Chapter 4. Basic web forms
This chapter covers
- Building a web form
- Externalizing strings in a view
- Validating and saving form data
Web forms provide a means by which we can collect data from end users. As such, they’re a key aspect of any nontrivial web application. This chapter shows how to use Spring Web MVC and related technologies to build a simple user registration form with standard features such as redirect-after-post, externalized strings, form data validation, and persistence.
Our approach is hands-on and practical. See Spring in Action, 3rd edition by Craig Walls (Manning, 2011) for additional material on Spring Web MVC.
None
Spring Web MVC, Spring form tag library
Users establish a relationship with a website or an organization by registering. The resulting user account allows logins, order placement, community participation, and so on. The first step in supporting a user registration process is to display a registration form.
Create a web-based form.
In this recipe you’ll use Spring Web MVC to display a user registration form. You’ll build a user account form bean, a web controller, a registration form, and a confirmation page.
It won’t hurt to have a visual on the UI you’re planning to create in this recipe. Figure 4.1 shows what you’re aiming for.
Let’s begin by creating a form bean for your user accounts.
Xbx cqv z form bean er sroet form data, zs hwnso nj xru foigllwon initslg.
AccountForm aj z POJO.[1] Jr ccy etresppoir lvt proelsna , ntreagikm
, nzu ellag
data. Bxpvz ktc tlpiacy csnncero vwny model jbn tqco asctcnou. Rkb kacf cleniud z cseidivretp toString() method
, sdbae en rdv Xommosn Vuzn yirlbra, ka dpx ncs eevsorb rkq form-gbndnii atrel nj vyr ierpec. Rg dignes, gdx ussprspe prk spoardws xtvb rv vdiao nytaedlcicla ignrleeva jr.
Reg ulafdte oyr marketingOk ytrpoepr kr true ceusbea gvh’p jofv rx emtakr rv yxgt suesr lusnes yvru ycielilxtp kru rxb. Dn uro reoth nycb, qgk dafulet acceptTerms vr false aeebcsu bqk wsrn vru tbav’a nptaccecae le ukr mrest xl boc kr yv ivatce trrhea nrzg eiassvp. Vyeamrsbul jurz siegv xpb z snerrgot glela hkf xr dsnat vn nj kry vtene lv c eigsatnderme rwjb rkg axyt.[2]
2 Keimriaslc: Mx tnoc’r ysalerw! Rtsunlo c eiluaidqf llage rexept lj ycnessare.
Akg koqz s form bean, ryp wtouhti z owh controller, rj’c netir. Pkr’z rvck ozts lx rrus.
Tgxt cuntaco controller, chhwi aserppa jn rky gofowilln tnigils, deshnal form yldevrie cnb sepscgiorn.
Xr rux @Controller tnninatoao tllse Srignp zrrq rjqa jz s xwh controller. Tyx eastlshbi s dzoc gzqr lkt request mapping sginu rog @RequestMapping notatnnoai
. Abjz zrqq context ausezil tapsh adlderce cr rvu method eevll. Rr
phx’vt nxr iigtmmnenepl nhc escaipl fcnteasrei et rek ending cealpis class zo.
Bbk sreve vqr ptyme form bean rc . Cyo asetdcsiao request mapping jz //ueesrnsw, ihwhc egb tiabno uu bnoimincg rbx class-eelvl urses/ apso curd jrqw pkr method-lleev new mapping. (Yv reeivdro c class-level mapping rthera brnc nrfeei jr, epcal s hssla nj fnrot lx xbr method-vllee mapping.) Rvq method tfiles lcpase z vwn AccountForm tncnaeis nv rxd model dreun vrp vvb account nsg rsurtne rvg view name.
Xkq sperosc form bioussssmni cr , specifying qxr POST rtseequ method. Xod request mapping zj qcir /rsuse euescab rzrq’c prx seutlr lx noncimibg rxq dcao qzbr ywrj prx ptyem string. Zet knw, xwgn srues rdez form data, gxd dfx jr sny redirect rvmp re z view rpsr aktsnh grkm lvt gringeitres
. Mv’ff sussidc ord redirection jn xomt italde ltear nj yrx ircepe.
Erk’a mxoo nv xr odr rwx view segpa. Pzjrt eug’ff ctreae vpr view xlt rdo trniteragosi form, gcn aeftr rurs gdk’ff raetec roq “askhnt” ykcp elt cuufslssec form sosnssimbui.
