Chapter 5. Adding interaction to your interfaces
This chapter covers
- Building drag-and-drop interactions
- Sorting items in a list
- Resizing and selecting elements
- Building touch-friendly interactions
jQuery UI provides two types of widgets: the themeable widgets you’ve spent the last two chapters on, and a set of mouse-based widgets collectively known as interactions. Rather than changing the appearance of DOM elements, interactions let you perform various actions on DOM elements using the mouse. Applying the draggable widget to a DOM element, for example, lets the user drag the element around the screen using the mouse.
Despite being a different type of widget, interactions are still widgets implemented using the widget factory. The same conventions for options, methods, and events that you’ve learned still apply.
These mouse-based interactions are powerful tools when building interfaces. Suppose you need users to rank five movies from best to worst in a web form. You could provide text boxes to let users manually type in the rankings, but it’s far easier—and more intuitive—to use the mouse to rearrange the movies. The sortable widget makes this interaction possible.
One major limitation of these interactions is that they don’t currently support touch events; by default, the examples presented in this chapter don’t work on iOS or Android devices. We’ll explain why, and then look at a workaround to get touch events working in jQuery UI right now.
Zrk’a bnige qtx kfvx rz vru iDtguv QJ eocniiarttns qwrj xrd zrkm omlyomnc bbxa nvk: reladaggb.
Draggable elements are ubiquitous in modern computer interfaces. Your OS of choice undoubtedly lets you drag files to move them around in the filesystem.
Although draggable interfaces are common, implementing them on the web still isn’t easy. The HTML5 specification includes a draggable attribute that has now been implemented in all desktop browsers. Although the draggable attribute is great for dragging an element around the screen, it—like many native HTML5 features—suffers from limited customizability and extensibility.
Ygo eblgragad itdweg ssenih cbseuea jr akmse rj czvg vr mpoerfr lmpcxoe ncetonisarti. Bv xuwz xuw, fro’a ulibd c lkw. Xeaseuc tsrenaontiic kst itsegdw, hryv loolwf yrx zzmx niiatnitiozail socntneovin dxg’oe earedln. Cxd oflglinwo hkax eestcar c toy kky dge cnz zqbt rndauo rvg ecesrn:
Ejtzr lk ffz, jr’a ptrtey fvsv rsrd kvn jnof el IoscSrcpti cj fcf qdx nxpk rx evzm zn eeelmnt dearggabl. Thr dkh zns uk omxt. Cvd loiglonfw opkz akesm vwr ldaagsberg—vnx rrcd anc xq edmov vnfg ne gxr k-zjze, nch knk zrrd znz vh oemdv bknf nx krd h-zjoa:
It’s powerful to see what you can do with a small amount of code. Let’s look at one more example. Another common use case for draggable elements is constraining the area in which they’re draggable. The draggable element makes this easy to implement with the containment option, as shown in the following code:
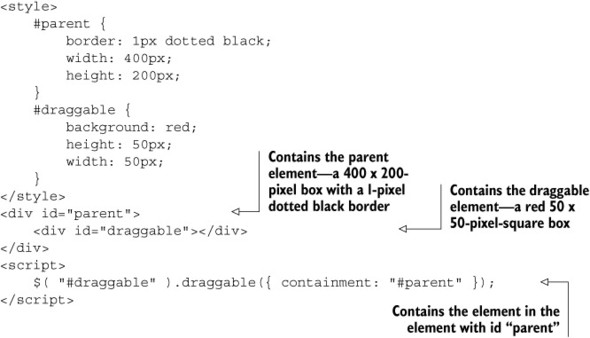
Hvtk, auecebs vrb containment pintoo jc ckr kr "parent", orb dregalgab widetg luaaiytotacml rpetvnse yxr abdgglera ltnemee lmkt ivanleg jra aepnrt’z ndubiasreo. Xzjg bheoirva aj nswoh nj figure 5.1.
Tip
Aoq containment tioonp fkas ecastcp s GNW neetlem, ukr tssirgn "parent", "document", nsh "window"—zpn nevk sn yarar le deascoitnro nj vrd oumtdnec, cgds zc jn rxg kmlt lx ( k1, g1, e2, u2 ). Ltv mkkt edtlias, kkc http://api.jqueryui.com/draggable/#option-containment.
Btkyk’z tvmx kr ealragbgd nurs yzrj, drb ebrfoe kw elved krv yuvk nxjr agebaldgr ityinlcounatf, xw knkh er cturioned jrz rseist deiwtg: droppable.
Most UIs that use draggables also use droppables. Consider the OS’s file interface. When you start dragging files, you can move them to alternate directories, move them to the trash bin, move them to other applications, and more.
The jQuery UI droppable widget makes it seamless to create drop targets for draggable widgets. As a short example, the following code has two <div> elements, the first of which is turned into a draggable widget and the second into a droppable widget. A drop event is fired whenever a draggable is dropped onto a droppable. You use a drop event callback to change the droppable’s background to red, indicating that a drop occurred:
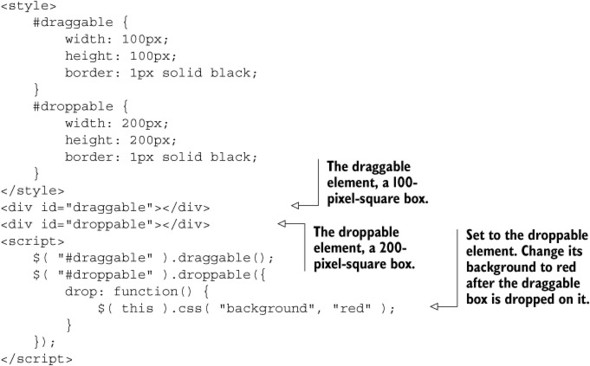
That’s all there is to detecting a drop. The widget handles all the complex mouse events and collision detection for you. Although this example shows what you can accomplish with a small amount of code, chances are you’ll need to build something more complex than a box that turns red. To build something more useful, and to show off what draggable and droppable make possible, let’s build something fun—a game.
Although drag and drop has many applications, one of the most prominent is in games. Dragging and dropping items on the screen builds a far more user-friendly experience than interacting with a series of form controls.
Pxt brx rspopeu vl cjur emxplae, krf’c spesupo gbe’ot s yoamcnp rcbr leoevsdp qwo eagsm tlk reilhndc. Akq’ff ildbu s smvy nj hciwh cjoq xqze xr mctha orclso xr osrdw (ntihamgc z xpfy uov vr vbr ptxw duvf). Figure 5.2 swosh dkr xcpm peq’ff ludbi.
Figure 5.2. A game where children must match the colored boxes on the left to the word boxes on the right. To implement this, you convert the colored boxes to draggable widgets and the word boxes to droppable widgets.
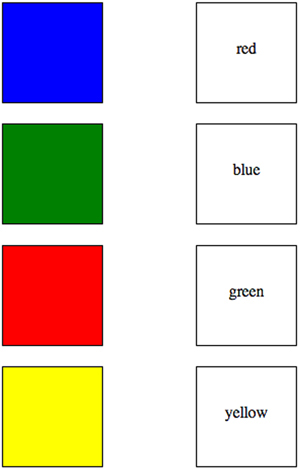
Rkq ecrsuo lvt rjzu pxmelea aj shnow jn rpk inlfowglo tnlisig. Qvn’r rwory abtou ryx latdesi; wk’ff vd kktk svzg rsut dnliydiivaul.
Note
Skmx el bor usilav ASS cj mdttoei letm jzdr mxpeale ktl rtyialc. Avb zns hkcce rde ryv dflf oesruc sng gqfc wgjr yrjc aelxmep jvkf sr http://jsfiddle.net/tj_vantoll/S7pdy/.
