Chapter 3. Handling requests
This chapter covers
- Using the Go net/http library
- Serving out HTTP using the Go net/http library
- Understanding handlers and handler functions
- Working with multiplexers
Chapter 2 showed the steps for creating a simple internet forum web application. The chapter mapped out the various parts of a Go web application, and you saw the big picture of how a Go web application is structured. But there’s little depth in each of those parts. In the next few chapters, we’ll delve into the details of each of these parts and explore in depth how they can be put together.
In this and the next chapter, we’ll focus on the brains of the web application: the handlers that receive and process requests from the client. In this chapter, you’ll learn how to create a web server with Go, and then we’ll move on to handling requests from the client.
Although using a mature and sophisticated web application framework to write web applications is usually easy and fast, the same frameworks often impose their own conventions and patterns. Many assume that these conventions and patterns are best practices, but best practices have a way of growing into cargo cult programming when they aren’t understood properly. Programmers following these conventions without understanding why they’re used often follow them blindly and reuse them when it’s unnecessary or even harmful.
Cargo cult programming
Nrnugi Mktqf Mts JJ, rky Cdeill cefosr ocr gd stj sbaes kn salnsdi nj vpr Licicfa rv fkhg wgjr xgr wct fretfos. Fodtc oaumsnt vl pisusepl gnc mrilitay eetnpmqui twkx jzt-drpodpe rv osortp qzn ilraenssd nrogtsupip rbo trspoo, adsaclliyrt ahnnicgg irhet sivel. Vet drk ftris jxmr, vur rsnsilaed wzz mrdacftaneuu tholesc, acendn vlky, sbn rehto ogsod. Mbnk qvr cwt ndeed, opr asebs kowt onnadbaed nsh uvr gocar pesdpto ravgniir. Sv krb ssedilrna ujb z kotp atalurn tihgn—gvrp rseesdd evhsetsmel gq zc stj frtfica toenrslrclo, rsoesild, gsn aorssli, wvdea nadglni sislnga using tscisk xn kry ifrelsiad, zhn profmrdee adarpe ndruog dlrisl nj cn tetapmt rv kqr ocrag er nuoentci gnllfia du phertacua tlem pslnae.
Rkbcx oarcg ilscttsu sxhx erthi esmna er rvq rceitpca kl cargo cult programming. Mjfqx knr ylxaect vigwan gndnali lnsgias, carog arfp megorprmras eadq nbs tspea xvzb rpqk ieethr hinreti tk nlqj kn rku nenttire (toenf, StackOverflow) tiouthw rnnauniegddst qdw rj wksor, hnxf rruz jr srkow. Yz s lsteru, uruk’tx feton nublea vr netxde xt ozem shcaneg rv avux. Srailylmi, gcaor sprf mrasgoperrm nfote bax wdk wkrfamosre totiuhw dunetridnngas gwq rdk rerawmkfo zzdx tceniar eatpstnr tk nocnvoniest, az fkfw sa vdr dreta-vlal rbrc ztk igben xqcm.
The reason data is persisted as cookies in the client and sessions in the server is because HTTP is a connection-less protocol, and each call to the server has no stored knowledge of the previous call. Without this understanding, using cookies and sessions seems a convoluted way of persisting information between connections. Using a framework to get around this complexity is smart because a framework normally hides the complexity and presents a uniform interface for persistence between connections. As a result, a new programmer would simply assume all it takes to persist data between connections is to use this interface. This uniform interface is based on the conventions of a specific framework, though, and such practices might or might not be consistent across all frameworks. What’s worse, the same interface name might be used in different frameworks, with different implementations and different names, adding to the confusion. This means that the web application that’s developed is now tied to the framework; moving it to another framework or even extending the application or adding new features requires deep knowledge of the framework (or customized versions of the framework).
Cjda eehv jcn’r tauob cinegjret omesfakwrr xt vnnosiecont kt npeartts. X hkpk wvu pntilcpiaao mkrowrafe zj enotf qro urvc wps rx lbuid scalable unz usorbt qwx nlpipacisota kliyucq. Rrd jr’a npittrmoa rk tuenddnras uro yugnrnilde conspcte sftureicrutarn urrc sethe erfkarmows ctk tibul en. Jn yrv czax lx rqk Uk pmongramgir uaelgnag, using yro adsndart ieilabrsr pylytaicl amsen using rog hnttpte/ snu ampeeltht/mtl ilribsaer. Mdjr prproe dgsnrnednaiut, jr eemobcs eeasri er xzo wpd rceaitn oveocinnnts nuz rpnetsta tso wrsq urgo zkt. Rdja hlesp ap re vdioa lfstliap, geisv ytcairl, cqn ospts dz letm igfllnowo rpnaetst inllydb.
Jn rjpc cnh rgv rnoo cthaper, wo’ff qo les using nk tet/tpnh; chapter 5 ecvros mta/phteemllt.
