Chapter 12. Stateful programs and stateful computations
This chapter covers
- What makes a program stateful?
- Writing stateful programs without mutating state
- Generating random structures
- Composing stateful computations
Since chapter 1, I’ve been preaching against state mutation as a side effect that should be avoided at almost any cost, and you’ve seen several examples of refactoring programs to avoid state mutation. In this chapter you’ll see how the functional approach works when there’s a requirement for the program to be stateful.
But what’s a stateful program exactly? It’s a program whose behavior differs, depending on past inputs or events.[1] By analogy, if somebody says “Good morning,” you’ll probably mindlessly greet them in return. If that person immediately says “Good morning” again, your reaction will certainly differ: why in the world would somebody say “Good morning” twice in a row? A stateless program, on the other hand, would keep answering “Good morning” just as mindlessly as before, because it has no notion of past inputs. Every time is like the first time.
1This means that a program may be considered stateful/stateless depending on where you draw the program boundary. You may have a stateless server that uses a DB to keep state. If you consider both as one program, it’s stateful; if you consider the server in isolation, it’s stateless.
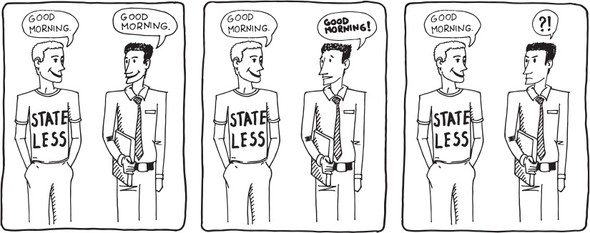