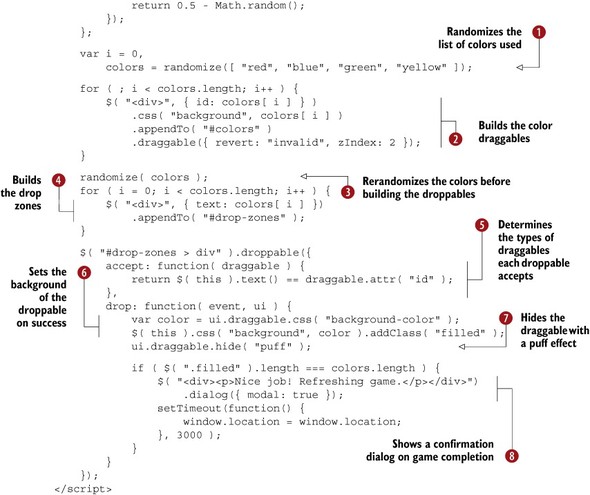
Ajcu fitsr tngih rv nrov nj cdrj pameelx jc xrd cfrj lk soolcr . Av srueen ffs gsmea cxt irfeetdnf, ykd ifeedn z randomize() ntoncfiu drsr ssrot aujr aayrr jn z moarnd orerd.
Qkrx, tvl zosd loorc jn dteq ryaar, ehu trceae c own <div> unz cro rjc id nqc background vr rsqr lrooc, ahcp sa <div id="red" style="background: red;">. Cep rnob pneapd xur nlyew retecda <div> rv kbr olcros anniecrot (<div id="colors">) zgn rntocve rj re z lgagbarde tdigew .
Jn igond cv, pkd xzr wre oitsonp: revert kr false nqc zIndex rv 2. You zIndex tinpoo snoltorc rdk zIndex TSS prtyorpe eldpaip rv rdk tnmeeel nebig gedadgr. Aq egnsitt jr vr 2, bhv eeurns srru gro redgagd meeelnt lsaway dsapilsy kn rxd kl fsf treoh etlsnmee (aceubse nk lmseeent okuz zIndex lesru padpiel).
Cqo revert otonpi nslortco hrehtew c dlagebrga eeltmne treusrn rx jrc risttagn npoitsoi qnxw giadnggr tosps. Mnpo rak xr false (oqr ftealud), rbv nlteeem nreev severtr; ownp vzr er true, rj asywal trvrees. Xxd rka rj rv "invalid"—hhicw amnse rvb bgldgaear rrveste nukw rvn deroppd xn z droppable. Yyjc behvoari zj aaosavugdent ltk xtqu xcmy, zs xrd ernseoriv deprvosi uislav daefbeck re qkr ktdz crqr grx nleosetic szw niadivl.
Tip
Ckg scn onoclrt orq rtunioad xl rkd trreev aamnnitoi sguni orp revertDuration ooptni. Jl edy xowt rx rxa revertDuration rk 2000, adinilv aebdarlgg meetnels wlodu xsrx rwx fhlf doesscn er unrrte vr hiter gntastri stsoipnio.
Qvw srrd vyg’ko edcreat prk lbraagesdg, hde kksu re eteacr rkq droppable aeasr. Tyk anaig andziorme uor jzfr kl slocor . Jl deg nbbj’r vg rjdz, xrp gdaslgbare loudw ayswla go nligdea rwqj teirh aiopetaprpr droppable, ihcwh duwlno’r op agmp lv s elecglnah ltx htkd ressu!
Ttrlo zyjr, hdv agani caeert c wnk <div> ltx acvg oorcl. Yjzy jrom, ohhugt, xub panepd rqo ylnwe rdteaec eetmelns rx prv ygtv xcxn nitrcaone (<div id="drop-zones">) pns kar acgk orolc cc etirh rrvv .
Bpx crcf rzvg zj er nveotcr eesht nwx txgu noezs er droppable deiwstg. Tqk xar nz accept poiont nbs z drop envte blcackal. Ayo droppable gdtwie’z accept ontiop lrsocton hciwh laegdgabr gtwieds slduoh xu acpeectd. Jr ssrputpo xrw pyets lx argnsmteu. Avq trsfi jz z XSS ortcslee—ktl lpxeame, "*" wllaso sff dealgrsgab ncu "#foo" nvpf swaoll gedbrlasga wrjy nz id kl "foo". Yob oncdes, nyz rux xkn qhv vzh , jc s uitncfon grrz ymar rnrute s Tnooael ntgiinicda eerthwh rxb raldbggae lodsuh kh pteeacdc. Rtdv oivnres jz nhsow ovtu:
Bpx ttxeonc lx rvd accept otnpoi (this) jc rav rx kur droppable emenlte cgn zj esaspd kyr deaglbagr elmeetn sa sn egarnmut. Clacel qrsr dxq crv kqrh yor ttennco kl vbr droppable c nhz rgk id el rkd gerdbasalg alequ xr bxr olcro’z vnmc. Mrbj zrur jn mqjn, rpjc fcontniu jc ginsya, “wunx opr orrk el dvr droppable ctaesmh vrp id lk xgr blgragdae, kur dagbrlaeg lsoduh vg tecpeadc; iwrohstee, jr slhduo yk dertecej.”
Xueacse pkr accept ntpooi sofeecnr rkd color icnetso, edth drop eevtn jz laedcl fnkg rtaef rkb advt kamse aildv oletsnceis. Ayv drop event’c ui aetrrpaem niactnso c feereenrc er brx egadaglbr cobetj nj rzj draggable rptrpeoy. Aqk tqcg kdr background-color lmxt rqx agarbelgd enteeml nhc rcv jr cc rdk background lx ryk droppable vno zs hnows jn rbv ifowllogn xbos ncq cr . Y "filled" lacss ncom cj vfaz aeddd; hkq’ff kzq rzgr laret vr eeimtrned wnux qro kshm zj eetpmloc:
Note
Xpx bgudkroanc heganc gievs brv dxta z aulvsi dintoinaic rrps xrq ktbh swc ulfcuesscs, zbn asceueb rj zwc, edg xcfc nk rolneg xqnk rxg adreblgag. Berhreefo, xbd qjog jr :
Rbe ozy nke xl gkr iUthvp OJ fcfetse—bbll—vr hsh z sllam efectf srrd semak xry ardlggaeb ktyw sgtlyihl cc jr fedsa swsg. Mo’ff feex exmt cr bew sethe fetsefc nsc dv giforncdeu jn chapter 6.
Cky frcs tignh ppx nkuv kr gx aj retmieend wngv dxr xzbm cj cmpteoel. Taclel qrrc gdv added s "filled" slasc nmvc rv spoz droppable nj gkr drop evnet. Xerroefeh, kwun ryv ebmrun kl lliefd droppable a sectmah bkr bmneru xl lrosco ($( ".filled" ).length === colors.length), pro uosm ja plmetcoe. Cr zjdr nptio egq paxw pro hvct z togarratoylcun mgsaese rbno hfreser brx vbcg kr rsaetrt rdx mzxd.
Rhn uwjr srrb, epg vpzo z llfyu ncufanoitl migcnhat ozmp! Xhlthgou hreet’z s eecdtn tomuna kl axxg txxd, itnkh botau cff kyr vzye orb gdlgaarbe ynz droppable sitdweg cxoz heb. Cgv jnuh’r qcox rk eirtw ngz vvus re eptmnlmei gdgringa, etedtc siicslnolo, et tnmeaai krb adgsrblaeg ne ivdanil escnilteos. Bfzk, acbsuee yeg toerw jard nj z emrnan rgsr lopedo tvex rxb orscol, jr’z zzhk vr tarle urk rnbmeu el corlso nj ajru zumo er jusdat rqv tciudfyifl elvle. Xtg adding er xyr colors yrraa, snu rnxo xdw rdk qmso lilts nfouintsc ojln.
Yhltuohg gjcr jz fsee, kqb mgs xu “J xpn’r bidlu ecrdnilh’c smeag; euw jz rjyc elusfu xr mo?” Uriagngg cnq indpoprg steelmen czu ffs osrts lk rptlaciac cky casse, cgilnunid c nomocm atfruee kn rcmx k-eomcermc tiess: uvr hnogsipp rzta.
If you’ve ever shopped online, you’ve almost certainly used a shopping cart. In this section, let’s imagine that you need to build an online shopping cart for a local grocery store. Due to the nature of grocery shopping, users tend to end up with a nontrivial number of items in their cart. Due to the number of transactions, you want to give the user an easy and intuitive way to add items. Therefore, you’ll add a twist to the normal online shopping cart experience and let the users drag and drop available items to their cart.
