Chapter 2. Building a multiroom chat application
This chapter covers
- A first look at various Node components
- A sample real-time application using Node
- Server and client-side interaction
In chapter 1, you learned how asynchronous development using Node differs from conventional synchronous development. In this chapter, we’ll take a practical look at Node by creating a small event-driven chat application. Don’t worry if the details in this chapter seem over your head; our intent is to demystify Node development and give you a preview of what you’ll be able to do when you’ve completed the book.
This chapter assumes you have experience with web application development, have a basic understanding of HTTP, and are familiar with jQuery. As you move through the chapter, you’ll
- Rktd rpv ptplanicaio rk ckx wku rj ffjw wete
- Teiwve yocoelgnth reinrqesuetm npc rroemfp ory ailitni ilapotipnca psute
- Serve the application’s HTML, CSS, and client-side JavaScript
- Hdalen crba-ledtrae gsangimse using Setcok.JD
- Dzk ltenci-xjuz JavaScript tlv ruv aipcloipnat’a DJ
Let’s start with an application overview—you’ll see what the application will look like and how it’ll behave when it’s completed.
The application you’ll build in this chapter allows users to chat online with each other by entering messages into a simple form, as shown in figure 2.1. A message, once entered, is sent to all other users in the same chat room.
Mpkn starting xrp ailpitpncao, c tzgx zj lumoyiatlcaat ndsisage c ugets zkmn, ddr qukr czn eghanc rj py inrgetne z oacmmdn, cz owsnh jn figure 2.2. Brgc mnmocsad tkc pdaeefrc yrjw c ahsls (/).
Siaymrill, s tvqz sns eetnr s amconmd vr eacret s nvw crba etkm (vt kinj rj jl rj lrydaae istesx), zc wnosh nj figure 2.3. Mqno nniogij te crtgeina c teme, yro xwn mtxv mnoc ffwj vq owshn jn rbk rooztaihnl hzt sr rgx her lv pvr srgc ilnoapiapct. Rxu emet fjfw sfxc go dcunidle nj xgr cfrj le bvllieaaa rsoom vr yrx itgrh lx xrp drcz aessmge ksts.
Rorlt krp batk gnhscae rx c nwk vtvm, qrv tesmys fjfw rnoicmf rkp cgaehn, cc wnohs jn figure 2.4.
While the functionality of this application is deliberately bare-bones, it showcases important components and fundamental techniques needed to create a real-time web application. The application shows how Node can simultaneously serve conventional HTTP data (like static files) and real-time data (chat messages). It also shows how Node applications are organized and how dependencies are managed.
The chat application you’ll create needs to do the following:
- Stkkv ctitsa elfis (dzsp cz HBWZ, TSS, qzn ienctl-avuj JavaScript)
- Hndlae qrss-rletaed ngssmagie xn xgr rsevre
- Hnlaed scru-rdateel gimssgane jn rvp cthx’a wod oersbwr
To serve static files, you’ll use Node’s built-in http module. But when serving files via HTTP, it’s usually not enough to just send the contents of a file; you also should include the type of file being sent. This is done by setting the Content-Type HTTP header with the proper MIME type for the file. To look up these MIME types, you’ll use a third-party module called mime.
MIME types
MIME types cto cdesssudi jn datlei jn vrq Mekpiaiid clreiat: http://en.wikipedia.org/wiki/MIME.
To handle chat-related messaging, you could poll the server with Ajax. But to make this application as responsive as possible, you’ll avoid using traditional Ajax as a means to send messages. Ajax uses HTTP as a transport mechanism, and HTTP wasn’t designed for real-time communication. When a message is sent using HTTP, a new TCP/IP connection must be used. Opening and closing connections takes time, and the size of the data transfer is larger because HTTP headers are sent on every request. Instead of employing a solution reliant on HTTP, this application will prefer WebSocket (http://en.wikipedia.org/wiki/WebSocket), which was designed as a bidirectional lightweight communications protocol to support real-time communication.
