concept Redis in category spring
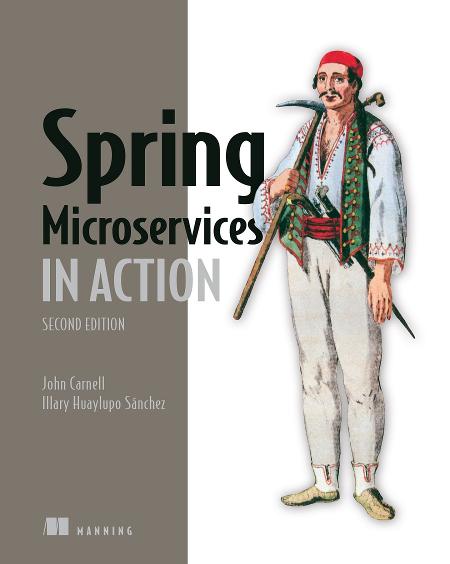
This is an excerpt from Manning's book Spring Microservices in Action, 2E MEAP V07.
Your microservices should always be stateless, by stateless it means that it doesn’t store data between sessions on the server. Microservices can be killed and replaced at any time without the fear that a loss-of-a-service instance will result in data loss. If there is a specific requirement to store a state, it has to be done through an in-memory cache such as Redis or a backing database. Figure 2.9 shows how stateless microservices work.
For our organization data cache, we’re going to use Redis (https://redis.io/) a distributed key-value store database. Figure 10.1 provides a high-level overview of how to build a caching solution using a traditional synchronous, request-response programming model.
Figure 10.1 In a synchronous request-response model, tightly coupled services introduce complexity and brittleness.
![]()
In figure 10.1, when a user calls the licensing service, the licensing service will also need to look up the organization data. The licensing service will first check to retrieve the desired organization by its ID from the Redis cluster. If the licensing service can’t find the organization data, it will call the organization service using a REST-based endpoint and then store the data returned in Redis, before returning the organization data back to the user. Now, if someone updates or deletes the organization record using the organization service’s REST endpoint, the organization service will need to call an endpoint exposed on the licensing service, telling it to invalidate the organization data in its cache. In figure 10.1, if we look at where the organization service calls back into the licensing service to tell it to invalidate the Redis cache, we can see at least three problems:
Now that we have the dependencies in Maven, we need to establish a connection out to our Redis server. Spring uses the open-source project
Jedis
(https://github.com/xetorthio/jedis) to communicate with a Redis server. To communicate with a specific Redis instance, we're going to expose aJedisConnectionFactory
in the/licensing-service/src/main/java/com/optimagrowth/license/LicenseServiceApplication.java
class as a Spring Bean. Once we have a connection out to Redis, we're going to use that connection to create a SpringRedisTemplate
object. TheRedisTemplate
object will be used by the Spring Data repository classes that we'll implement shortly to execute the queries and saves of organization service data to our Redis service. The following code listing 10.11 shows this code.Listing 10.11 Establishing how our licensing service will communicate with Redis
package com.optimagrowth.license; import org.springframework.data.redis.connection.RedisPassword; import org.springframework.data.redis.connection.RedisStandaloneConfiguration; import org.springframework.data.redis.connection.jedis.JedisConnectionFactory; import org.springframework.data.redis.core.RedisTemplate; //Most of the imports and annotations have been remove for conciseness @SpringBootApplication @EnableBinding(Sink.class) public class LicenseServiceApplication { @Autowired private ServiceConfig serviceConfig; //All other methods in the class have been removed for consiceness @Bean #A JedisConnectionFactory jedisConnectionFactory() { String hostname = serviceConfig.getRedisServer(); int port = Integer.parseInt(serviceConfig.getRedisPort()); RedisStandaloneConfiguration redisStandaloneConfiguration = new RedisStandaloneConfiguration(hostname, port); return new JedisConnectionFactory(redisStandaloneConfiguration); } @Bean #B public RedisTemplate<String, Object> redisTemplate() { RedisTemplate<String, Object> template = new RedisTemplate<>(); template.setConnectionFactory(jedisConnectionFactory()); return template; } //Rest of the code removed for conciseness }The foundational work for setting up the licensing service to communicate with Redis is complete. Let’s now move over to writing the logic that will get, add, update, and delete data from Redis.