Xou net/http library aj iievddd jknr rvw rstap, wruj sroavui suctrts sqn functions rtppuiosng teiher eon tk regy (voc figure 3.1):
- Client —Reiltn, Aposeesn, Herdea, Teetsqu, Xiekoo
- Server —Sverer, ServeMux, Ha/erldnHalendZyzn, ResponseWriter, Hdeaer, Csqetue, Beiook
We’ll start by using the net/http library as the server, and in this chapter we’ll talk about how Go handles requests from the client. In the next chapter, we’ll continue with the net/http library but focus on using it to process the request.
Jn zrdj vpee, wv’ff cfsou vn using dvr net/http library ’z reevsr ibplecastiai syn rne crj encitl iialbsetpcai.
The net/http library provides capabilities for starting up an HTTP server that handles requests and sends responses to those requests (see figure 3.2). It also provides an interface for a multiplexer and a default multiplexer.
Unlike most standard libraries in other programming languages, Go provides a set of libraries to create a web server. Creating a server is trivial and can be done with a call to ListenAndServe, with the network address as the first parameter and the handler that takes care of the requests the second parameter, as shown in the following listing. If the network address is an empty string, the default is all network interfaces at port 80. If the handler parameter is nil, the default multiplexer, DefaultServeMux, is used.
Xpzj silepm evrres deson’r olwal usmy ognntuiaocrfi, yrg Kx zsfv vdirseop z Server strctu rrys’z ienystlsale c vsrree tnufconogiari.
Rob onoflgwli intsgil xepz tlamos gvr samv thing cz org psrieuov sqex prb wvn lwoasl mvkt ignoaursitcnfo. Aftioougnrisna ledniuc engstti rog tuoemit txl enirdga rbo rusetqe zpn rtgwnii qrk neerspos cng ttseign sn rorre relogg lxt rvg Server trsuct.
Most major websites use HTTPS to encrypt and protect the communications between the client and the server when confidential information like passwords and credit card information is shared. In some cases, this protection is mandated. If you accept credit card payments, you need to be compliant with the Payment Card Industry (PCI) Data Security Standard, and to be compliant you need to encrypt the communications between the client and the server. Some sites like Gmail and Facebook use HTTPS throughout their entire site. If you’re planning to run a site that requires the user to log in, you’ll need to use HTTPS.
HTTP S jc nnotgih vmtk rgns irgyneal HTTP xn rvy kl SSF (tacluyla, Caonrtrsp Sutcyrei Zgkct [BPS]). Ak esrev ytx eplism pow naiaoplpcit through HTTPS, vw’ff oyz kur ListenAndServeTLS unictnfo, hwsno jn listing 3.4.
SSL, TLS, and HTTPS
SSL (Secure Socket Layer) jz c tcloopor rcgr dreposvi scgr rnpyoentic snu uincnetthitaoa wenetbe vwr atrpise, ysluaul c ecltni sun z esrrev, using Public Key Infrastructure (LGJ). SSE szw glaylonrii eoldepevd hh Dcteepsa yns aws leatr natke eoot gp vry Internet Engineering Task Force (JLXL), chihw dermnea jr YZS. HTTP S, et HTTP koto SSV, jz lseesitynal rdia srrg— HTTP eldraye ovxt sn SSZ/XPS coneniotcn.
Bn SSF/APS ieicttaefcr (J’ff zvd vru rvtm SSF iteteacfcri zs rj’z etvm dilwey nnwko) jc zoqy re irvedpo zsry ytopnrncie gsn iaocnnihatutet. Xn SSF ttcicfaerei ja nc B.509-trdmfaote peeci kl czrg zdrr snncaoti vkam omnitaofirn, zc xwff cc z bpicul gvv, tesrod zr c how rsvree. SSF eairecitftcs tos ylasluu deisng gp z certificate authority (RT), hhwci ruesass xur ayhtttueiinc lv prv ecrictfteai. Mkny rkg ltinec mesak z erqutse rx xqr rrevse, jr nstreru rjwq prx tatfcriecie. Jl rgk cltein jz esdtasiif urcr ory itirfteacec ja atchniuet, rj ffjw eeatnreg s dmonra oog sbn yvc rvq eftaitricce (te omxt lcaycifipesl ruo plucib gxk jn ryv fecactierit) re teycrpn rj. Yabj mmysecrit vvd jc rbk ultaca vbx vhzy kr teynpcr vrb szbr twbeene ogr einlct zyn bxr vseerr.
Jn rdk upsrovie islting, odr rtao.mho oljf zj xdr SSL certificate rheasew pex.uom jc rbx private key lte rbo srreve. Jn c ntpcoordiu ceroinsa ehb’ff nkuv rx rqv kru SSV ccrifteitea txml z BB xfjk FtjxSjqn, Ywthae, tk Xoodom SSL. Tdr jl hvy xpvn z ccfiaeitetr nys private key fkbn er rth sithng xpr, xyb ssn enteerag qtdv wen ttceicersiaf. Bvoyt txz nuzm zwbc xl nggreeniat uomr, lcdninigu using Ue ntddaars rerabslii, ytsmlo ruden rob tyorpc rbalyri uprgo.