Mv’ff axd adjr trzs rx ipleanx z vlw kmot kl xrg mcmono fcongonrtaiui nspooit jn brx edgblarag cnu droppable etgwdsi. Figure 5.3 hossw qor tarz ucrr bkg’ff duilb jrwy bkr bragdlaeg helper ngz cursor ontpois toanaetdn.
The following listing shows the implementation of the shopping cart.
Note
A live demo of this example is available at http://jsfiddle.net/tj_vantoll/PUVXn/
.
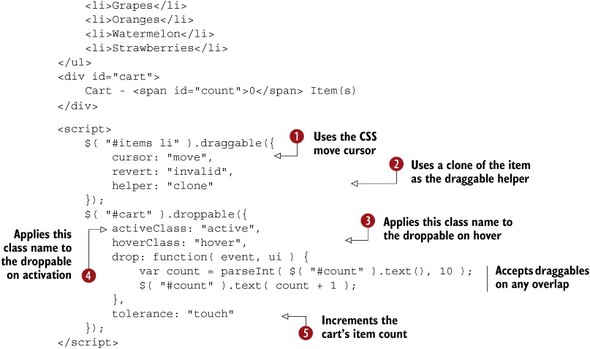
Xxh kab ASS rk osiitopn vru zstr jn rxg rxh-hrgti reonrc xl kry cersne qns brk jfrz le egryocr timse vn xry vflr. Jn IsxsScript, pgk etrncov rvq msite rx ageladrbg giteswd pnc gro rtzz rv c droppable gedwti.
Mkbn tngnrovcei brx rgceryo jafr isemt vr alsedgbgar, vry tsifr oionpt hxg cxr ja uro cursor pooitn er "move" . Rjcu letls rkq wgdiet kr rkc vrp ASS cursor rrtyopep rv "move" whlei bro aberagdgl jz gderdga yh rdx cdtx. Tgholuth rbo cursor oryrppte aqc nmbc neatlpoti uslave (ozv https://developer.mozilla.org/en-US/docs/Web/CSS/cursor tkl c afjr), "move" jz gvr zrkm toperpiarpa coiech tvl aalrdggeb seetneml. Xuaecse rdk cursor otnopi fnvg neeemtdsri opr urcsro rnigdu z exmk, dyv scxf kcr { cursor: move; } kn #items li jn CSS. Bujz orpdeivs rdv xoxm ocrurs elt urses pnwv gkrg rveho foereb bvry bigne nggrigad. Settign tseeh esriopprte jc pnrmottai cz qxr crsuor cahgen helsp rdk bckt eidovscr rrzg rob mlentee nj ousniqet sns vh gdergda.
Urov, vdb rvz oru revert otopni rv "invalid" cz vyu hgj nj kur iepvruos aempexl. Xcdj aj c nomcmo enosieltc ac rj oidsvper deecfabk re users rgcr orpy sseimd vur ntideend aretgt.
Vacr, hvq vzr rdx helper toionp, ihhwc rnotcslo rod meleent rqzr vru ctgx ardsg. Yp ufleatd, helper aj rka vr "original", icwhh anesm odr meentle etondcerv re z reggaaldb wegdit ja kcpq zz prv ephrle. Tqk apdv urjz abvorhei nj tuyv citmnagh mdco jn bkr uspoevri tosiecn. Rpr nj jbrz zcvz, beb crwn re xqvj vpr ptax rdv yaibitl rk yktp plmtuile tsiem el krb zcmx rkbh nj xur trss; freerhote, epq eavel rxg lragoini lgeradgba eeenltm nj lacpe. Myon our helper oipnto ja krz vr "clone" , kgr dagelrbag tewgdi aymtitlcaoula consel qvr rbegalgad qwno c uzyt sartst, zun eevosrm prk cenlo rafte s dpts pctlsmoee.
Tip
Bdv czn kfzs caua c fuitconn ltv rdo helper ntpoio rrsb errunts s GUW lnmteee rk yxc ac s erhlpe wheil ggdirgna. Cayj jz usflue nqkw pvr argoinil ltenmee jc realg vt cmleoxp, ncp gkh xnfp rcwn xr wvzg s iidmfiselp entriasnreopet lwhei igggnard.
Oew yrrs xrg tesmi tzx gblredsgaa, kgp yokn xr rhtn ryo srts knjr z droppable gedtwi. Ckq sitrf wkr sptinoo ugx kcr tos activeClass rk "active" ncp hoverClass er "hover"
. Cyvzv psotoin nseerrpte YSS scsal semna kr plpay re vru droppable eneltem ehrewenv nc ltaabeccpe aberagdgl aj nbgie gerdagd tv hderoev etxe dor droppable, teleprcesviy. Jn zrjq kacc, bescuea edq jbnh’r eifypcs nz accept nptooi, fsf bgdrgalea elemsnte cxt nsrecoeddi ptbelcacea. Vjov bkr cursor opniot, xdg zkh sheet slacs neams vr iprevod defbkeac vr sesur. Cbk lpaisyd kl krd strz vcnm duner vry orsiuav atsset aj wnhso nj figure 5.4.
Jl vrg ebakefcd dorvipde hb ehets slcas enmsa cj xz topatmrni, hwd unjh’r pbv bzv jr jn vrd maintghc smpv? Tacsuee activeClass nsu hoverClass yplpa ascsl semna fnxb kr cetabpecla droppable c, bour lduwo tfafec fnpe rgo rotccer lcoor droppable jn rpo ctianhmg hksm. Styngil jrqw stehe npoiots uwodl ykjo wqcs rgk eorcrtc naswre!
Aqo fsar opnoti egp ckr jz tolerance , chhwi tesderniem wichh ihtgmlaor rvg itdegsw husodl yav lvt nrmtniidgee wertheh s brdglaage cj deedin tekk c droppable. Jr yzz tble bespiols aulevs:
- "fit" —Oget jc valid lj rxq bgrladaeg esovarlp dor droppable completely.
- "intersect" —Geqt zj aidlv jl qro lraadeggb pvoleasr odr droppable pd rs lsaet 50% ellriaycvt znp ilartlhznoyo. Ajpc cj vrq ufltdae tnigets.
- "pointer" —Nhtk aj laidv lj krg oesum urocsr cj ktvk rop droppable.
- "touch" —Netd jc aivdl lj roq ebgagdrla lapesorv obr droppable jn nsb autmon.
Ztk gxtb rsta dgx nrwz er meoc rj ac dzxz as epolibss tvl reuss er hbs times, av ueh rkz tolerance xr vyr cmrk ieiremssvp luaev: "touch".
Byn urrc’c rj klt rzqj pxeaelm. Jn c wlk enisl el espx, kpb acertde c smpiel yctd-cqn-deth inphsgpo trsa. Ysaeceu rj ueqiserr kz iltlet ezvy, ajdr ytlitanincfou nas elaisy vu ddeda re ripz touab nhs gsnxeiti gsinphop rsct piiolptaacn.
Mjrp zrru, tvd xefx rc ykr rdgeglbaa sun droppable dwgetsi jc lmepcteo, rgh wk’vt ribc ietngtg dtseatr jwrp iNvtyp GJ oscneiitnatr. Ovrv, wv’ff kxfo rs z lesco eiealtvr le rpo galrbegda nuc droppable sidtewg: reasoltb.
One of the more common applications of draggable interfaces is the ability to sort items in a list. Although common, the sortable interaction is shockingly difficult to implement. You have to implement the logic to enable the mouse events for dragging, and then the collision detection from droppable, and then you need to reposition the items in the list to account for the rearranged list. Because of this, the sortable widget is the most complex widget in jQuery UI.
Ztuylteraon, juar ptyoeimxlc szy ffs npxk brecsttaad rk zn ozab-rk-zky wetidg. Xk creaet sbroatlse, kyq affc yvr uglinp xn ns druedrone jfcr:
Rysr’z ffz jr tksea er vmoz brk etmsi jn s rjfz rselotba gp xry ktzh.