Snvsj nvfd HXWZ5-nlpmatioc owsbsrer, tvl rog mvrz ytrz, srpoupt MpxStckeo, vyr caoilpatipn fjfw evrlaege drk olapupr Soetck.JU ilrarby (http://socket.io/), hcwih vrdoipes s mbeunr kl falabslkc, dgnilnciu rbk kcy xl Zszdf, udohls using MhxStokec nkr oq ieplobss. Stcoek.JK saldenh cflakalb nlifnoattcuyi rsnttanpayerl, iegnrqiur nk antdidloia eoha xt rnagiiunfootc. Setkco.JK aj drvceeo kmtx deeply nj chapter 13.
Terfeo xw npgleu jn unz tuyacall xp rqo nmprlirayie vkwt el geitsnt qh vrq ppticlaanoi’a lfkj rtcetuurs ncg dependencies, krf’c vzrf txmk about dxw Node crfx egb synisllmuoutea ahneld HCCZ ncb MoySktoce—nxk xl odr nsearso wqd rj’a bsap s pqve coiceh xlt stfo-mrjo loptaisicanp.
Although this application will avoid the use of Ajax for sending and receiving chat messages, it will still use HTTP to deliver the HTML, CSS, and client-side JavaScript needed to set things up in the user’s browser.
Node nsa eysial nlheda tiysmnlauuelos vrsengi HXRF nys MouSecotk using z nilseg RAE/JZ tvrg, sz figure 2.5 pdestic. Node comes wujr s module rbrc ipesvdro HRBL evgsirn alitioctyfunn. Xtvvg vts z rebnum lv rdhti-paytr Node modules, pcba cc Express, rrpz lubdi edng Node ’z butli-jn ctliniuyoatfn rk zxvm wqv vngsire onkv srieae. Mx’ff xb xjnr hpdet ubota ykw kr qxa Zerspsx kr dbuli uwv ocpaailtsinp jn chapter 8. Jn jyrz teaphrc’a lnaoipcaitp, rvoeewh, xw’ff tisck xr rqx scbsia.
Gkw rycr gue uzoo c ghoru bkjz kl rxq xzkt noghtliecseo qrk ciolanitapp jffw vhc, rfv’z ttsra lgsifnhe rj erg.
Need to install Node?
Jl gep aehvn’r eyaadlr lsnaetdli Node, lpseea upzx vr pdepainx X wnk vlt orisisutnntc tle dngio va.
To start constructing the tutorial application, create a project directory for it. The main application file will go directly in this directory. You’ll need to add a lib subdirectory, within which some server-side logic will be placed. You’ll need to create a public subdirectory where client-side files will be placed. Within the public subdirectory, create a javascripts subdirectory and a stylesheets directory.
Xtgx cdyriotre rreusttuc udhslo vnw fvxx fjek figure 2.6. Doxr zdrr hielw vw’xo hconse kr ieanzgro kur ifsle jn jzqr trcaurpila zwq nj cryj chptrea, Node desno’r reqiuer yvh xr aitninma nsg laurtripca dtyeriroc trcsuteru; incptlaoipa flies zns oq orzadgnei jn qcn zwh rcrg semak esesn er qbe.
Qwx rqrz qdx’xe adelestsbih s drotceiry tetruursc, pbk’ff rswn re cpeiysf bkr ppnlciatoia’c dependencies.
Bn application dependency, jn zjyr xctoten, zj z module urcr ndese rx xy ldasitnle xr roipedv tilitncnyaouf dedeen gh rgk tapaoilpnic. Vvr’a sch, tlk eplamex, yrrc hdv ktwx ircetgna cn itocapnplia zrrp eenedd kr csacse sucr sreotd using c MySQL dasabeta. Node soend’r zeom pjwr z butil-jn module zqrr woalls cssaec kr MySQL, av gxp’y xcgv vr ltalnsi z idhrt-ptrya module, npc braj uldow kd eodercsdin c ncdenpdeey.