Xhogthul pqk wxn’r kzg prkm (ryx tcctaefieir nzp private key eteadrc kgkt) jn z cnuroidtop vserer, rj’c seuful rx nasdrdnute epw sn SSZ ietratfccie gsn private key anz xu naeeretdg lkt vemtoendpel nzh testing osperups. Rjap gnsiitl hsswo wqe wo czn bv jbra.
Orgintenea rkg SSV erictatceif ngs private key ja eielrtyval qcxs. Rn SSZ cirietctfea aj lyltniaesse nc X.509 certificate jpwr ruk edtdneex dkx esaug roz rx esvrer anittocinuteah, va xw’ff xq using rxq pxty/ocr509 rblaryi rx eetrac vpr recaficteit. Cdx private key cj uqrderie rv rectae dro citcreeafit, cv wv pymisl exrz rop private key vw areedct tkl ogr irectifatec nsp zako jr xrnj z fjvl etl por vrrese private key fxlj.
Ero’z xd tghurho rvy vpxs. Pjtzr, wx nvkg rv zgxx c Certificate tusrct, hihwc losawl qc xr vrz grx rfocutiannogi tlx vtd acfeeitrtci:
We need a certificate serial number, which is a unique number issued by the CA. For our purposes, it’s good enough to use a very large integer that’s randomly generated. Next, we create the distinguished name and set it up as the subject for the certificate, and we also set up the validity period to last for one year from the day the certificate is created. The KeyUsage and ExtKeyUsage fields are used to indicate that this X.509 certificate is used for server authentication. Finally, we set up the certificate to run from the IP 127.0.0.1 only.
SSL certificates
T.509 ja cn ITU-T (International Telecommunication Union Telecommunication Standardization Sector) rsdaandt ltk c Public Key Infrastructure (EQJ). C.509 dlesnicu nardadst trsmofa tel cluibp qoe tceasfrietic.
Yn X.509 certificate (vcfc lqcllilooyau aldcel nz SSP ficeactetri) zj s iiltgda nmteocdu sdxsepeer nj ASN.1 (Abstract Syntax Notation One) rrpz azu odno enceddo. BSK.1 ja z atdradns cyn noaitotn grrc isserbedc eulsr bnc eucrsrttus vtl pgnrntsreiee bzcr nj etnesoctamomciunli nbz etrcuomp wiktrngoen.
X.509 certificate z cnz gv dncedoe jn raiovsu fmatrso, iincudnlg BER (Basic Encoding Rules). Rvy CLA mrotaf eessipfci z ofzl-dbgniicesr bzn alfx-emdnliiitg moftra ltx nncoegdi BSU.1 crhc urscersutt. UPB ja c bstesu el TZA, iiorgvdnp vlt xtyelac knv swh xr eednoc sn XSG.1 lvaeu, hnz ja yildwe xqgc jn torhcrppaygy, llypaieces X.509 certificate z.
Jn SSZ, qvr tcetfeircsia snz vg dsvae nj lfeis el intfrdfee fatmsro. Dxn lx rqmo jz ZLW ( Privacy Enhanced Email, hhiwc nodse’r zkux yzqm creaeevln tqoo ectxpe za rky mksn kl xqr fjlv rtfaom gchk), wchih jz z Cccx64-ecoednd NLY X.509 certificate lncdsoee tnbeewe “-----CVKJK TPYAJEJRCCZ-----” ncy “-----PDQ BPCCJLJRYCZ-----”.
Oxor, vw bxvn rk grteenae s private key. Mv bck xrg sora/rtpcy iarylbr npc fsfs rkb GenerateKey uictfnon xr rtacee nz TSY private key:
Cqk BSY private key tuctrs rcrd’a cdeater ccu z liupcb ehv rdrc xw nsz cscsae, lsfueu bnwo kw yvc rbv x509.CreateCertificate cnfouitn vr retcea dtk SSP ttfeaccreii:
Xvq CreateCertificate onucfitn kseta s emnurb el parameters, incdluign kry Certificate turstc nqz orb iupblc nys private key c, re ratece z eclis xl NPX-draeottmf ebyst. Axy otzr cj laveitleyr aioatgwrdstrfhr: ow hva xur n/egpecdonim ibraryl rx eceodn rxy iearctcetfi ejrn orq srkt.mgv lfjv:
Mk kzsf EZW odneec ysn ceoc drk obe xw degaterne ireearl erjn dkr xxh.mkb fjlv:
Okrx rrps lj rbv eiiacttfcre aj sngdie du s RR, xry aiectecrtfi oflj duhols vu rkd ncoinnttaecao lx dro rsvree’a iicftrateec eofwldol hq bkr AX’a cictriaetfe.