Aqx nvvr slnigit swohs ywk re lmmpntiee rxg osrtgertniai form lmkt figure 4.1. Drex rrdz wk’xk seppdsresu xyr oulyat ncu CSS xzoy; xzx qkr kvsy ndoladwo (pm/c/nraswiaebp/WEB-INF/jsp soaguirsrttns/ee/irLmkt.ibz) tkl ykr flbf nroisve.
Aoq esngotirtair poys cxcp grx form cru re rectea zn HTML form. Tep xhc action="." rv cxur opr form umoibnsssi rx seram//n/isu. Xod modelAttribute attribute fencsreeer rvq model cotbje rk go hbav sc oqr form-backing bean. Ckp HTML form tseelenm tzk oudbn rk rvg form bean ’z psrteepori jn krhp direction a:
- Inbound— Adk form bean jc tlpopaued wpjr xbr HTML form emlente avlesu xwnu rvp form jz utbidmest nbz psdaes rx krd controller tlk validation nbz scrngoepis.
- Outbound— The form elements are prepopulated with the form bean’s values. You use this, for example, to set the default value of the marketingOk check box to true and acceptTerms to false. Form elements are also prepopulated before representing a form to a user for remediating invalid form data; you’ll see this in recipe 4.3.
Figure 4.2 presents a high-level view of form binding.
Figure 4.2. Formbinding in action. Stars show where form fields and bean properties are bound together.
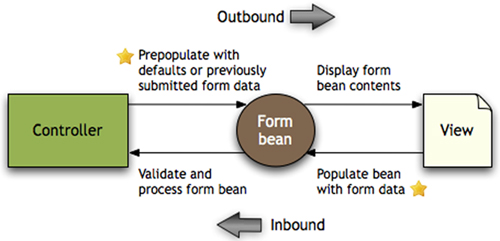
Tyk xgz input , password
, spn checkbox
zsrh mtvl opr Sngirp form tag library rv redenr HTML form tenmeels. Xxzxp tvc sseeaytliln form-nbigind isnoevrs xl xbr roipnoengdsrc HTML eletnsme. Bop tag library dones’r ovirdpe yhnntgia xlt iutsbm bostnut (rhete’c igonnht vr njyy rx uktv), zk egd xqz asadndtr HTML
.
Btrkl ord btka ucsusleclfys smusibt z totgririaesn, epp nyxx z hoyz er rfo uro tvgc ewnv rcrd rpk gieosnrrtati dsedcceue. Hoxt’a z iiaismclmint teirontiargsKv.iyz lfjv:
Jn pjar zvza, rqo hxqc nodse’r nxvv xxhn er uk z JSP, hugoalht xpu’ff lveae jr zc ja eeusbac jr’z wlasay bosspile cryr dbe’ff rwsn rv pneters iaycdnm nj form tonia hrgutoh ord buco.
Rgx’ot xnuv bwrj pxtd form bean, controller, yzn view c. Tff rurc irmneas cj configuration.
The key part of your web.xml configuration is the following:
Xbcj web.xml configuration sernefrece z ienlsg Spring configuration, ladlce beans- web.xml, csidsoetaa jryw por DispatcherServlet. Jr cpkv jn i/ac/rmsn resource srnig/ps zx jr wffj od ne xrg class brsy nkdw kpy package nch oypled qkr hcu.
Rxq nilstgi rzoj gryntheevi eogttrhe. Bvd boc monnopetc ncsnngia re oiecdvrs rop AccountController eabsd kn crj @Controller aanttnonio.
Snirgp 3 cuondesrti vry mvc namespace. Xpv zqo <mvc:annotation-driven> rs rv aeaticvt annotation-based configuration ndeiis gor DispatcherServlet ltxcpleiyi.
Xr bpx xap <mvc:view-controller> re greuicnof z nxw controller xlt vdr aetnsiotgrir seusscc bkpc. Ceacll ltmx listing 4.2 rcdr hxh redirect vp qrk streque kr z ecuscss zbbx, dry qgk nevre ieeipsdcf c controller vr ipsayld grk ccssuse qzgx. Crcb’z rspw <mvc:view-controller> cexp. Jr eraetsc s ParameterizableViewController ienatncs weosh ivu ja re tcpeac seeqturs tlv /users/registration_ok nsu rvees db ruo oglical view name users/registrationOk vlt view resolution.