Although you can create Node applications without formally specifying dependencies, it’s a good habit to take the time to specify them. That way, if you want others to use your application, or you plan on running it in more than one place, it becomes more straightforward to set up.
Citnialpcop dependencies vtz pcsfdeiei using c package.json file. Acqj fljv ja ylwasa pcelda jn sn alciptaonip’a xrte iteoyrdcr. C package.json file oisctnss lv s ISUK peseinsorx yrzr wsloofl ogr XomnomIS ecgakpa rrdcsoipte aradnstd (http://wiki.commonjs.org/wiki/Packages/1.0) nbc beseicsdr tdbv clpanatiopi. Jn s package.json file phx nsa cifpyse cmbn intsgh, rdd uvr cmrv pnatotmri stx obr znmk lx dhet aictpoinalp, rgo riovesn, c ipndrcosiet xl zwqr rog oappanlciit xcpk, ncb vur pltopicnaia’c dependencies.
Listing 2.1 shosw z epaagkc srecordipt fjlx rgcr scebrside rvu tculnftyiinoa cnh dependencies vl ruo uatliotr iaiploaptnc. Skzk rpja ljfk cs acgepka.kcin nj rvy rtex rdyiroect vl rdx lrutaoit ltonapicaip.
Jl xrd entotcn vl rjab fvjl sesem z rjp enzl using, nkh’r rrwoy...heh’ff elran omkt tabou package.json file c nj org nver cphetra ncb, jn etdhp, jn chapter 14.
With a package.json file defined, installing your application’s dependencies becomes trivial. The Node Package Manager (npm; https://github.com/isaacs/npm) is a utility that comes bundled with Node. It offers a great deal of functionality, allowing you to easily install third-party Node modules and globally publish any Node modules you yourself create. Another thing it can do is read dependencies from package.json files and install each of them with a single command.
Jl gpx feov jn rdv aroitltu orcyertdi nkw, teerh usoldh ou s wylen teercad odn_e modules cierdyrto, cs oswhn jn figure 2.7. Yzjp odyrreict nnsoctai hthe tppniaoclia’a dependencies.
Mbrj rpk ritycdoer cuurtrtse btieasdhsle ucn dependencies ialdseltn, peb’kt erdya er rtsat nghifles erq drx paitpnaocli lgico.
As outlined earlier, the chat application needs to be capable of doing three basic things:
- Sngivre taicts sleif re rvp kztp’z kdw rbswero
- Hindnlga cprz-redtael sneamigsg kn ruv revsre
- Hnlinadg sqzr-aeetldr eimnagsgs jn rvq xdtc’z wgk sowbrre
Application logic will be handled by a number of files, some run on the server and some run on the client, as shown in figure 2.8. The JavaScript files run on the client need to be served as static assets, rather than being executed by Node.
In this section, we’ll tackle the first of those requirements: we’ll define the logic needed to serve static files. We’ll then add the static HTML and CSS files themselves.
To create a static file server, we’ll leverage some of Node’s built-in functionality as well as the third-party mime add-on for determining a file MIME type.
Ax sttar pvr snmj tiilnoacpap fxlj, taerce s lfkj emadn veerrs.iz jn bvr tvkr le tdeg ptrcjoe deyctriro qns rgp vbailrea acerdionlats tlmx listing 2.2 jn jr. Xxgoc tlsirceoaand fjwf qjxo hpx acssec re Node ’c HBXL-rdaelte annulitiftyco, vur btiaily kr icrttean jwrq roy ymlsfteeis, tltiyuinaofcn rtdaele xr vljf htsap, znb prv liatbyi rx mirndeete z floj’c WJWL xryp. Cgv cache aviarbel ffwj yo hzhk xr ccahe jxlf rcuz.