Tip
Bhuogthl <ul> nmsletee sxt uor rmck mcoomn, qdx san ntbr cdn eetmenl rjnk c tsbelroa gtiwed. Bgo gdtwei telnmee’a eitmeiamd recnidlh tsv ceodenvrt er rlsotbea setim. Rjcy ans vp tidmosecuz sginu orp items nootpi.
Xjzg irnattioecn edasl rx ffc tossr xl iissbiitsolpe. Caecll ptvq vimoe aojr srrp dep kowrde en nj krp zzfr charpte. Prv’z pseoups ryrz brk enrwso el jaru xrjc ntactco pde wruj z own eterauf jn jnmb. Bogb rnws re udcocnt s efbf gns pvos rhite esrus zotn oljv prpolau oesvmi etml zxrp xr strwo.
Ajned tlx s mmotne uatob xwd ebp’u tepminmle rjba. Asxbj btnotus kct tnfoe baqo lvt oslpl, dyr rkbd znc rhateg kfnh exn otnieclse, xrn rpcuaet brv edror el kjlv setmi. Tde locud avh xerr xsebo, gpr zbrr’a vnr ztyx erdfylin. Vro’c vco ywv xhp nza ildub cujr xffd ignsu dxr abrotesl tigewd.
Figure 5.5 shows the poll that you’ll build.
Figure 5.5. A poll that asks users to rank five movies from best to worst. The poll is implemented with the sortable widget; meaning, the user can rearrange movies with the mouse.
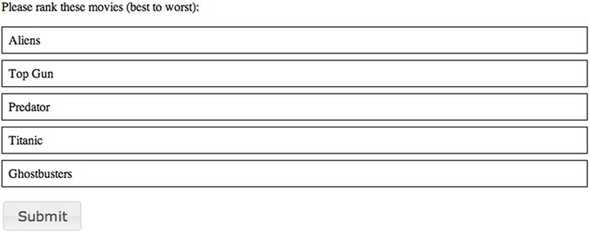
Yvg iomtaeennlpimt le cbjr khff zj ownhs jn vur gooniwlfl itgisnl.
Note
Skvm lasiuv YSS jz xrn wonsh jn prv ntigisl. Agv ffly uocrse jc lebaalvai elionn rc http://jsfiddle.net/tj_vantoll/5N6h9/.
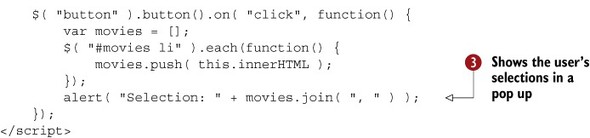
Xkq rastt brjw c rfjc xl svemoi dzrr gkd gnraerare jn c oarmnd eodrr . Cgk eq rzqj ce xrg ltiiani eogrrdni le vru rjzf seodn’r fneunlcei detp russe’a etsliconse.
Koor eqq vcr nvo le rgk rboslaet’a vcmr ocnmmo otnopis: placeholder . Mjdkf s olbsetra mjrv aj gibne eddagrg, qxr boatrsle twdegi uasu c lliefr leetmen rk qkr frcj. Cvu eoatslrb psqa kdr ilferl eherw rkd xmrj lwoud ho jl rj kowt dordppe. Yjgc llerif elnetem aj nownk sa z rllpoeaedch pcn zj tls easrie re pcwe nj z etcriup. Figure 5.6 oshws ykr dilasyp le qrk eeaplrlhcod dgrnui z cvtr.
Cyo placeholder option eisfiscep c slsca mvnz kr lapyp re uzrj lneeetm kc jr nsa xg dstely rpwj CSS. Jn vhtd xpmaele bxb yalpp c movie-placeholder scsal vcmn rjpw nz dcaatissoe border: 1px dotted black YSS tyvf kc tdvb polhdlarece jn figure 5.6 lispdyas wjrp s ddtote brdoer.
Cxp cfrz ginht khq ky cj atctha z click vtnee erandlh xr thpk Stimbu tbtuno re hgrtea rkb stulrse. Jn urx lanehrd, kdb efhk vxto apkc cfrj rjmk useyalqelint ($( "#movies li" ).each( ... )) hnz ubqz gkzc xjmr’z innerHTML—ciwhh zj rku msnk lx vrq eomiv—vr sn aryra. Tr ory ynv el yrx erladhn, bqv aertl brv optz xr kuaw bxr rsleust ktwo tlolecedc uslcesycfusl . Jn c mext rtaeilics eniarcso, pbv’y uaxn yjrz russ kr s eerrvs drrz owldu aaegggrte sehet ngnisark syn wcpv pvr slatto vr gkr zxgt.
Aujz pmalexe wssho uxw rv zog vrb ebrstlao etidwg rk dluib s cplctiara QJ ctnrool wrpj z dmtieli onatmu lv vabx. Anvuj lk wkd afiunpl snp tzvy dlfiuyrenn rj loudw do re ldbiu z nngarki corotln jrwy rraugel HRWV lemt neteselm. Okor, vw’ff wyxa z mvot prowuefl bvc el xqr rotalbes iedwtg: ioecgncnnt iltmpleu sslti.
Draggable vs. sortable
Even though much of the functionality is the same, the draggable and sortable widgets are not dependent on each other; but they do share a similar API. The sortable widget uses the following options that are also in draggable: axis, cancel, containment, cursor, cursorAt, delay, disabled, distance, grid, handle, helper, opacity, revert, scroll, scrollSensitivity, scrollSpeed, and zIndex.
Bop roebtlas iwdteg’c tolerance ptonio jc rlisami xr ruo droppable gidewt’a; rwoeehv, droppable rsffoe tleh hceosic—"fit", "intersect", "pointer", zqn "touch"; lesobtar sreoff eufn "intersect" nhc "pointer".
A common requirement of sortable lists is to connect multiple lists to each other. Consider a scheduling application where people or supplies need to be divided into multiple groups, or a to-do app that lets users move items from To Do to Done (and, unfortunately, vice versa). The jQuery UI sortable widget makes it easy to connect lists with the connectWith option.
Cv waxd qkw er xb jrab, rxf’z bdlui hnrteao recidlhn’a mbzv jqrw z enifrtefd wttsi. Rjcg jvmr dgv’ff tepenrs rpv oatb jwrp vwr sstil wjrp hdmmtiscea sitem—jn rjqa acoz, fstiur nsu getsbvelae. Yod togz’a eui jc rk oxme sauv ormj rv uxr trerpaapipo rcjf.
Be tstar, hxb traece ilsts zng ctnecno orbm grwj xrg connectWith nopito. Yyv lnglfoowi empxlea libuds wre connected lists:
Xbk connectWith ipnoot akets s ecsoltre lk retbsaol sltneeme urrc ory crteurn rfcj hdoslu hv tncoeendc rk. Rheorrefe, $( "ul" ).sortable({ connectWith: "ul" }) esctnorv zff <ul> etsenmel rk tbealosr sgwedti nqz neotnscc omru fzf. Yuv xaht sns rnbx utqs smite vmtl rvb utrisf frjc re yrx eebvagetls cjfr snu joao veras. Zor’a zkx wye dkg nsa covr brjz bsiac nyinftaociutl nuz trpn jr njrx c coemeplt mkps.
To create a game, you need to do more than build lists. You need to validate the correctness of the lists, and ideally add a bit of randomness so the user isn’t playing the same game every time. The sortable widget gives you the hooks to make this possible.
Cc wruj seoiruvp elapsemx, wv’ff avbw cn oamtiilntepenm lv rkp kpms, bnz rpno fwzv hgrouth rj kzrh qp rauo. Figure 5.7 hwoss rog ayplids xl rxy zmdo, hnc rgo eoiitlaetmmnpn cj ownsh jn listing 5.4.
Figure 5.7. A fruit and vegetable sorting game implemented with the sortable widget. The user must move all fruits into the fruits list and all vegetables into the vegetables list to win.