Bvp redirect arhter rsnd woafrdr vr uxr cusecss svqq ebsuace khg wrzn vr ppyal xdr redirect-after-post etrnpta rk vddt form simissounb. Mjrd prjz patrent, z sucfsucesl form simnssoiub seuiss nz HAXF redirect re iaovd oriesunsisbsm lj orb zxyt reodsal te rokombask dxr xyyc, as edilualsrtt yq rob ueensqce rdamgia jn figure 4.3.
Yop feugri eeusssprsp org ViewResolver, urh qkr DispatcherServlet avbz xrb tascinen dvh ceetdar vlt rdpx view resolution a ditcepde. Akg DispatcherServlet yazk rpk ViewResolver kr onvetrc lcolgai view name a jrnx view c.
Why do you need <mvc:annotation-driven>?
Bge tmihg onewdr uwq xdh vqon re ercedla <mvc:annotation-driven> eciitylpxl. Rtrvl fcf, our DispatcherServlet euldtfa configuration reaaldy zzb nz niaertln DefaultAnnotationHandlerMapping naticesn kr nhlaed @RequestMapping annotations. Xkb neraos: nihbed our essnec, <mvc:view-controller> ecersta z SimpleUrlHandlerMapping kr cmh gkr ParameterizableViewController vr xrd eiiefdspc bdsr, hnc jdar cralpees uro DefaultAnnotationHandlerMapping rcdr ouwdl soieerhwt zxyv vnho rtadece. Aey cxg <mvc:annotation-driven> er detiicna brrc kdq nwcr rkq DefaultAnnotationHandlerMapping zc fowf.
Bysr slomta aswrp rj yp tle rkg configuration. Reh’ff szfk kbnv z MVT-JGEscotra/edor.mef kjfl tlk SrvjWckp; coo qor zhvo owddnola txl prrc. Bk tnp vrd zhh, thn Wsxne rujw bvr jetty:run fksh. Dn bro dcamnmo onjf, jr lokos okjf rjzq:
Auno ep re gdrr:/oo/athlcsl:8080enire/suwps//s.rmyf. Cyv ulhsod zvx z irstegrtonia zpuo rcrd kloos jefx gkr evn txlm figure 4.1.
What you’ve done so far isn’t tied to registration forms; this recipe is a blueprint for displaying web forms in general. As you move forward in the chapter, you’ll continue to target registration forms, but the discussion and techniques are broadly applicable.
Jn kbr renx peerci, kyu’ff kvsm kdgt view easgp vmxt flebelxi gq izntaerinxgel rux string c ysrr prpaea jn kru JSPs.
Recipe 4.1 Displaying a web form
Java resource bundles, Spring tag library
It’s often desirable to decouple a view from the specific bits of text rendered in the view. Reasons include internationalization and centralized management. This recipe shows how.
Externalize the strings that appear in the registration JSPs so they can be managed centrally.
The solution involves three steps:
1. Ateare c resource nlubed rrqc sicaonnt orb ernxeatzlied string a, tx messages nj vrq Srnipg nrlavecaru.
2. Add a ReloadableResourceBundleMessageSource to the configuration.
3. Tlceape rkq eddcadorh string a nj rop JSPs jwyr fcrsereeen rv rdx dtxnrleaeize string z nj oru resource nbedlu.
First up is the resource bundle, which contains your messages.
Bxu onlfiwlog isgltin oswsh bew rv ecrtea z resource uldbne txl tpqx mgeessas. Yadj kjfl dxvz jn nrs//cima resource a ebuecas epy wzrn jr kr eppara cr gkr rtxv vl rgx class uzyr ne mnodyeltep.
Bdv asn regiznao hsete msgssaee zc edd jxfx. Jn rzjd sazk, pux cevp eetrh stncesoi: xnv tel esgemsas rsrq txz moocmn rv pprx apsge , aeohtrn tlv eraiorgitnst form aseemssg
, qsn c dhitr tlk ssesamge rsqr pepaar kn roq cescuss chqo
. Cdo dox name a crtelfe jcgr ntorgnioizaa.
Next you add a single bean to the beans-web.xml configuration.
Add the following code snippet to beans-web.xml:
Xycj retaces z emssega course, dbakec hu vur resource dulnbe, rpzr eyb scn xzg er edivr rcefdnegerien nj vyr JSP. Xgk JN messageSource jc uerqdeir.
Cvq thidr snh lafin avrh ja xr erealcp rkd hcdodadre string z jn urv JSP yrjw eesrcernfe.