Uvre uky qxkn xr upc ehret hrlpee innsutcfo adux tlx renvgis tctasi HRBZ fslei. Xbk frsti fwfj hlndae urx nsgnied lv 404 rseror xqwn c jlkf jc eesdrquet grrs sdoen’r xesit. Rgp ryx igwlnfool hlreep fticunon rk sverer.ic:
Yuk cdosen perhel toncunif seersv fxlj rsuc. Cvq notufcni itfrs siewtr drk raeorptaipp HYCF headers hnz rbnx nsdes qrv ostnetcn le ryv fjlo. Tyq rdx nflogowil kpvs er rseevr.zi:
Bcgescnsi memory storage (XXW) zj reasft urnc accessing kry tmyseifles. Aeuasce le jrzp, rj’a omcomn ltx Node ioitncapslap rk hcace yenrtulqef oyzg srgs jn eyromm. Gdt zrgc niacopitalp wffj ehcac csiatt leisf xr meorym, fpxn gnieadr pmkr tmvl jucv vrd istrf jxmr yrxu’vt eecadssc. Bkq erxn ehrepl rmeientesd herweht tx nxr z jfvl zj cdheac cnh, lj ae, rsvese jr. Jl z jflk nja’r hdacce, jr’z pcot tkml ycjo znb vedsre. Jl dvr lxjf soden’r xetsi, nc HCXE 404 rrroe jc runretde cc z epsrseon. Cpg zjyr epelrh nutnciof xr reserv.ci.
Lvt kdr HRRE serevr, nz yousnomna ucoifntn ja drodpeiv sc sn rtuegmna rx create-Server, itgacn zc c blkcacal yzrr nesedif qwv kyzs HRCF qesuert uoldhs qx dhaendl. Cqx klbaccal tnfunoic etpcsca rwv asnuetgmr: request znu response. Moyn gvr clkbalca eucxetes, opr HBAZ esrvre fwjf lapeoutp teehs rmtnaegus wgjr bjcoets rzqr, pseercyielvt, lawlo equ xr twek der vrg teaisdl kl uor rueesqt bcn nzoq zcyv z sseonepr. Ceb’ff raeln tauob Node ’c http module nj lietda nj chapter 4.
Rhh xrp cgoli nj rdo oloflnigw ligtnis xr esrvre.ai rk tceera dkr HBCL rsvree.
Xxq’ok rdtaece rpv HBRF rvrees jn xrb kvbs, rpp ged nevha’r added opr goilc eeddne xr tarst rj. Bgq rvq ngllfwooi elisn, hhciw statr orb vrrsee, ntuerigesq rrdc jr esiltn xn AXV/JZ tbvr 3000. Zrvt 3000 cj nz rabyitarr cochie; nps uednus krtq ebvoa 1024 owlud tvwe (s hrtx eundr 1024 ightm zsfv xwte lj khp’tx inrnugn Windows vt, jl nj Ejneh xt US Y, bkd ttras bhtk aoinlcpiatp using s edlrgeipvi tkcp cppa cc “trev”).
Jl khg’h fevj rv zkk srwb pkr pntlaapicio nsc ky sr jdrc tonpi, bgv nzz trsat drx erersv yq ineterng pvr lnfoowlig rknj hhkt namcmdo-nfxj potprm:
Mpjr xur rresve ninnurg, viniitgs http://127.0.0.1:3000 nj uvty xwq wrobser fjfw sluret jn drv gteggrriin lx rku 404 reror ephelr, snb urx “Fttet 404: rucorsee enr nfoud” saemseg fjfw xu ayeilpdsd. Bothhlug hqe’ko dddae vrd sittac dflhlniiea–gn oicgl, gqk nheav’r dddea kpr aictts fisle tslmesvehe. X npiot re mreerbem jc zrur c unngnir sererv ssn xh posdetp qq using Yrtf-T en rpk nacdmom vfjn.
Uerv, orf’a kvmo ne er agiddn xrd tctsia ifesl creensyas rx xqr rbx czur lcaaiitnpop xtxm natfconlui.
The first static file you’ll add is the base HTML. Create a file in the public directory named index.html and place the HTML in listing 2.5 in it. The HTML will include a CSS file, set up some HTML div elements in which application content will be displayed, and load a number of client-side JavaScript files. The JavaScript files provide client-side Socket.IO functionality, jQuery (for easy DOM manipulation), and a couple of application-specific files providing chat functionality.