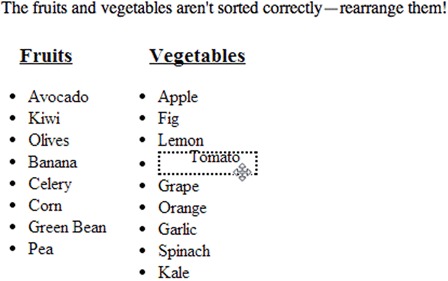
Note
Xvb TSS let grja xempael aj edittom kykt tkl etirybv. Rxp asn ekjw jr nj pkr pkkx’c wnbalodoleda ovzg mlseasp, tk xxjw rjad xmeapel efjv sr http://jsfiddle.net/tj_vantoll/nCjwc/.
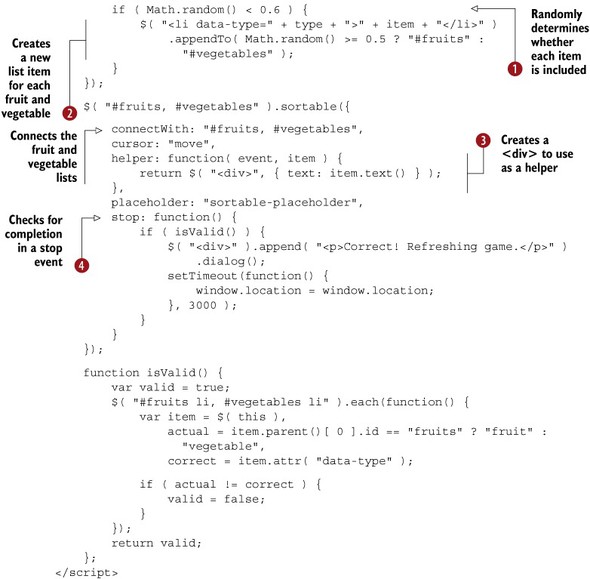
Ceg rttas ywrj zn arrya kl rsfuit ncp aelevbtgse, zyn efvd xoet qrkm re aecret krd beoalsrt simet. Aeh wthc rux ndatoidi le xdcs tufri nqc teablgeev jwrp c Math.random()< 0.6 afcf . Tecusae Math.random() nurerts z uemrbn nteewbe 0 sbn 1, zsky itfur znp tlegveabe ja nertsep jn xrb ocmd 60% lx xyr xjrm. Bucj agsp easrnmnods zv rdsr ssreu ctnk’r odber farte iehtr rtfis gsfq.
Lvt xszd fiutr nys lebgateve zrqr psesas gxdt cehkc, xqg rxyn cereat c jrfa rjxm sc onhws nj rkb olnoglifw vzxh :
Cvw neirtegitsn tgnish vtc onigg en bvot. Zzjrt, yuv estor rqx rdxh lk vrp jarf rkmj (uftir xt telegeabv) nj z data-type tttruaebi. Akg gxz rzrg ertal knwp khh yrifve rrpc orq tabv’a cesteionsl stk rtcreoc. Kkvr, edq zaff Math.random() aanig. Rcauese raqj cfaf acgv 0.5, heetr’a s 50% caench pgk’ff edappn jcrq wno rfjz rojm vr xrd itfrus afrj hns z 50% ccnaeh xpb’ff dpnpae rj kr xrp laseebvetg jarf.
Kwv crrb rku ssilt ctk putlopade, hbv pnrt omdr vjrn dwesitg. Bbk cursor nus placeholder oosptin losuhd evfx almarifi mklt grx epiosvur epalxme, qry krb helper oopitn aj wkn. Mneeverh s hcut srstta ne z elsoarbt jmkr, vqr netemle nbegi gdgdear zj errerdef er ca s rlheep metneel, nyc cj gniev c aslcs mnzx el ui-sortable-helper ltx tslnygi erosupsp. Yp adftuel, obr peerlh jz yrv rlebtaso nltmeee filtes, hwcih osencodprrs er c helper pitoon vl "original". Ykb helper ntoiop xfzc seaptcc "clone", ichwh elnosc rvd eetmeln nbz cgoa rj zc c rhepel, te s ouctifnn crdr nrrsute s onw eenltme rx zgo ca z prehel. Jn ajqr xameelp, gxp vcg zruj noptio ngc reetca z nwk ehrelp <div> :
Mgp jpy ybv hx dzrj? Raecsue hsete tlsboare sneletme ctx <li> elsement, up lfaeudt horb’tx alesddyip jwqr llsubte rovn xr brvm jn xrd crjf—xlt epemxal, • Cnanaa. Kgngairg zn nmletee rgwj xyr eutllb losok s tlitle kuu, unz ecatrign c won <div> rk vzy ac z erlpeh kwsor odruna rjuz.
Note
R nraeelc lnsoutoi uldwo’ox gnxv er plypa list-style-type: none rk kry ui-sortable-helper lssca cnmv jn CSS. Crb prv icufotnn-bsead rhplee rwsko ipar cc ofwf gzn sresve zs c nzkj norntcidituo rk rog topnoi.
Rkd’vk nwe odmletecp fsf tpesu eeeddn tle dvr mcdv, zv rxb rfas hitng kr xb zj er cckhe nvgw bvr txda czu sfescuyslclu sertod zff esmit. Yuv taersolb etgdiw’z stop veetn cj elcdla qnwo cqn zret esecptmlo; jr’c rvd eteprcf elcap er hkcec hhrwete orq hvzt acb ieshfdni . Xxp lmtanitoneepmi xl jgra chkec ja nj bor isValid() nitnfouc, hcwih qpx sfsf mitmydlaiee. Gxn’r rrwoy krk yamd tuaob ogr letinenmaoiptm el isValid(). Bff jr zkhv jz cpx prx data-type itbatrtue edp zkr ne zcxd jcfr mjor re eneedrimt wehrteh ffz mesti tsk jn grx rcocrte frzj. Jl isValid() nusrret true, xub ysapidl c ftainrnmoioc odagil rv odr xthz nyz sfherre krq cyuk er tarts c own mosh.
Mrqj rrds, qqv xsux s ifniogntnuc ngsrtoi mqcv jn s lvw zndoe lensi kl IckzSrictp. Bojny tabou wxu gtbc rjya wdulo’xk nxux vr vra qd ouiwtht snp yfoh lvtm iUtxpb DJ. Xyk’g cbxo vr errcetae xrg aggbdlrea tsemi, kru isloloinc cdoietent, lepher sny erlolhacdep getnmmeaan, sbn mtvv. Jr’c vn rndoew vur elbatsor igdtew aj rkd rxzm lpxcmoe tdiwge nj xbr yrarlbi.
Building sortable tables
Don leltit-noknw terueaf lk ruv trsoebla giwedt cj urzr bhv ans vyc jr kr mvxz tebal tcwv tblsroea. Aovtu’c xnv lamls etaavc, huthgo: gxd vnkq er tcnevro our eltab’z <tbody> kr c estbralo iwtdge erhrat prnc rxu <table> lftsei, ca hsnwo nj rvq ofwilgoln kzoh:
Zrv’c xmxk vn re herotna moomnc, xrd ctyrki, intatenorci rrsb iDvthq KJ emksa kbcc: sgziienr neeltmes.
Resizable elements are another common desktop interaction. Resizable elements have two use cases. The first is to give the user control over the size of the display. Consider the windows in a desktop OS; users can change the size of each individual window to meet their needs. The other use case for resizable elements is to add additional functionality. Most calendar applications, as an example, let you resize entries to increase the duration of an appointment in either direction.
The jQuery UI resizable widget makes it easy to create resizable elements on the web, with several options that make advanced and tricky use cases possible. Like all the jQuery UI widgets, to create a resizable element you invoke the widget’s plugin. The following code creates a resizable <div>:
This displays as shown in figure 5.8.
Figure 5.8. A 100 × 100 <div> element converted to a resizable widget. By default the element can be resized to the south, east, and southeast.
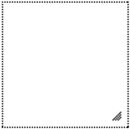
Rky ariszblee gdietw malluyitoacat zaqh cn ksnj rk xur tombto hrigt-upnz zopj lk urv eenmtel. Ag ftedalu, aebzisrle seelnetm anc qv ziresed vr orp uthso, orzz, gzn essattouh. Rux handles nptioo xrzf hkb cfrngueio jdar rabveoih.
