concept DataSource in category spring
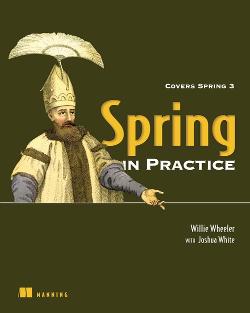
This is an excerpt from Manning's book Spring in Practice.
Consider the relationship between a data access object (DAO) and the DataSource it relies on in the following code sample. For the DAO to work with the DataSource, you need to create and initialize the DataSource with various connection parameters within the JdbcAccountDao class:
In this code sample, JdbcAccountDao specifies a dependency on a JDBC DataSource. Coding to interfaces is certainly a best practice. As shown in figure 1.3, the code also specifically creates a dependency on a BasicDataSource, a specific DataSource implementation from the Apache Commons Database Connection Pool (DBCP) project.
Figure 1.3. JdbcAccountDao specifies a dependency on Apache DBCP’s BasicDataSource, which is a concrete DataSource implementation.
![]()
An obvious problem here is that the JdbcAccountDao class is intimately aware of the DataSource’s implementation, creation, and configuration. Another potential problem is that it’s likely that many DAOs may need to share this connection information. As a result of the current design, changing the DataSource’s implementation or configuration may involve multiple code changes, recompilations, and redeployments every time the DataSource implementation or configuration changes.
You configure a JDBC DataSource at
. A DataSource is essentially a factory for database connections. As such, DataSources are an important part of most web and enterprise applications. Here you’re using the Apache Commons Database Connection Pool (DBCP) implementation, BasicDataSource, which pools connections. You specify a bean ID and class, as usual. The optional destroy-method attribute tells Spring to call DataSource.close() upon shutting down the Spring container, which releases all pooled Connections. Finally, you have the standard driverClassName, url, username, and password attributes.
Note that in this configuration, you create and manage the DataSource from the app itself, rather than looking up a container-managed DataSource.[2] See figure 2.2.
2 You’ll learn how to use a container-managed DataSource in recipe 2.2.
Now that you have a DataSource, you can create the JDBC operations object. The specific implementation is NamedParameterJdbcTemplate
. You pass the DataSource into the constructor using Spring’s constructor namespace.
You can address the issues we raised in the discussion of recipe 2.1 by adopting a centralized approach to configuring the DataSource. Instead of having each application manage its database configuration, you can configure the DataSource inside the container and then have the applications point to that shared configuration. In addition to streamlining the configuration, this allows you to share a single connection pool across applications.
In this configuration, the application container manages the DataSource, and the app makes a JNDI call to get a reference to it. See figure 2.3.
We assume that you’ve already configured your application server to expose your DataSource through JNDI. If not, please consult the documentation for your app server for more information. The sample code for most of the chapters in the book includes a /sample_conf/jetty-env.xml file that shows how to do it for Jetty.
To do a namespace-based JNDI DataSource lookup, you need to declare the jee namespace[3] in your app context and include the jee:jndi-lookup element. The following listing shows how to do this.