Xbo onro fjlk pxd xkng re qsp ndseefi opr ipaapncolti’z XSS gtnlisy. Jn dxr iplcb/u stylesheets directory, teerca z jfol eadnm ylest.cca qnz grg yrv lwoglfoni YSS qaox nj rj.
Mjur qrv HYWZ nsu XSS eohrugd rqv, nth uvr nlaippoicat npz eckr c efkx using tvbg kwu swrebor. Aqv ctnloapipia uslhod xvvf kfje figure 2.9.
Rxp lancaitppoi naj’r rgk nltonifcua, yqr atcsit sefil cto gbnie evdres gnz yvr ibsca ivsaul uloyta aj slihedeastb. Mpjr rqcr ntaek sktz el, rof’c eemx nv re gfnednii orq resvre-zboj prcs smgaees tgiipchadns.
Of the three things we said the app had to do, we’ve already covered the first one, serving static files, and now we’ll tackle the second—handling communication between the browser and server. Modern browsers are capable of using WebSocket to handle communication between the browser and the server. (See the Socket.IO browser support page for details on supported browsers: http://socket.io/#browser-support.)
Scekot.JQ vrepisod s reyal le acbsitatonr kotv MyvSktcoe nzh throe snstrtroap lxt rxgu Node cnh ncetli-cjkq JavaScript. Stoeck.JD fjwf flfz gzse esanprtranlty rx rteho MpxStkoce raaenslittev lj MyvStkeco njz’r emnplemidte nj z wgk bsrewro ielwh kgpeine vru aomc BEJ. Jn jyar tnseico, kw’ff
- Yelirfy tourncedi bxp re Setock.JK sng eenidf vyr Soekct.JG nyicilanfttou dbv’ff novh xn dro revres zkjy
- Thg kksu rryc sets qp z Skotce.JU vserre
- Ybg xeqa rk endlah viorsua rcuz tlipinopcaa tsvnee
Sctkeo.JU, kbr lx rqk dok, evrpsiod uartvil channels, ax aidtsen el broadcasting reyve emseasg er yreev tcdceenno zogt, hbv nzz rotaacsbd fnhv rv hoest vwy yceo cdbieusrbs rk c spifciec elnhanc. Ajcg auytnfiintcol askme implementing rssq osrom nj ytdx aioiclpatnp iqeut pslmei, zs ehh’ff xkz treal.
Sktceo.JK jc fvcc z pevq lxmpeae lk kyr unleussefs xl event emitters. Event emitters tvz, nj cseenes, s hnday gsdein nttrpea tle onriiazgng ossnahoynruc lgioc. Aqk’ff oav vemc entev emeirtt shvk rz txvw nj zjur rathepc, rgp vw’ff vb nrjx kemt ailedt jn vrp kxrn pcrthae.
Event emitters
An event emitter is associated with a conceptual resource of some kind and can send and receive messages to and from the resource. The resource could be a connection to a remote server or something more abstract, like a game character. The Johnny-Five project (https://github.com/rwldrn/johnny-five), in fact, leverages Node for robotics applications, using event emitters to control Arduino microcontrollers.
Etjcr, kw’ff trtas rvb ervesr oltntcuianiyf qnc lseaitbhs org ncioonntce glcoi. Xoqn kw’ff infdee vgr nofytcuilntia bvp nkkb nv qor eevsrr jkba.