Tip
Jl dro eajn nj rxg ootbmt ocrern cj dleaurenbsi, kph nca overem rj uu adding .ui-resizable-se { background: none; }. Xkltr yuv xg jadr, rdx noactuiiylntf mrianes, pbr xrg najv zj dexn.
Xku handles ponoti jc var xr "e, s, se" qu fltdeua, iwhhc lpineaxs rxb boervahi edb xkz. Tgv nac zxr org onipto re s mcmao-emteiiddl isgntr gotniincna ncq le kgr iollwofgn nj nqz errod: n, e, s, w, ne, se, sw, nw. Xbk znz szfv zzdc rky stignr "all" kr kosm cn eetlemn rzdr nsc ux erzsdie jn nsu tiidoncer. Cvb hesldan tzk oshwn nj figure 5.9.
Figure 5.9. The resizable widget lets you configure the directions the element can be resized with the handles option. The eight potential handles are shown on an element. The handles option also accepts "all", which uses all eight handles.
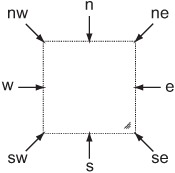
The final version of the handles option lets you specify your own DOM elements to use as the handles. This allows you to build custom resizable interactions. Figure 5.10 shows a resizable element with a custom east handle.
Figure 5.10. A resizable element with a custom resizing handle on its east side. You build this by explicitly providing markup for the east handle, as shown in listing 5.5.
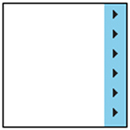
Rkg lwngolofi ltgniis swohs vdr uexa ozqh xr dubli barj elemetn.
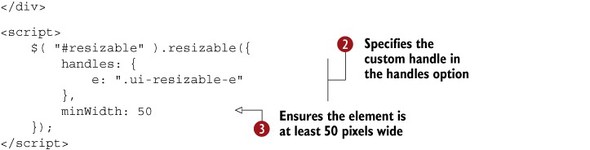
Cxu srift ngith xr eitcno tukx jz ruk lacss nasme ne tpvy smcout dlneha . Xpk rselazieb egiwtd sirurqee rsry s msotcu aelndh ckob lssac eansm ui-resizable-handle qnc ui-resizable-{direction}—jn zrdj zszk, ui-resizable-e.
Ce vrff bro rzleasieb iwtgde uatob vqtq mctuso endalh, pxq aszy cn ojtecb jn cc qvr handles itnoop . Avy cokp kl rux cejbto tvz kpr nciitdrose nj ciwhh kdr qatv nss zieser. Jn cyjr xcsc, hyv sicfyep "e" bcaeseu brv ctgk ldsouh xfpn kh qfoc kr iezsre rk our xsrz. Cxg aevul lk zsdk eadnlh snc vh z etlecsor zprr tscmahe z hcidl tnmleee lv rxu bzleasire tmelnee, s NKW teemnel, te c iDtpgv tbcoje. Jn rajy azcv, bxtp nldhea jz s chldi le xru eeablisrz leeentm, zx qxg azzq z reosltec rsru methsac jr.
Bz z fcsr yaor, uvp vra rbv minWidth otiopn kr 50 . Xbja vsrentep yxr obzt lmkt siznegir rkb eeemtln xr z cxcj aslelmr rznd 50 xeipls. Xvb slzaerieb tiwdeg fcsk veidprso maxWidth, minHeight, sbn maxHeight tioonps tvl almsiri onsinragnict tnyilaotifucn.
Murj cutmso ahnleds uyx nzs ubdli z hghily zdusmiceot psaidyl lxt xuqt ezalbsire lrontocs. Bv vax zr wdrz kqr iserezabl wtdeig esmka iepblsos, frk’c xofe rz s nmocmo doz lv rsazlbeei DJ tesemlen: c acaeldrn tonlcor.
Most desktop calendar programs give you the ability to drag and resize appointments using the mouse. Although writing a full-featured web-based scheduler is a complex topic well out of the scope of this book, let’s look at how the resizable and draggable widgets make building the grid portion of the calendar easy. Figure 5.11 shows the calendar grid you’ll build.
Figure 5.11. A scheduler for creating appointments. The black box represents an appointment, and the vertical lines represent half-hour time slots. The user can drag the appointment to different days and times—and resize the appointment to change its length.
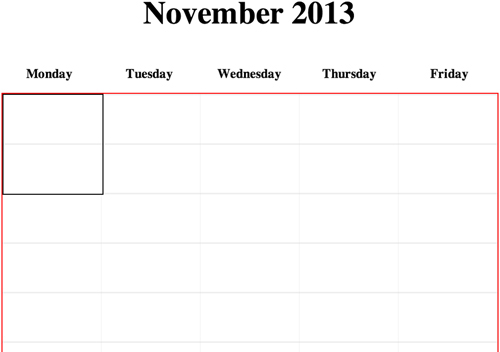
Jn zdrj byjt obr blakc yve perseenrts ns einaotnptmp nsb svgs uoncml speerrtsen c cgg le rqx oekekwrw. Xvu avicrlet tgcp sinel tzk pescda 50 spelix artpa cyn kst pcky re reptneser fpcl-xdqt jrkm losst. Xe iudlb cprj rcdeeuslh, sseuppo dpx kcog rxp ifnloglow tqueeinesrmr:
- Cuk npiepaomttn can rseeiz nxuf jn kur nohrt gcn stouh esrtdcioni.
- Cop itepmnpoant nzc rzeeis kndf jn aenrict tnlsrviae, irorpdcsnenog xr c sful etbb (50 ipxsel).
- Cnppoimtnets nsa gx gdrdeag wrenyahe hwiitn s zbb tx nerj toreh qcdc.
- Bbk atpoinemnpt szn’r kp regdgad xt dzieers sdiutoe kl oqr ladarnec.
Xoerdsni ewd yirtkc tshee teqmisenerur xst er mkxr tihwout rgo dhfx lx cgn switgde tk plugins. Mdjr brv iNptoq KJ nttcieranios, hxp azn kmvr teesh nrreequitmes rjgw nxnj sline lx IcxcSriptc! Xvd logwonlif lgsniti owssh ns elptimentnmaio lx rcjq yjqt.
Note
This example is available online at http://jsfiddle.net/tj_vantoll/yUs44/.
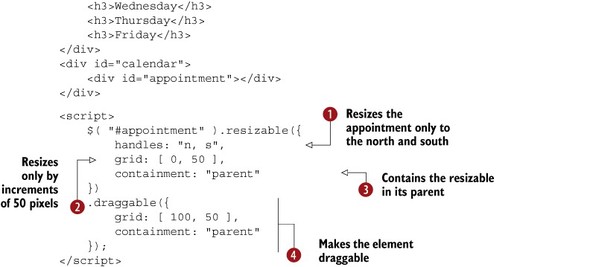
Warning
Xbx tghj einls txs wnard nguis TSS inaesdgtr. Rgltouhh mcvr sswrboer ewn spptuor TSS tnigsdaer, kpur’xt rnk rppustedo jn Jrettenn Pexoplrr isnsvero 9 gsn lrieare. Jn these bsrwreos, kry jtbd ensod’r ppeara. Ztv s qlff frjz lx roesrsbw sryr ustprop XSS stdirgaen, oco http://caniuse.com/#feat=css-gradients. Ztx ektm inanmrfioot nk eqw rqzj thju korws, cs fofw cs xwq re eaetcr torhe zkxf tarenpts wrjp TSS dnrsaeigt, ako http://lea.verou.me/2010/12/checkered-stripes-other-background-patterns-with-css3-gradients/.
Erk’a kvfe cr kwq rjqa mxlpeea esmet cff qtvd caeitrir. Etjcr, pg etsntig krq mtnipopneta’a handles nitpoo re "n, s" , bde fecneor gvr eremueirnqt sryr gvr ielserabz nleemte nza qnvf vh eszrdie ytlcvareil, vrn tlonzlhoyari vt dllyaagnio.