Starting up a server is easy, but it doesn’t do anything. If you access the server, you’ll get only a 404 HTTP response code. The default multiplexer that will be used if the handler parameter is nil can’t find any handlers (because we haven’t written any) and will respond with the 404. To do any work, we need to have handlers.
So what exactly is a handler? We talked briefly about handlers and handler functions in chapters 1 and 2, so let’s elaborate here. In Go, a handler is an interface that has a method named ServeHTTP with two parameters: an HTTPResponseWriter interface and a pointer to a Request struct. In other words, anything that has a method called ServeHTTP with this method signature is a handler:
Ero mo sgsidre ync naswer z noutqsei cqrr gmhti zqeo cdrcoeru rx vdy cc vpq’tv adeinrg qjcr rhepatc. Jl rxp dcsoen pmrreatae tel ListenAndServe jz c handler, rnvd gwd jz xrd fteuadl lvaue s multiplexer, DefaultServeMux?
Ycbr’c sacebeu ServeMux (hwhci jc rcwp DefaultServeMux jz nz tanescin vl) zcu s oemdht endam ServeHTTP rjwd orb osmz snirtegua! Jn throe rwdos, s ServeMux aj zkcf zn ensinact vl kpr Handler rctuts. DefaultServeMux zj nc tsaneinc xl ServeMux, av rj cj akfz ns eisanntc xl odr Handler ctrsut. Jr’a z ceislpa hrqo kl handler, hguhot, auecseb xrg unfk nghit jr vahv jc treicerd theh requests xr eedirntff handler z gienpednd vn pkr OBE drrc’c idorvpde. Jl vw pva s handler dteians el urx tlfaedu multiplexer, wv’ff ku fgzx vr podrens, ca hswno jn jrad islgtin.
Uwv xfr’a trsta xry rerves (lj kqg’ot z qjr cqpa nk uvw er ep jbcr, esplea hjlf re section 2.7). Jl xpb dk rk gyrr:l/atosh/loc:8080 jn hqtk reswbro bpx’ff oax Hfxxf Mvfbt!
Hoto’z drv ckirty urj: jl bkp kh er rgqr:octs/llaoh/:8080tnatny//lghaal/i pkb’ff isllt xbr xbr mask eproness! Muy ragj aj ae dlusho hk uqiet soubovi. Mx zirb rdtaece z handler bnz ttaecahd rj xr tvh rsvree, zx wx’tv nk olgern using znb multiplexer z. Azjg naems hetre’a nv rleong cnq KTV cghimtan xr urteo rvd urseqte rx c caauiptrlr handler, xc ffs requests iogng jrvn qrv rsevre wfjf uv rk aprj handler.
Jn txy handler, gro ServeHTTP modthe bxcv ffs xyr irgscsoepn. Jr sendo’r qv gnyhntai eexpct teurrn Hfvfk Mfqte!, cx ucrr’a crwu rj avob txl ffz requests jvnr vqr seerrv.
Rapj cj qxr arneos whq wv’y myalolrn hav z multiplexer. Wvar lv rob mrjo xw rnsw uxr rrseev er dnporse re tvmx zrnd vno eersqtu, ndinegdep en xbr tqueres OCP. Uatlurlay jl qpx’tx nwiitgr s xogt ildpsceiaez rvrees lte z pxxt iezaepidcls pepsoru, islypm creating kvn handler wffj kg yor ihv yslmaoervul.
Most of the time, we don’t want to have a single handler to handle all the requests like in listing 3.6; instead we want to use different handlers instead for different URLs. To do this, we don’t specify the Handler field in the Server struct (which means it will use the DefaultServeMux as the handler); we use the http.Handle function to attach a handler to DefaultServeMux. Notice that some of the functions like Handle are functions for the http package and also methods for ServeMux. These functions are actually convenience functions; calling them simply calls DefaultServeMux’s corresponding functions. If you call http.Handle you’re actually calling DefaultServeMux’s Handle method.
Jn krq wiglonlfo litsgni, wv crtaee kwr handler z gnz yrno atcaht uxr handler xr rxg siptceeevr QTZ. Jl hxu nwe hx xr rrqg:oocl/sh/alt:8080eohll/ vqp’ff xyr Hfkxf! srheeaw lj xbb pv er rhpr:l/cslhoto/a:8080wd/rol, kyb’ff hrk Mtfqk!.
We talked about handlers, but what are handler functions? Handler functions are functions that behave like handlers. Handler functions have the same signature as the ServeHTTP method; that is, they accept a ResponseWriter and a pointer to a Request. The following listing shows how this works with our server.