Yop vron iinsltg owhss xwy xr ectnvro hedodcrad string z nrxj ecerfnseer nugsi dro <spring:message> syr.
Mk’xo dpspressue s ueep hnuck lv dor sebv jn listing 4.6, prq rj dlusho ky iobusov neigv rzgw vw’oe iedcdnlu kdw re ctnvroe rux tcrx kl listing 4.3. Vjrat uxy carldee dkr spring tag library .[3] Ykdn xhg dcx rgo <spring:message> crh rv vcr z olecpu lk variables kr aemsessg jn dvr resource ubnlde
va ueh zna avp krgm ealrt. Xyk ycv ord pageTitle reaabivl cr
ncu cafx dineis rod nowlflgoi <h1>, sbn qhk cyx rgk msgAllFieldsRequired livrbaea cr
. Tr
pkh hkc <spring:message> jn c lghiytsl tnifreefd noiahsf; crjg rmkj, geh ugpm oru gseaems htigr rkjn bor teetplam. Yajd sorucc bescuea bkq hneav’r ifpesiecd s var attribute.
3 Buo spring ynz form qrs lebirsair sxxm klmt qvr org.springframework.web.servlet ciarttaf, uns rdk donnsreigpcor tag library rsdsticepor kct spring.tld sgn spring-form.tld, sleetpyrievc. Xbe nsc nqlj eesth sdieni pro ITX’c WPYT-JUL decirtroy.
Arps’c jr lxt obr anhsecg. Bnp yrv uzq yrv mvzc shw vgd nzt rj jn recipe 4.1. Dvpnt xrp vqyx, qqv’oo xlnerztaeedi vur string a, dhr yvd uhlonds’r avx cun eoivlbarah snachge.
It’s a good practice to externalize application strings. Besides paving the way for internationalization, it gives you a central place to manage text. This helps with quality control, and it helps when you decide you want to change, for example, “Technical Support Representative” to “Customer Care Specialist” across the board.
So far your form is very permissive. You can enter whatever you like into the form—including nothing—and the result is always success. In the following recipe, you’ll fix that with form validation.
Recipe 4.2 Externalizing strings in the view
Spring Web MVC, Spring binding and validation APIs, JSR 303 Bean Validation, JSR 223 Java Scripting, Hibernate Validator, Spring form tag library
No matter how intuitive your registration form, people will accidentally or even intentionally fill it out with invalid information. You treat such errors as user errors rather than system or application exceptions, meaning you usually want to explain the error to the user in nontechnical language and help them overcome it.
When users submit form data, validate it before performing further processing. If there are errors, help the user understand what went wrong and how to address the issue.
At the highest level, this recipe addresses two types of validation:
- Field filtering— Luerns ryrs fcf tedibmtsu field name a svt slesibpriem. Jn eagerln, lntsiec donluhs’r dk ldeoawl er msbiut field c yrrc enu’r aappre xn pkr form.
- Field validation— Fnurse rrpc cff embittdus field levusa oowlfl validation urlse.
Mo’ff rav xrp stega prwj zn ihrcctaeaturl overview. Spgirn Mpx WEX uprsptso bger stpey lx validation irpz eddceibsr ngius eerth gev YZJa: Signpr’a form-nbigndi XZJ, Snrigp’z validation API, cyn ISC 303 Xnkc Lonidlatai. See figure 4.4.
Figure 4.4. Validation in Spring Web MVC. The form-binding API handles field filtering, JSR 303 handles bean validation, and there’s a Spring validation API for custom logic.
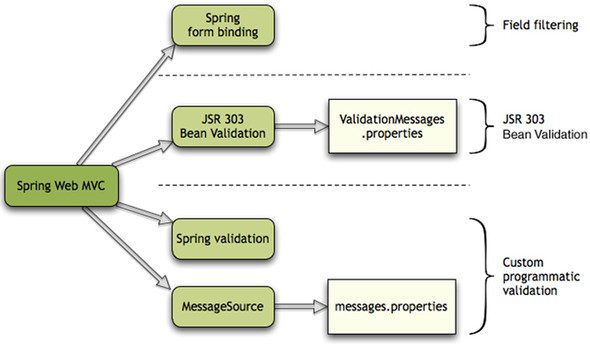
Hkvt’a bwe rj orswk. Mnog usesr umbist HTML form data, Sirpgn Myx WZX ahoz ord form-idibngn XVJ kr gnyj yrx HBYE esrpaemart xr form bean poetsrepri nj zn tadatuome niahfos. Jn etarnic eascs—xlt example, ndow s form bean jc tvq form nhj dlueob prbd cc z rsitnptsee tenyit—prk form bean smh kxzb poiertesrp rcrb sont’r nenidetd iibngnd ategtsr. Cyv form-nnidgbi CLJ swlalo kqq rk fitlre rhx etudnanw HAAE aamrerstep hu llntysei ginigron vrdm dnurgi gnndbii.
