Chapter 10. Managing data
In this chapter
- The lifecycle and states of objects
- Working with the Java Persistence API
- Working with detached state
You now understand how Hibernate and ORM solve the static aspects of the object/relational mismatch. With what you know so far, you can create a mapping between Java classes and an SQL schema, solving the structural mismatch problem. For a reminder of the problems you’re solving, see section 1.2.
An efficient application solution requires something more: you must investigate strategies for runtime data management. These strategies are crucial to the performance and correct behavior of your applications.
In this chapter, we discuss the life cycle of entity instances—how an instance becomes persistent, and how it stops being considered persistent—and the method calls and management operations that trigger these transitions. The JPA EntityManager is your primary interface for accessing data.
Before we look at the API, let’s start with entity instances, their life cycle, and the events that trigger a change of state. Although some of the material may be formal, a solid understanding of the persistence life cycle is essential.
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
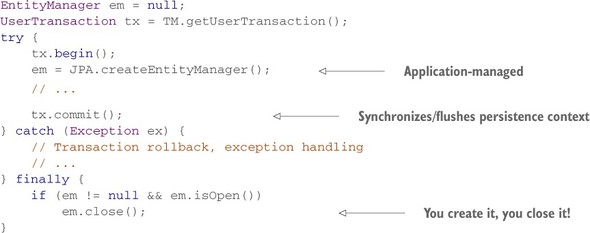
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java

Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java

Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java

Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
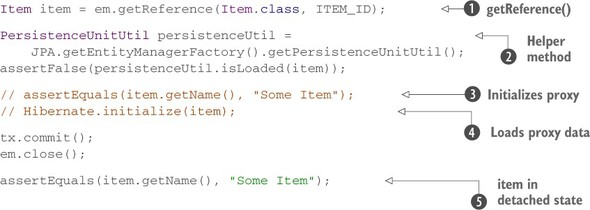
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
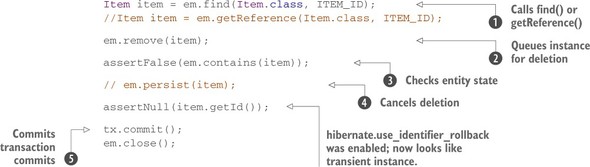
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
Path: /examples/src/test/java/org/jpwh/test/fetching/ReadOnly.java
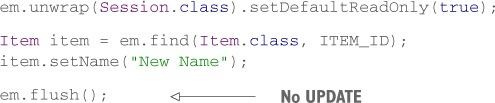
Path: /examples/src/test/java/org/jpwh/test/fetching/ReadOnly.java
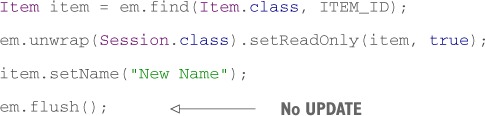
Path: /examples/src/test/java/org/jpwh/test/fetching/ReadOnly.java
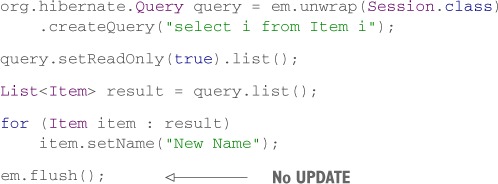
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
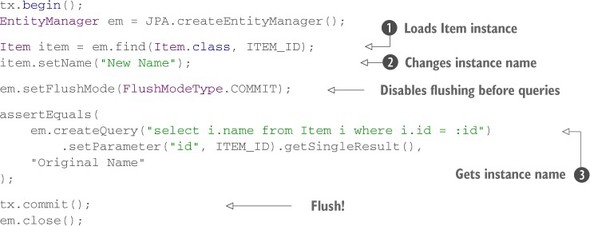
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
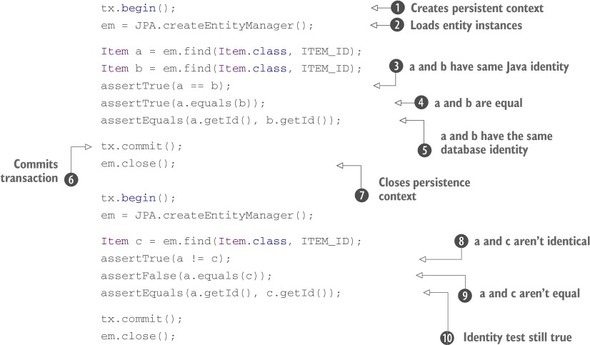
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
Path: /examples/src/test/java/org/jpwh/test/simple/SimpleTransitions.java
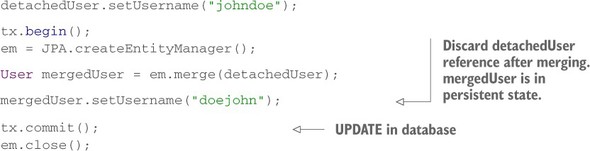