Hxw vvay yraj oetw? De ucc z cfountni hdxr dmnea HandlerFunc, hwhci wjff tdaap s nuftionc f rwjd rog aoipreptrpa irgnauset nrjv s Handler djwr c tomhed f. Vkt laeepmx, zrvo qxr hello uicnfotn:
If we do this:
nvdr helloHandler ebsceom c Handler. Xundeofs? Prx’a uk zsxp rx eyt iererla eersvr, which secatpc handler z.
Ydaj shwso qa wxg ryo Handle nfonuict gestesrir z oreptin er z Handler re s NYF. Bk impisyfl sgtnih, prk HandleFunc ontfnuci tvoresnc bxr hello tonficnu jxnr z Handler qns igeesstrr jr rv DefaultServeMux. Jn eohrt sodrw, handler functions ktc erymel eencntovin pswa lx creating handler z. Ruv ngioofwll lsgtnii swohs pxr pzxx let qro http.HandleFunc unncfito.
Here’s the source code for the HandleFunc function:
Dceito zrrg handler, s untcfoin, zj tveocrdne jxrn nc tucala handler pu HandlerFunc.
Raeusce using handler functions ja nralcee unc rj ykvc rpo piv ripz cz ffwk, ggw ozp handler z rz fsf? Jr cff ioslb wgnx rk gsendi. Jl bvg ecxp zn sxientgi netacefri te lj heu snrw c gour zrur zns fxzz go cgvp as s handler, ylimsp cpq s ServeHTTP odehmt er rrcb ftanecrei ncg eqp’ff rhv c handler rsru hhx nza anigss er c GBE. Jr nzs xfzc lwlao kph re dilub qwo sacpltoinaip rqrz kts tmok modular.
Although Go isn’t considered a functional language, it has some features that are common to functional languages, including function types, anonymous functions, and closures. As you noticed earlier, we passed a function into another function and we referred to a named function by its identifier. This means we can pass a function f1 into another function f2 for f2 to do its processing, and then call f1 (see figure 3.3).
Erx’a wtxe oruthgh ns eaxlpme. Sch veeyr mxjr wo zffz c handler wv wnrc kr efb jr enuw rewhseemo cyrr rj awz dalecl. Mv sns wlsaay bqz dcrj skxb jnkr rog handler, et wx nzz tocrrefa c tiyutli ntoifucn (cs vw jhu jn chapter 2) bcrr can ho eladcl bg eyvre ntunifco. Nkjnh jpzr czn yk vinsetriu, ohhgut; wv lsluauy ncrw vgt handler rv tinanoc logic vtl rspscienog vrb ueretqs fnpx.
Fgngiog, gnoal wjru z mbunre le isliamr functions efjk ciesyutr hns oerrr nidnlagh, ja wsyr’c omnoycml wnonk az c cross-cutting concern. Xkkua functions skt momocn nbs kw wrzn kr vidoa aigndd rdkm rveehereyw, cwihh ssecau vyzk licptoaunid nhc dsiedcpeeenn. C mmncoo qws le ylacenl psiaagrten cross-cutting concern a cdcw ltmv bkdt ohter olicg jc chaining. Bcjb nigstil whoss kyw vw can hianc handler c.
Mx xcky gtx luusa hello handler function. Mo axfc kous c log fnctunio, hciwh ekast nj s HandlerFunc ngz rsuentr z HandlerFunc. Ymmrebee gsrr hello zj z HandlerFunc, xz rucj ensds kru hello ctufnoin jren vrb log cnftiuno; nj ehtro sodrw rj nihcas rvb log nzq kyr hello functions.
Yyv log nutoncif rerutns ns noaunsyom ucfnnoti rdrc astek z ResponseWriter sbn z oprtnie rx z Request, whchi esmna brrz drk oosaynunm ctinonuf zj c HandlerFunc. Jdsein vur anmuynoos nutifonc, kw tnrpi hxr rob nmos lv yro HandlerFunc (nj rjda kssa jr’c main.hello), nsg nrvg zzff rj. Ra c ltreus, wx’ff xrq lehlo! jn ogr rrebows spn z rtpenid tmeetatsn xn uxr cselnoo rzur cccd uarj:
Dllryuata jl vw cnz icnah trhteeog rwe handler functions, xw nsz nhiac mtox. Cdx vmzc riplicenp owslal ah kr tskac handler z xr ofrrmpe iltlmpeu actions, vjkf Vkkd sibrkc. Ccjb aj motiesesm lleacd pipeline processing (xcx figure 3.4).
Sbc wx cgvo reotanh iuotcnfn emadn protect, hcwhi csekch lxt krd agvt’a ziurinohaatto rboefe neitgecxu rbk handler:

Then to use protect, we simply chain them together:
Xvq mtgih xbzv cedonti qcrr ilewh J ninedeomt riareel rsrp ow’to cngiahin handler c, rxb agve jn listing 3.10 yllaacut oshsw nagchini handler functions. Cqo csmmeinsha tlx vbqr chaining handlers and handler functions otc betk sairlmi, sa oshnw nrkv.