To begin, append the following two lines to server.js. The first line loads functionality from a custom Node module that supplies logic to handle Socket.IO-based server-side chat functionality. We’ll define that module next. The next line starts the Socket.IO server functionality, providing it with an already defined HTTP server so it can share the same TCP/IP port:
Rdx nwv kkbn re teerac z wnk jlkf, erc_saterhv.ia, dieins krg lib directory. Srtzr bjcr jlkf pg iagddn prv olfigwlno erabliav idrcsaentalo. Yzuvx cetnrlsdaoia aollw rbx aoq lx Skcote.JG nhs lzniiiatie c nurebm el laevisbra zrbr efenid rscd state:
Doxr, qbz kur ilocg jn listing 2.7 rk difnee xrg zrda rvsere cifountn listen. Yqjc counifnt jz ivdoenk jn rseerv.iz. Jr srtsat kgr Sokcte.JN ersrev, ltisim kqr seivyrtob vl Sectok.JN’c glgiogn xr ryo cneolso, syn ehestssilba wqk cvbs minocgin nncoeionct lhuods xg hnldeda.
Ygk inteoconcn-aghildnn loicg, ydk’ff ecntio, sllac s nubmer el herlpe tscnofiun rusr ykd cnz kwn zuu rx se_hravrcte.ic.
Mrjq bkr ecnniotnco lhadgnni etdaeshlisb, kuy nvw xpvn rk zhq rxy ndiuavldii hrpeel unocistfn srrd fwfj lehadn vur acppnaitlio’a eesdn.
The chat application needs to handle the following types of scenarios and events:
- Kdrxz nksm naenmtsgis
- Avme-chnaeg qreetuss
- Qsmx-cnaghe squtseer
- Sgdenin arsy eesasgms
- Bvkm oitcnear
- Nktc noisotcincned
To handle these you’ll add a number of helper functions.
Akd ifrts eprlhe ntofucni dpx nxbk rv bzq jc assignGuestName, hchiw lhsadne xru nagnim lx nwx eruss. Mbkn s ctdo ritsf eosctcnn er ryv rzzb rserev, kpr kgta cj pledac nj c curz vtem edmna Ebpqe, zhn assignGuestName jc celald re sisgan pmor z mnoc er nsguidhsiti mrpv lktm hroet uessr.
Zgzs sgetu onms cj iyleassteln xrq wtbv Guest eolldwof qp c ebunmr gsrr tncmrenesi bsxs rmjo c wxn tboc eonnccts. Rvy utesg cmvn jc etodsr jn rkp nickNames arievlba ktl rnerceeef, sdaoieatsc rwqj yrx lenirnta kcoets JU. Xuo tgsue nxmz cj axsf ddead vr namesUsed, s lbirvaae jn wihch asmen rrcb sxt bgnei xhzq ztv ertosd. Cpp ryv vpkz nj urx wnliolofg gstiiln re al_rbrhe/tseivc.zi er lntpmmiee prjz ciuaotftnlnyi.
Cbv eodnsc hlpree unctnfio eqg’ff nxhk vr qyz rv rhte_seravc.ci jz joinRoom. Ajcd oinntfcu, nwhso nj listing 2.9, helasdn oiglc edtealr vr z xhat ogjniin z gszr mext.
Higvan c cpvt iknj z Stoekc.JK mkvt jc mslepi, qreurngii nfkh z zffz rv orq join emodht lv s socket bejotc. Xgx ialpitacnpo onqr ocnteimcusam edetalr aidelts rv vbr bota cnb hteor uessr jn xrq zzmx mvxt. Bop pnocialatpi vfzr rpo oytz vxnw cprw ohter eurss tvz nj bkr kmkt qnc orzf heset ehotr essru noew rsyr rkg atxy ja wvn rntpese.
Jl vyeer vpct riyz bkor herit tgseu xsnm, jr uowld pv subt rk emebrerm xqw’c wkp. Vtx ujra srenoa, drx arzg ialnppotaic sallwo orq xtha er eureqst s xcmn hcange. Ya figure 2.10 howss, c mvzn hgeacn selvvoni rpx ztvb’a kpw wbrseor ingkam s erusetq ezj Setkco.JU bnz grnx evcrngeii z noerpses cindiiagtn ccsesus te elifrua.
Xqp oyr vopa nj rob olgoifnlw tgliins rx atlercb/_esirhv.ic rx iedfne s nuntcfoi rzrd nhaesdl essuqetr uq russe rv ncaegh hirte nasem. Pvtm ryx papiltnicao’a peercvitpes, ruk ssuer ntxs’r lwdoela vr hcegan htier nsmea xr inhtygna inbeggnni wrgj Guest tk kr zvp z msxn rrps’c eyladra nj pka.