Redis is a key-value store data store that acts like a big, distributed, in-memory HashMap. In the simplest case, it stores data and looks up data by a key. It doesn’t have any sophisticated query language to retrieve data. Its simplicity is its strength and one of the reasons why so many projects have adopted it for use in their projects.
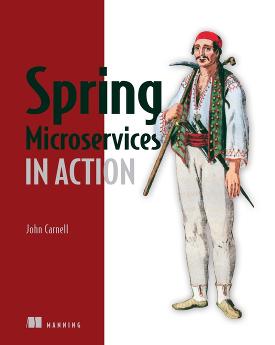
This is an excerpt from Manning's book Spring Microservices in Action.
For your organization data cache, you’re going to use Redis (http://redis.io/), a distributed key-value store database. Figure 8.1 provides a high-level overview of how to build a caching solution using a traditional synchronous, request-response programming model.
Figure 8.1. In a synchronous request-response model, tightly coupled services introduce complexity and brittleness.
![]()
In figure 8.1, when a user calls the licensing service, the licensing service will need to also look up organization data. The licensing service will first check to retrieve the desired organization by its ID from the Redis cluster. If the licensing service can’t find the organization data, it will call the organization service using a REST-based endpoint and then store the data returned in Redis, before returning the organization data back to the user. Now, if someone updates or deletes the organization record using the organization service’s REST endpoint, the organization service will need to call an endpoint exposed on the licensing service, telling it to invalidate the organization data in its cache. In figure 8.1, if you look at where the organization service calls back into the licensing service to tell it to invalidate the Redis cache, you can see at least three problems:
Now that you have the dependencies in Maven, you need to establish a connection out to your Redis server. Spring uses the open source project Jedis (https://github.com/xetorthio/jedis) to communicate with a Redis server. To communicate with a specific Redis instance, you’re going to expose a JedisConnection-Factory in the licensing-service/src/main/java/com/thoughtmechanix/licenses/Application.java class as a Spring Bean. Once you have a connection out to Redis, you’re going to use that connection to create a Spring RedisTemplate object. The RedisTemplate object will be used by the Spring Data repository classes that you’ll implement shortly to execute the queries and saves of organization service data to your Redis service. The following listing shows this code.
package com.thoughtmechanix.licenses; //Most of th imports have been remove for conciseness import org.springframework.data.redis.connection.jedis.JedisConnectionFactory; import org.springframework.data.redis.core.RedisTemplate; @SpringBootApplication @EnableEurekaClient @EnableCircuitBreaker @EnableBinding(Sink.class) public class Application { @Autowired private ServiceConfig serviceConfig; //All other methods in the class have been removed for consiceness @Bean #1 public JedisConnectionFactory jedisConnectionFactory() { JedisConnectionFactory jedisConnFactory = new JedisConnectionFactory(); jedisConnFactory.setHostName( serviceConfig.getRedisServer() ); jedisConnFactory.setPort( serviceConfig.getRedisPort() ); return jedisConnFactory; } @Bean #2 public RedisTemplate<String, Object> redisTemplate() { RedisTemplate<String, Object> template = new RedisTemplate<String, Object>(); template.setConnectionFactory(jedisConnectionFactory()); return template; } }
Redis is a key-value store data store that acts like a big, distributed, in-memory HashMap. In the simplest case, it stores data and looks up data by a key. It doesn’t have any kind of sophisticated query language to retrieve data. Its simplicity is its strength and one of the reasons why so many projects have adopted it for use in their projects.