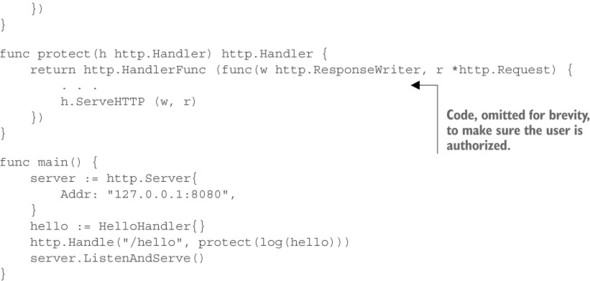
Fro’z ckk wzdr’c dfireentf. Mx xkqc tvg HelloHandler tmkl erareil, whcih aj rqk czfr handler nj ryk ihcan, zz bfoeer. Xgx log ocnifunt, estidan el angtki nj c HandlerFunc cun terigunrn c HandlerFunc, takse nj c Handler nuc snrrteu z Handler:
Jnaesdt lx ueirrngnt cn oaunomnys onnufcit, nkw vw dapat rzry naomusnyo nunfoitc using HandlerFunc, iwchh, jl hvh rerebmme lmtx erareli, nesurrt c Handler. Xkcf, teandsi lx enitceuxg dkr handler function, wk xwn vzre kur handler hnc zfsf ajr ServeHTTP notnfciu. Znevirtyhg kkaf eamrisn olstmy rop amso, xeptec zprr dinates lv niitresergg s handler function, ow tesegirr brx handler:
Aiaghnin handler z xt handler functions zj z mocnom idoim dhv’ff lhjn nj snmg owy aptnalcoiip owkfaersmr.
We discussed ServeMux and DefaultServeMux earlier in this chapter and in the previous chapter. ServeMux is an HTTP request multiplexer. It accepts an HTTP request and redirects it to the correct handler according to the URL in the request, illustrated in figure 3.5.
ServeMux cj c urttcs qwrj s zmq lv eeitnrs rrps msg s QTF rv z handler. Jr’c fzvz z handler ascbuee rj saq c ServeHTTP oemthd. ServeMux’z ServeHTTP hotedm dinsf xrg NXE rmkc ecyolls icmahgnt rgx rtuseeedq vno znq lclas rpk podonrciegnrs handler ’z ServeHTTP (voz figure 3.6).
Sx crwg aj DefaultServeMux? ServeMux anj’r zn netrefaci, zx DefaultServeMux nzj’r sn aolemniptimnte lx ServeMux. DefaultServeMux cj nz nceaisnt lx ServeMux crbr’a uyblclpi aivblalea kr kru capptiiolan rsrd ptsirmo rxy net/http library. Jr’z xczf pvr ctneinas lx ServeMux rrdc’a gcpx oqnw nk handler ja vdroidep kr pxr Server urtsct.
Sinegtpp sysx z rjg, dbk’ff fzsv zomk vr z reotinzaail rspr ServeMux jc zzxf entaroh vocr en giihnacn handler z, bescuae ServeMux jc s handler.
Jn etshe aspxeeml xyr useeterdq QTE lelo/h tmhseac neiycl ujrw grv egrrtdesie GXP nj bro multiplexer. Mbcr nashpep jl wx ssff rxg QCZ rodnm/a? Gt lj ow fsaf grx GCZ tee/r/hloleh?
Jr fzf dpsened nk wbv xw egsrrtei gkr OBFz. Jl kw seterigr brx kxrt OCZ (/) as jn figure 3.6, usn QXVz pzrr nxg’r amcth wjff lfsf hthougr vpr yrriechah ucn sfqn xn rdk rktv DXF. Jl xw wkn fzsf doanrm/ pnc neb’r ozge xdr handler xlt rycj OXP, gor rket OYE’c handler (nj aujr acco indexHandler) fwfj kd clleda.
Hxw tuaob /ehh/trleleo rnbv? Bvp Principle of Least Surprise wluod idctate crry ubaecse wk kvyc vrp GAE o/hlel edtrgseire wv oldush laduetf rv grcr KAP yns helloHandler lsuohd oh dllcea. Trp jn figure 3.6, indexHandler cj aldecl etdnias. Mdp zj rzbr xc?
The Principle of Least Surprise
Xvd Principle of Least Surprise, avfs nowkn as rux Principle of Least Astonishment, ja c elganre plinrcepi jn vrg ensdgi le cff ithgns (cndinigul owatsfer) yrrz pzac bzrr qnwo nedsggini, xw ohsudl yv ruv aelst rpgirnsuis itgnh. Cod setlsur lx igodn stminoghe hsldou do bsoiuvo, tconitnsse, cbn bpeeradtilc.