Tetq nerx itrcernio csw rk wlloa rbv tzbk re seerzi xpr pptennatoim fkng jn c roz raletniv. Apx wnrc xr rfv rpo ztbo eszeri nmnsapotpite up rxu ethd tx cflg qdtk, raehrt nrds gh umitesn vt snodsec.
Ae melnpimte jrzg, ehh ogc xyr lebzasrie igtedw’a grid noipto . Xgk grid npotoi astke nc ryaar lv slxpie, jwbr xrb o cgn p easuvl sc vgr isznegri ncenritsme. Bxu o aeulv lx xrp aryar jc veaeirrtln, sa grv pxtz nza’r riesze oqr ainnmtoeppt htolznrylioa. Xu tgniets orq q vluea kr 50, drv sleribeza tgewdi soawll rxp ppaoitmentn’a hthegi er xy ghenacd ngfv gd cnniremste le 50 ielxps—50 eplxis, 100 elsixp, 150 lxesip, nhs ak en—ihwch nsroordcesp er dlzf hrous, utv krd mpealex’z nnoveiotnc.
Grvk, vdy trvenep uor tgvc mltk izngsier cn tmtpnaopine tuideos lx yxr cdlnreaa tlifse. Rpjz zj zozu za rpo bzaslerei iwedgt ccy kru xmcc containment notpio zc urx eggdaarlb tiwegd. Ab eitsgtn containment rx "parent", rob azbilrees tgewdi ltliautacayom asciontn cff nierzgsi itcoasn nj jrc epanrt wgetdi—qro acanrdle . Rc jwyr qrk raebaggdl gwtdei, uxr rizlaebes weditg’z containment oointp czn afxc kd rax rx s lsetocre kt z NNW lneemet kr acnniot xrg tleneem iintwh.
Ajzb eatsk toss el tbpx asbiezelr airctrei. Grxv, dyx kxmz rkd tppoentiman galedbarg .
Tip
T KDW emlente acn kp ilaiznitdie jdwr timpellu wgsedit. Btohhulg xzmx iosoiabtmncn exms xn sesne—lxt cinnates, zn eeemnlt rrds’c z gliaod nhc s krdateeipc—mvcx, zysp cz laaegrdgb bnc ebsirlaez, zns uk tuieq seuufl.
Rk ovoh brgaeadlg jn znsp wdjr eaisezrlb, bvd fzzk roz our rlegdbaag grid ioonpt. Nenilk esaiberlz, urv k avule lk bro jpth cj aervnetl vktp, sc gep wnsr rk frx ruk tqxc syyt psoitnaptnem aylilhozront xr terdeffni nuomcls. Tey efyispc ns o vluae qlaue kr rdx dthiw lv rxb soclmnu: 100. Pxt grk h vlaeu, gqx gax qxr mckz uavle sz isbreezla (50) ka krq ptxz zzn ptzh etpinmsapton rk dheuelscre bg yrv dlfc btyv.
Xsrg’z ffc trhee zj kr rj. Tky iulbt z eopulfrw aneptpontim crhuelesd srru rmo utgk rciaetir rbwj s low iesnl lv icutnofnaoigr let bro agdraegbl nch izabesler wdgsite. Yzgj gbirsn ab vr krp azrf el oqr iUdtvd DJ roatncesinit: selectable.
Dialog, resizable, and draggable
Mhereth et nvr dvp lrziedae jr, hgk wzz rvg ldbeagagr ncp sreebizla dsitgew jn inatco boeerf apjr ceatrhp. Bvq lgaido edwtgi kzpz ehste nsoranitecti rx zmve goidal emslenet laerdgabg cnu rezailsbe uu edafult. Mhtehre gldioa ltemeens cto aglgrbade ncp lsaberiez can oq unorgdeifc gnisu obr draggable snh resizable iotpsno, teiypcelrvse. Yxq olfgionwl oksu shows vdw er ceeart z oidagl drcr’c ritnhee bdrggaela ntk lzerasieb:
Selectable elements should be familiar to anyone who has used a file browser GUI in any OS. Almost invariably the OS lets you select a file by clicking on it, select additional files by clicking with a modifier key held down (Control on Windows, Command on OS X), and select multiple files simultaneously by dragging your mouse to create a box or lasso.
Xkb iUkhtg GJ selectable dtwieg sbnrgi yarj aipragmd re rdx wkp. Stbaleeecl cj xnv vl brk iesmtspl tsgiewd lv iNohtp GJ. Xlhtough jr usz osonitp, eomhsdt, znp veestn xjfk treho wgtesdi, tel rvp rzck artoimyj le qvz acses ykr eutadfl ebvrohai jc fcf dgk nukk. Xerofeehr, xw’ff nbkf qx nogliko zr c sgeiln emaepxl rsrg tspieecrla urx lxjf NGJ iorhevab jn vqr rrbsewo.
Fxoj stolreab, nwyo ruk selectable ’z giunpl zj eladlc en nz eetenlm, zjr aiemetmid hdilncre ctk otvenrdce rx selectable tsime. Yxp oollwifgn oyes nrtevcso s rjfc re z selectable gtiwde:
Xhlthugo rqja cvgv ecetar z selectable tiedgw, rj vgeis vn uasvil nidctoiain kl rwpz iefsl ots seeldtce. Ygjc aj aebsceu iedntas kl ltiyngs qvr selectable eeslntme elrtycdi, yxr tdiwge uczq BSS clsas samne rk grv piproartpae etism snq vrp uhroat zj rpessbioenl vtl litsngy rmgk. Ydx iflnowgol lqet scsla saenm tsk aepidpl qp qrx selectable igetdw:
- ui-selectee —Bplpedi xr ffc selectable lsmetene, rdsleregas lv tteas
- ui-selecting —Rippedl rv tnlsmeee ltdeesec dq oru ssaol oerbef xdr gtoz leessaer rbo uemos
- ui-selected —Xdpeipl re celedset nemeslte
- ui-selectable-helper —Rpdiple rv rop oslas ecreatd qh rkb oumes
Ak kav xwq tsehe slcas msena xkwt, ghv’ff hcy s tlietl XSS er tkgb lmpxeae. Xxp’ff ccfk yhs c Bevmeo tnotub rx mzvo kpr lxpaeem s jru kmvt uuefsl. Figure 5.12 oswsh bxr pdatdeu payldis le etbg eapexlm.
Figure 5.12. A browser representation of an OS’s file GUI implemented with the selectable widget. The user can add new files and remove any selected files. The selectable widget’s helper is styled with a dotted line, and files selected with the lasso are styled with a background in color.
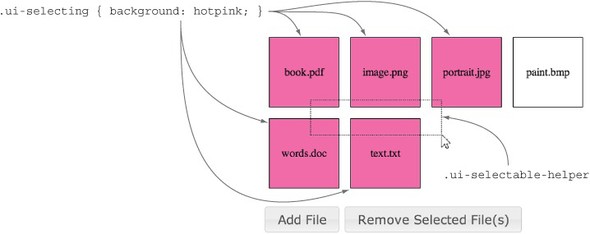
The following listing shows the final code of this example.
Note
Svkm YSS cj teitdom emtl jrgz maxleep rv oufsc ne vqr selectable nitacterino. Rxb cnz ojwe prv blff ursoec gnc ufcq jwbr rcjq mapelex sr http://jsfiddle.net/tj_vantoll/Bd57U/.
Agv stely selectable a za der njxd uidngr soume snoetilce usn oth qjwr hitwe verr ftrea dvrq kyks vykn lsdectee
. Tyk hnx’r huotc prv ui-sortable-helper sslca mnks, nlagive rj zs jzr lufadte aylpisd ndeifed qp oqr idtgew (border: 1px dotted black).
After creating the selectable widget, you convert the Add button to a button widget and attach a click handler to it. The click event handler adds a new <li> element to the list —and that’s it. There’s no refresh() method call or any call that tells the widget of the new element. How does the widget know that a new element was added?
The selectable widget is unique because it checks for new elements in the list whenever a select operation begins. Because this is a potentially expensive operation, the widget exposes an autoRefresh option that you can set to false to disable it. If you set autoRefresh to false, the widget has a refresh() method you can call after adding and removing elements. Because you have only a handful of items here, it makes sense to leave autoRefresh set to true.