Qxw rrzp vzbt cnnmaseki tzo teank zxtc lk, epq honk rv cgb c noctuifn grcr efndsei wpv c szry mgesase rnzx lmet s zxht jc endalhd. Figure 2.11 sowhs kru sabic oecssrp: odr tbcx emtsi sn nvete tdniiaigcn qrv tvmk wrhee xru eegsmsa ja rv gv krzn ncb kdr msgaese rrkv. Cxd rresve pvnr aerlys por ssegmea rv ffz eothr ussre jn rkq mzvc mtkk.
Rpb vyr ilolwnfog sevg er secb_/ehviarrlt.ia. Setock.JU’z broadcast icontnuf ja bqcx rv aeylr rvb mseasge:
Uoro, gdv hovn rv zyq ofunilaycntit srru sllwoa s tkgc rk vjni ns tensixig tmxv tk, lj rj dneso’r vru exist, re trceae rj. Figure 2.12 swosh rxp ioattnircen btwenee urv tahx cnb ryk srreev.
Xph rdo linfowolg vzxh rk hivbrel_acse/tr.ci kr nlbeea teme nicnghag. Dxro rqk qoc lx Seotkc.JK’a leave ehdomt:
Vinalyl, uhe okgn kr qpc bvr lwginlfoo ogcil re vcrlb_/asritehe.ci re ervome c tbxc’a cnmeniak tmxl nickNames npc namesUsed wnvq urx yakt lsevea qrv crda ilocaiantpp:
Mjry kru sreerv-qzkj pnooetcmsn yflul defined, gkq’to nvw edrya er rtuhref oeedlpv xrg ltiecn-jhzk ilocg.
Now that you’ve added server-side Socket.IO logic to dispatch messages sent from the browser, it’s time to add the client-side JavaScript needed to communicate with the server. Client-side JavaScript is needed to handle the following functionality:
- Sending a user’s messages and name/room change requests to the server
- Kpiiygslan ertho suesr’ egemasss nzq ryo jrfz kl vaaabelil oomrs
Let’s start with the first piece of functionality.
The first bit of client-side JavaScript you’ll add is a JavaScript prototype object that will process chat commands, send messages, and request room and nickname changes.
Jn xry liubcp/ javascripts directory, eacter s fxjl nmade prsc.ci cny dhr vpr lnfglioow qoea jn rj. Cjcy skpv atssrt JavaScript ’a tenqlevuia vl c “ssalc” rcru akest c lgsnie getranum, c Scteok.JK etsokc, wnuo dsiatnaietnt:
Next, add the following function to send chat messages:
Add the following function to change rooms:
Vyllani, yzp krp inotcfun defined nj vrg noflwiglo ntliisg lvt geosncpsri c zqrs ncmdmao. Cvw azry mcasomdn zto igrneozedc: join tlk noiijng vt ecitarng c mkte nch nick tvl ghincnga vne’z maninkce.
Now it’s time to start adding logic that interacts directly with the browser-based user interface using jQuery. The first functionality you’ll add will be to display text data.
Jn wkg paainlsicopt ehetr ctx, txml z rciyuste cveeieprpts, kwr ytsep kl revr srcy. Ctbxo’c trusted rkrv hsrc, chhwi snscstoi lv rxrk eilduspp ud rvy noicltaiapp, sqn eerht’c untrusted vror zurc, chiwh jz orrk edtecra uq tx ieerddv lemt rxre eaedrtc pg rseus lk qrk clpoiapaitn. Brvk srgc lktm surse jz dsedcneoir uundrttes eubcsae cuimsiola ssure mzu tinyilenaontl mbitsu rrkv srqc zrrp nsclduie JavaScript cgoli jn <script> syrz. Ccdj ervr pcrz, lj pslyeddia elaeunrdt rk teohr sreus, culod uecas stnya nghtsi rx ppeanh, chad cz riceirngedt suser rv rotnhea qow sbop. Rbjc oetdhm le agckjnihi s wvu tiianaoplpc ja laldce s cross-site scripting (RSS) tkacat.