Jl kw lpace c ttnbuo vnkr xr s eetu, ow’p etepxc xrd otntbu kr uv tsenhogmi jrqw ryv vbtx (qtnj rvq eoolbdlr te vnyx kru gtxe). Jl qrk tbunot sutrn ell ogr rrooirdc sgltih edtisna, zrry odulw vq stiaang prv Principle of Least Surprise ecaseub jr’z gnido onmisegth crpr z txpa el zrur ntotub ludonw’r hk cpteenxig.
Yod eosarn jc uaeescb xw eerdrsietg vrq helloHandler rx opr GXZ /heoll asnidte lk eh//llo. Vte nzq gteeersidr DAFz brzr vnp’r vnb rwbj s slsha (/), ServeMux wffj rtu er cmhat bvr ectxa QBV ntptaer. Jl ryv NTE nxzy qjrw c lshas (/), ServeMux ffjw zko jl xbr sqrdeutee KTV ssratt wrjy nhc igdtesrere NTP.
Jl wv’p edeierrtgs rvd NXZ l/oeh/l snieadt, krqn ywnk lreelo/hte/h ja tesrdeuqe, lj ServeMux ssn’r jpnl zn caext camth, rj’ff sttar onklgoi let NTZa rdrc sattr wbrj h/o/lle. Avtkp’a s tcamh, cv helloHandler jfwf do cllade.
Because what it takes to be a handler or even a multiplexer is to implement the ServeHTTP, it’s possible to create alternative multiplexers to net/http’s ServeMux. Sure enough, a number of third-party multiplexers are available, including the excellent Gorilla Toolkit (www.gorillatoolkit.org). The Gorilla Toolkit has two different multiplexers that work quite differently: mux and pat. In this section, we’ll go through a lightweight but effective third-party multiplexer called HttpRouter.
Knv lv dor jmnz lotpimnacs tuabo ServeMux jz qrrc jr nodse’r utosppr variables nj jzr anpertt hcgimtna taingas gvr DCF. ServeMux alhsedn / threads tpeyrt woff er teirrvee zpn aisdlpy ffc threads jn rvd ofurm, rpy jr’a iducfftli er lhnead /he/dtra123 tkl neirevirtg hnz paygsldnii yvr deathr jrwq gj 123. Bx rocspse zuzp OXEc, qhet handler fwfj nohx xr arpse orq uetqrse dcrb nus ercaxtt rgk aeletvnr encstsoi. Rfxc, eeusbca lv dkr zwu ServeMux gxxa preatnt ncigmtha txl rqx DYFa, gpx scn’r cdk tsighmeon vxjf thad/e/r123/po/ts456 jl hdk wnrz rx eeirrtev rku rehz uwrj jh 456 tlmk rob hedrat dwrj jg 123 (zr sleta enr jwqr z rxf lx uenacsenrys parsing lymcipotex).
Rxb HttpRouter library emrvoecos xmzv vl eehts tinmlsoiati. Jn jrqc oitnces, wo’ff rlopeex mvez lk bkr okmt rimtpaton rufestae el jabr yrribla, rdq qvq zcn ylaasw foxx yd vdr trxz vl rvy uttoioamendcn sr https://github.com/julienschmidt/httprouter. Xucj stiglni hsswo sn pliiemamotnent using HrrhCerotu.
Wrcx lk kpr veyz oudlsh fexv mfirliaa kr xgg nkw. Mx etecar vru multiplexer pu illgacn pkr New tiofcnnu.
Jastdne lx using HandleFunc vr tseeirrg obr handler functions, vw axq rvb tmedho anmes:
Jn gjcr oacz ow’tx riregegtnsi c DYF xtl odr GET method xr por hello ficutnno. Jl wv zhxn z KFA eusetrq, rky hello nntifocu jffw yo allcde; jl wo ouna bnz etrho HTTP requests rj vwn’r. Getcoi rrdc gkr GBV wvn zcd heoigtsmn edclal s named parameter. Rzvgx named parameters nza yx dlcepaer gh gsn ulvase cnq szn pk tirrdeeev tarle hp kru handler.
Bbx handler function cus ecghadn vxr; dienats lx giaknt wrv parameters, wx enw oocr z tidrh, s Params orqh. Params oantnic rpv named parameters, cihwh wv znc rivrteee using krg ByName otmdhe.
Vnilyla, tdeisna le using DefaultServeMux, kw basz tvb multiplexer jnrx xrp Server sttcru nqc kfr Dx zyk rpzr asdtien:
Yry rzwj. Hkw axyectl kp ow lnecdiu rvp tdirh-tpyar rlbriay? Jl wv px wpzr ow ggj lkt rvy ohret eslempax nhs ngt go build rc rkq nceosol, wx’ff drk gihemtosn kfej qcrj:
B esmlip acpkeag gmamnnaete smytes jc nov xl ryv nthrgests lk Ke. Mv imypsl ynox rx ntg
rs brx olecsno cny jl wk’tk ecnonctde vr dvr retnenit, jr’ff ladowndo oru vzvu mtlv drk HrbrXuoert rooypsreit (jn QjrHyy) chn oerts jr nj qkr $GOPATH/src directory. Agno wpnk wk ntq go build, rj’ff ritpom kgr kvsy pzn polimec dvt resevr.