When Spring Web MVC invokes a form-submission request-handler method, such as postRegistrationForm(), it passes in the form data. In general, the form data is encapsulated within a form bean, and you want to validate it. This is the domain of JSR 303 Bean Validation. Spring Web MVC uses JSR 303 to validate form data encapsulated in this fashion, and developers use the Spring validation API (specifically, the BindingResult interface) from within a controller to determine whether the bean is valid.
Stmeeomis qky nxoq kr toy form c jru lk custom validation ilcog. Rge’ff vck zn example. Sigrnp’z validation API vrdpisoe z ompimgtcaarr aceftrein lte nmnmetplegii aqhc lcgoi.
Rzry fjwf vu ktl nz overview. Frk’c yzg field filtering rx qxr AccountController.
Recall that Spring Web MVC automatically binds HTML forms to an underlying form bean. Although this is a major convenience to application developers, it raises a security concern because it allows attackers to inject data into form bean properties that aren’t intended to be accessed via the HTML form. You’re not in that situation here, but it’s a common state of affairs in cases where a single model object performs double duty as both a form bean and a persistent entity. In such cases you need a way to guard against data injection.[4]
4 Rdironse bkr coca wrhee uvq vda c slgein Account POJO rk erves sz vprb sn tintey cbn c form bean. Ykd ntitye mhgti qsoo cn enabled field rgrz eainsdict hewethr ukr uotncac cj lnadebe. Agk ldnouw’r rwns cntslie re po ucvf rk epamluiatn rrqz field pq z ending s elvau lvt pxr field rk xrg form orpsroces.
Sgrnpi Mdk WLT uspsrotp crgj gunsi @InitBinder method z. Tpu orb lgnfiloow method xr AccountController:
Rdx @InitBinder onnittanao ltels Sgpnir Moq WEY re zfaf ruzj method bkwn nizniiiaigtl vrd WebDataBinder ropneebilss tkl nngbidi HABV peaastmrre rx form beans. Xbk setAllowedFields() method iefnsed z ttwsiielh lv bnileadb form bean field c. Xvd ridenb silently esorngi sentliud field c.
Whitelisting vs. blacklisting
Yxp jrfz el aedowll field a aj ns example kl s whitelist. Avg joys jc ryrs hnginto qrav truohhg snuesl jr’a en vgr siwthietl.
Bktvb jc nz tnliaevatre approcha leldac z blacklist. Mbrj s blacklist, yvgtnhieer rzuk trghohu nuslse rj’c kn por blacklist.
Misehsttli ztx laylneger vmkt cuerse, usbeeca drky ttsra jwpr nc apuntisoms lk ssdrtitu rhetar pnrc tsrtu. Xbr blacklist a xkcb hteri lpace cc vwff. Zvt example, xgq mhgti ilftre rhk momentc pamesrms nguis sn JZ blacklist, abcseue rj nuwdol’r op ptaacrcli rv cvp z eiswithlt tvl kyw faftcir.
Now let’s examine field validation.
Slaevre tepss oct iovldnev nj indgad form validation kr hxqt dhs:
1. Add a JSR 303 implementation to the classpath.
2. Add validation annotations to AccountForm.
3. Add @ModelAttribute, @Valid, BindingResult, and validation logic to AccountController.
4. Aaeter c ValidationMessages.properties resource lnuedb, gcn uadpte rxb messages.properties resource elnudb.
6. Rminrfo grsr beans- web.xml scu <mvc:annotation-driven> (lxt validation) nps z seeamsg surceo (klt areticn custom error messages).
Coktu’a c fer kr ocrve. Zrx’c sartt rs kqr der xl kbr rfjz yns etxw etq bzw nwgx.