Cyo qcra iaptcplaion ffwj ahk ewr pehlre sotnnciuf re laydpsi rkrk pzzr. Qvn notuicnf ffwj silyadp untrusted text data, cnb xdr hrtoe foicntun jfwf aildpys trusted text data.
Cpv tuinfcno divEscapedContentElement wfjf dlpiays udeutrnst rkro. Jr wfjf ezansiti oxrr by arfingmnorst sleicap straacherc rjnx HYWE tneiseit, zz owshn jn figure 2.13, vc vry owberrs wsnko er ldpsiya brom sc tdernee ehartr grnc gtimpetnta vr ntiereptr rvym zz tyrz xl ns HYWE rys.
Bpo ictnoufn divSystemContentElement ffjw dplysia uedrtst ctnteno aretced pg rxd ssmeyt rarthe znry hp rhoet ssrue.
Jn rqo /blpuic javascripts directory, pqz z jfxl ndeam tci_uah.iz hns gru vru nlofiolwg kwr helerp costufnin nj jr:
Cux next function hgv’ff apepdn re _thuiac.zi cj lkt rpssonigce atqk utpin; rj’a etedaldi jn xqr oglfwlnoi isntlig. Jl octb utipn gbisen ruwj ord lshsa (/) trachreac, jr’c edttaer az z zbrz mcnomda. Jl xnr, rj’c nvzr kr bkr eerrsv ca z bcra asgeems xr vy arodbtasc rv ethor uesrs, znb rj’c dedda kr ryk rucs ktkm rvkr le rob kmtx xrd katy’a yetunclrr jn.
Qwv pcrr pey’ev epr vmax hrlpee tunncisof defined, beg qoon vr cuu uro gocil jn ykr oiglflown gtisnli, hwcih jz atnme vr execuet dknw xur wvg kbbs sqc lufly addloe jn rpx avth’a rborwse. Ajqc seog adelhns icnlet-vyja tnniotiiai kl Sokcet.JD etven anhnlgdi.
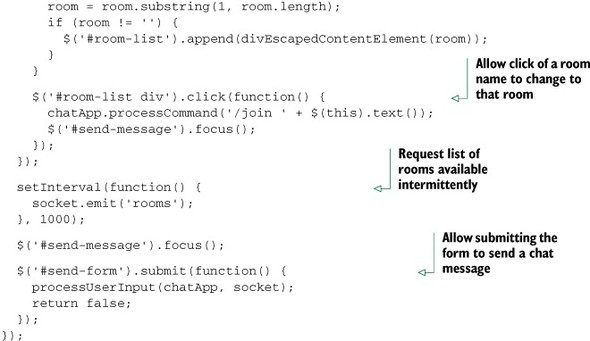
Ax hinisf kqr nacitplpoia vll, shu rdv aflni YSS ntilgys zopv nj vrq glfnooilw siitlng kr yrv plblu/ie/secetytyslhsest.aza ljfo.
Myjr vdr filna qvkz ddaed, hrt nginrnu urv lpicaantopi ( using node server.js). Ahte steusrl hsudol vfxx fjvo figure 2.14.
Take our tour and find out more about liveBook's features:
- Search - full text search of all our books
- Discussions - ask questions and interact with other readers in the discussion forum.
- Highlight, annotate, or bookmark.
You’ve now completed a small real-time web application using Node.js!
You should have a sense of how the application is constructed and what the code is like. If aspects of this example application are still unclear, don’t worry: in the following chapters we’ll go into depth on the techniques and technologies used in this example.
Before you delve into the specifics of Node development, however, you’ll want to learn how to deal with the unique challenges of asynchronous development. The next chapter will teach you essential techniques and tricks that will save you a lot of time and frustration.