—in this case, starting the allocation for events 1, 2, 3, 4, 5, and so on every half-second. Because an observable is an abstraction over many different data sources, the effect will vary from source to source.
In RxJS, you want to map functions across all the elements emitted from an observable. To help you better visualize operators, we’ll use the marble diagrams. Recall that arrows and symbolic characters represent the various operations that convert the input stream into the output stream, as shown in figure 3.5.