concept partial function in category scala
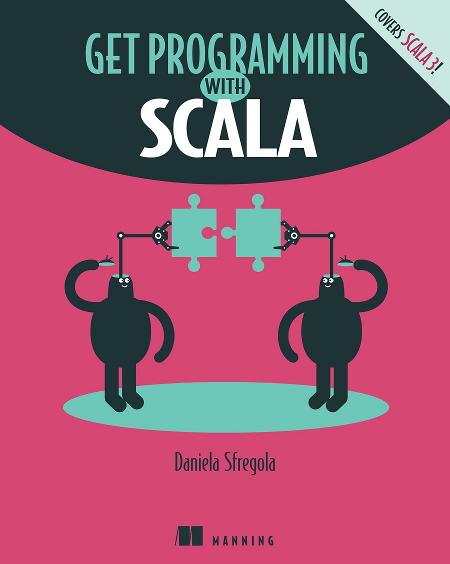
This is an excerpt from Manning's book Get Programming with Scala MEAP V07.
You’ll learn about try-catch statements in detail in lesson 17, in which you are also going to discover to partial functions. In unit 6, you’ll master how to handle errors without throwing exceptions, but using a more functional and safe approach that uses types.
After learning about pattern matching, in this lesson, you’ll discover partial functions and how they relate to pattern matching. Partial functions are functions that are defined only for some input. You’ll see how they can be useful to abstract commonalities between functions and how you can compose them to create more complex functionalities. Finally, you’ll see how you can use partial functions to catch and handle exceptions. In the capstone, you’ll use partial functions to define the routes of your HTTP server.
16.1.1 Implementing a Partial Function
A partial function is a function that you can define only for some instances of a type. You have already encountered examples of partial functions when discussing pattern matching: you can consider each case statement as a partial function. For example, you can define a partial function to compute the square root of a non-negative integer as follows:
Listing 16.2: The sqrt partial function
val sqrt: PartialFunction[Int, Double] = //#A { case x if x >= 0 => Math.sqrt(x) }You can view partial functions as a particular anonymous function that has one or more case statements as their body. The compiler cannot infer their type, so you need to specify its argument and return types: in Scala, the type PartialFunction[A, B] identifies a partial function that a parameter of type A and that returns an instance of type B.
Listing 16.3 shows another example of partial function:
Listing 16.3: A toPrettyString function
val toPrettyString: PartialFunction[Any, String] = { //#A case x: Int if x > 0 => s"positive number: $x" case s: String => s }After you define a partial function, you can perform function calls using the same syntax you have learned for anonymous functions. But do not forget that your function is now partial: if your input doesn’t match any of its case statement, you will receive a MatchError exception at runtime:
scala> :paste // Entering paste mode (ctrl-D to finish) val toPrettyString: PartialFunction[Any, String] = { case x: Int if x > 0 => s"positive number: $x" case s: String => s } // Exiting paste mode, now interpreting. toPrettyString: PartialFunction[Any,String] = <function1> scala> toPrettyString(1) res0: String = positive number: 1 scala> toPrettyString("hello") res1: String = hello scala> toPrettyString(-1) scala.MatchError: -1 (of class java.lang.Integer) at scala.PartialFunction$$anon$1.apply(PartialFunction.scala:255) at scala.PartialFunction$$anon$1.apply(PartialFunction.scala:253) at $anonfun$1.applyOrElse(<pastie>:14) at scala.runtime.AbstractPartialFunction.apply(AbstractPartialFunction.scala:34) ... 28 elidedFigure 16.1 summarizes how to implement partial functions in Scala.
Figure 16.1: Syntax diagram on how to implement partial functions in Scala. Do not forget to fully specify the types of your partial function using PartialFunction[A, B]: A is the type of your parameter, while B is its return type.
![]()
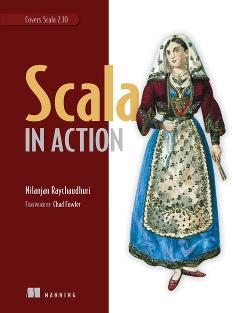
This is an excerpt from Manning's book Scala in Action.
A partial function is a function that’s only defined for a subset of input values. This is different from the definition of a pure function (see section 5.1), which is defined for all input parameters. Figure 5.3 shows a partial function f: X -> Y which is only defined for X=1 and X=3, not X=2.