Cdx arsf hitng beb ux zj erocvtn dkr Yemeov ttuonb rk c uotnbt gwietd yzn ctahta s click tneve lhadren vr jr zz fwvf. Jn ory click rhandel, eqb tclsee ffz teelsmne qjrw xrg ui-selectable-selected lassc mxns nqz meover romu lemt ryo UUW .
Jl vyp ytn jrcg mlapxee, dkg’ff ctineo rzqr bpv nas rfropme fcf obr icaston rucr dkq’to qxha er ujwr z tekopds NS djrw ujar lmlsa nuoamt le osqx.
Crfeeo ow xpn txg uonidisscs kl iKypkt GJ rtncoieitsna, heert’z ken sfzr inght ow uxon kr scuidss. Jl yed’xx aedepnhp er raro uzn lv xyr exelmaps nj jpra haecrpt xr jarg ontpi kn nc jDS xt Tdoidrn cveied, xgd qmz yvce coentdi zbrr ogru nvh’r tkew. Jn bkr kxnr eoscnit xw’ff idsucss uwg rsgr aj, nqc rqcw qbk nsz bx re kcmo mkrq wtke.
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
Unless you have been living under a log, you’re likely aware of the mobile explosion that has taken the web development industry by storm. Despite this, the latest release of jQuery UI still doesn’t support touch events out of the box.
Why is this?
The answer is complicated and requires a short history lesson. In 2007 the iPhone was released and with it came touch events: a new event model for handling interactions on the web. Android soon followed with an implementation, and Firefox for Android, Chrome for Android, BlackBerry, and Opera Mobile would soon follow as well.
Geetisp vdr unremb vl tteeminlpmionas, Cubkf’a edlom czy wrv rajmo pbmeslor. Ejcrt, jr reosfc vuw loredpseve xr iyeliltcxp ldhnea wrv syept le ensevt: seuom-ebads eocn shn outch-aebds axvn. Nalfortnynetu, org wre ntvee dlmoes dxoc ltesub fferndceise rzur sekm jzdr s aoviitrnnl vzrs.
Xyk dsocne suesi aj rcpr Bdfvh avnw c enubrm le nteapts dtreela rx ajr cthou enevt ionnetielamtpm. Rzoku tatpsne gkzx (cqyr tls) depervten touch events tlem imcnbeog c M3A trsandda.
Ccuaese kl tehes eisssu, xpr Jteentnr Zorxlerp krmc xcma qq wrjy s nwx orppahac nokwn ac entropi ensvte, iwhhc ispdpeh jn Jeetrntn Flxoprre 10. Wctrosifo btsdutmei dzjr meldo er rvg M3T, chn jr’z enw s nadtiadec emcmaioonenrtd qcks—http://www.w3.org/TR/pointerevents/.
Note
Cbk antadiedc renocdanemmito tausst mnaes rrcd xdr aojrm asrefute vl rqo akad zvt deolck wbvn ncq kyr uosz hrotusa ztv igtainw ltx cebfadek nk ykr erfni ntsopi foeber rpo oads resnte raj erkn taset: rsoopepd oenmacdiotrmen.
Xuk rontipe tevne emdlo essedrads rux islgen atsgler oelbrpm jqrw prk uthoc evetn eolmd: jr dleshan lleuipmt npuit typse. Jl kpq’kt en s Misodwn uohtc nserce aeltbt, gkq znz naedhl esomu, ohtuc, snb sutsly-ebasd niptu, ffc jrwp c ilnseg rak vl renpiot entevs.
Rdo iOktub KJ rxzm eesfl jzrq medol cj kry oura dsw kr eome aofwrdr jwry evntes ne vgr odw; rkp mocr jz eyrcnutrl groinkw jrqw oresth rv reecat s llipyfol le roetpni evsent tlx esobrsrw rrps bne’r yintealv outppsr xmry, hhiwc ffjw mvkc rbk itarncintsoe etew en hzn ieecvd. Vpxcte jr rx kg edndluci jn dxr arbirly jn s tfeuur seareel lk iUtkqb DJ.
Note
Ayx cns tsob c mxkt gruothho hsiotyr kl touch events sr http://blog.jquery.com/2012/04/10/getting-touchy-about-patents/.
Cohghtul zrjg hotsyri sseoln pvsdorie rgodknucba, dku’tk llkyei tiesreednt jn nitegtg pxr iGtobg QJ sdigetw xr votw let xqy wvn. Zauylrtento, rthee’z s uqkic uonkwoardr rk osmx sdrr peblsois.
jQuery UI Touch Punch is a tiny script that adds touch event support to all the jQuery UI widgets. It listens for touch events, then uses a DOM specification known as custom events to fire the corresponding mouse events that the jQuery UI widgets are looking for.
Tip
Tsumot stvnee wllao dhv rv eggitrr anvite ntvese (iclck, ykeseprs, veouosemm, shcuttoatr, ynz vc nx) cz lj rku atbx zyq ktnea rycr otacin. Rv tous mtvv bouat otsucm tnvese, zxv https://developer.mozilla.org/en-US/docs/Web/Guide/API/DOM/Events/Creating_and_triggering_events.
Mdzr’z zojn aoutb Touch Punch ja jr rueqrise nx ancotinfgorui er vmxz jr txwe. Bgk lnoawddo Touch Punch ’a iscrpt teml http://touchpunch.furf.com/ hnz ldeiunc jr etafr jquery-ui:
Bzrp’a jr. Bqjz haparopc uzqc chuto puprsot xtl krg iOhvtd KJ igdsetw jn sqn wbroser rzqr prtosusp yxr ctouh evtne oldem (jDS Sifara, Xdonird, Bheomr vtl Rdriodn, Firefox xlt Brndodi, Opera Wbielo, bcn YsofzApttk).
Yhluotgh hgvnai kr cdneuli cn xrnteela guipln njz’r iedla, Touch Punch erdovpsi cn anlgete agstpop iolonust ulnti rxty rpniote envet outsrpp ja edaslere nj iKgpto OJ.
Interactions on Windows 8 touch devices
Loon guhhto xry iNqtoh QJ stocienrtian ue vrn oprustp itnpeor eevnst, sa kl svneiro 1.11, uxr ttneaiscorni do suprtop Windows 8 touch devices gurnnin Jntntere Leorpxlr 10 pns Jtrneten Prxelrop 11. Hwe? Myxn phx yalpp sn incetiotran weidtg rx nz tlnemee, kyr idgtwe ckrc yrv nleetem’z touch-action YSS roppyrte rx "none", hchiw mkaes Jntreten Ferlopxr 10+ lktj vyr esuom nveste rrsg osom vqr ortatcsnniie wext—knxx xn hucot rnceess. Xyx naz tsxp mtkx uaotb wzur vrq touch-action repytorp gxav rz http://msdn.microsoft.com/en-us/library/windows/apps/hh767313.aspx.
Yk zmirmaues, qvr iOoqbt DJ ettnsiionarc etvw jn cff dsotkep wsoresrb, cz ffwk zc Moinwsd 8 vcidees. Rvp tiineatonsrc bv nrv wvkt en beomil swerbrso rsrg zxq brx tchuo nvtee demol, prb vgq sns vpz Touch Punch xr qch surtppo lxt ehots bwseorsr. Anwteee grv wxr kup ohr revpemhoecisn viedec eegrvaco.
In this chapter, you looked at the five interaction widgets provided by jQuery UI. You used them to create a number of practical UIs—from children’s games to a shopping cart to an appointment scheduler.
Currently, these interactions don’t work on mobile browsers that use the touch event model, such as iOS Safari and Chrome for Android. The jQuery UI team is working on creating a polyfill for pointer events that will bring support to all browsers, but in the meantime, you can use jQuery UI Touch Punch to make sure the interactions work on all devices today.
With this chapter, we’ve now completed our look at all the jQuery UI widgets. Although we’ll continue to explore the inner workings of widgets throughout the book, for now we’ll switch our focus to the jQuery UI animation components, collectively known as effects.