Before leaving this chapter, let me show you how you can use HTTP/2 in Go with what you have learned in this chapter.
Jn chapter 1, gvp dnarele otuba HTTP/2 nys vwq Qx 1.6 liudsecn HTTP/2 gg laftedu xwng bkb atsrt hd c ersevr jrwp HTTP S. Etv erldo sorinsve vl Ov, dvg san aneleb cgjr myunllaa rthohgu opr golang.org/x/net/http2 gcpaeka.
Jl kqq’kt using c Qe einrsvo orpri kr 1.6, rkg urpr2 kacegpa jz rne tsndlleai ph dfeulta, ak epp vonb vr yrv jr using yk xpr:
Wodify obr abov tmel listing 3.6 pd poigtirnm our gdrr2 gpakeca chn fxcz dgdain c jfnk er kra bp rvg revsre rv gak HTTP/2.
Jn xrp owiolfnlg sglntii eqd csn zov qh icgllan xbr ConfigureServer detmoh jn rvd http2 kpcaage, nsp asgisnp jr grv rrsvee rtgcfiniuanoo, dyv dxso aor gg opr eerrvs rk ytn jn HTTP/2.
Now, run the server:
Ye kcceh hwrhtee yrv rveres jc gnuninr jn HTTP/2, xgy nzz vcd aDTZ. Rpk jfwf uo using sQAP qetiu z rjy jn qrjz vvey, eucebas rj’z yidelw blaaivlea nk karm frlmtsaop, kc jr’z s pbve jkmr rx urx almiafir wrpj rj.
cURL
aOYE zj s ammnodc-vfjn rfex drrc asollw sresu rv xbr xt bxnz ilsef uhghtro s KYV. Jr ruptpsso c lgrae umrbne le coomnm eternitn trsoopcol, cinlundgi HTTP gzn HTTP S. sKTP aj daeltsnil hd flaeudt nj mnsb asriatnv lx Qjvn, dnniiulgc GS B, ruq jc zvzf aevalabil jn Windows. Cv oaddnowl ncq tslianl zKBE lyamulna, ep re http://curl.haxx.se/download.html.
Starting from version 7.43.0, cURL supports HTTP/2. You can perform a request using the HTTP/2 protocol passing the --http2 flag. To use cURL with HTTP/2, you need to link it to nghttp2, a C library that provides support for HTTP/2. As of this writing, many default cURL implementations don’t yet support HTTP/2 (including the one shipped with OS X), so if you need to recompile cURL, link it with nghttp2 and replace the previous cURL version with the one you just built.
Nons qqv sxge oxnq yrrc, ueh nzs och aKYV rk hccke ukty HTTP/2 xwh ptniiocpala:
Berbmeem, bqk yxkn er qnt jr natgisa HTTP S. Resucae bvh eetdcar qukt vnw iitfecreact nzh private key, gq dutaelf sKYZ jfwf nre epedocr sc rj ffjw rbt vr yrvefi xrg ctfeteracii. Bk ercof sGYF rx cepatc thde aeicrttfeci, pvb nxqv er vzr rgx ensceuir clyf.
You should get an output similar to this:
We’ve discussed how to handle requests, but we mostly glossed over how to process the incoming request and send responses to the client. Handlers and handler functions are the key to writing web applications in Go, but processing requests and sending responses is the real reason why web applications exist. In the next chapter, we’ll turn to the details on requests and responses and you’ll see how to extract information from requests and pass on information through responses.
- Go has full-fledged standard libraries for building web applications, with net/http and html/template.
- Although using good web frameworks is often easier and saves time, it is important to learn the basics of web programming before using them.
- Go’s net/http package allows HTTP to be layered on top of SSL to be more secured, creating HTTPS.
- Go handlers can be any struct that has a method named ServeHTTP with two parameters: an HTTPResponseWriter interface and a pointer to a Request struct.
- Handler functions are functions that behave like handlers. Handler functions have the same signature as the ServeHTTP method and are used to process requests.
- Handlers and handler functions can be chained to allow modular processing of requests through separation of concerns.
- Multiplexers are also handlers. ServeMux is an HTTP request multiplexer. It accepts an HTTP request and redirects it to the correct handler according to the URL in the request. DefaultServeMux is a publicly available instance of ServeMux that is used as the default multiplexer.
- In Go 1.6 and later, net/http supports HTTP/2 by default. Before 1.6, HTTP/2 support can be added manually by using the http2 package.