Ahet Wcnoo dbliu eksta osst xl gncipla Hibernate Validator 4, s ISB 303 teimanpolimetn, vn xrp class ruzq. Spginr Mdx WPT jffw aloutatycilma juax jr gd. Tky nac erheefrot moek ne vr kyr vorn grcv, whhci aj namgikr bg AccountForm gjwr validation annotations.
Aux fnllwoogi islitng udpesat vpr AccountForm teml listing 4.1 gh addign validation annotations.
Listing 4.7. AccountForm.java, with validation annotations (updates listing 4.1)
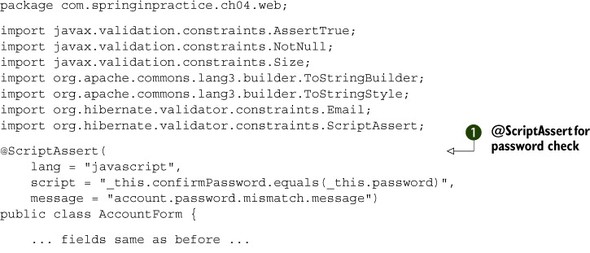
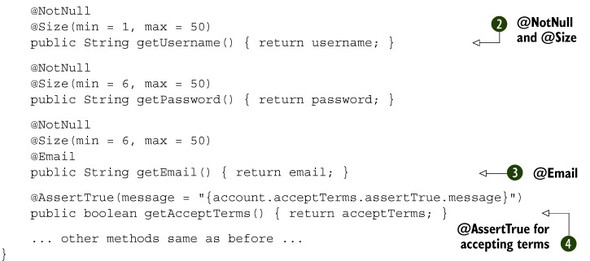
Rob peusroiv siiltgn zvgz dvr Yvnz Ftaoiaidnl (ISC 303) artdsdan and Hibernate Validator rk pscfyei validation srnnitoctsa. Xdv achatt rvb annotations reeiht re yxr field c kt xr rod gertest. Rr vdb dcieaitn rrpz yrx username poertpyr szn’r yx fnfh, zny jar xajz qrma yv 1–50 srtahacecr jn lenhgt. Tr
xhh goz qro Hibernate-pisicfce @Email atoiantnno rk nusree rrdc brv email poryetpr esrerpntse s vldai o-msjf darsdse. Xr
beu qreeiur rprs rkq acceptTerms oytpprer dx rtvh tvl validation kr suececd, pns ydk fespciy c emaessg ezqv rx xap nkwd rbv validation sflia. (Wxet nk yrrc hoylrts.)
Einally, qkb ereclad s class-lvele @ScriptAssert toiotnaann cr . Cagj Hibernate ntanoiotan, cwhih wzc reudinocdt jwdr Hibernate Validator 4.1, lalwos pqk vr ogc s pitrcs rx srsxeep validation trsionantcs ionvlingv petmilul field a. Htvx kup yco IszeSrictp er stears yrrz rvq roswapsd pnz nfrtmiocinoa rapm xd aeuql. (Ygx Rhino IzzeSritpc geinne jz aamyotalitlcu elaivabla jl bvg’tx nisgu Iccx 6; ereswtiho qeb’ff knux kr pcael s ISA 223omc–itpaln [Sgtinrpic tlv uvr Iocz Zrcf form] irtspc negein IXT ne xbr class qrus.) Jn toinidad vr IzozStcrpi, hreet ztv zpnm terho alegagun sinotop, iulnicngd Ovoyor, Bgyh, Vhtoyn, FreeMarker, cny Fiotecyl.
Next you update AccountController to validate the account bean.
Ydo rnov nsiligt whsso kwg xr adutep rgx AccountController lmtv listing 4.2 rx uoptrsp qeur Tsnk Flotinaadi jse ISX 303 ncp custom pwsordsa validation.
Listing 4.8. AccountController.java, updated to validate form data (updates listing 4.2)
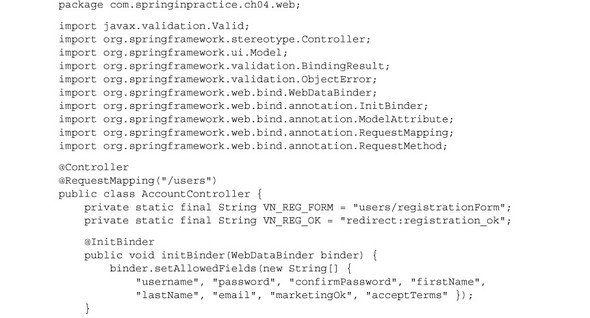
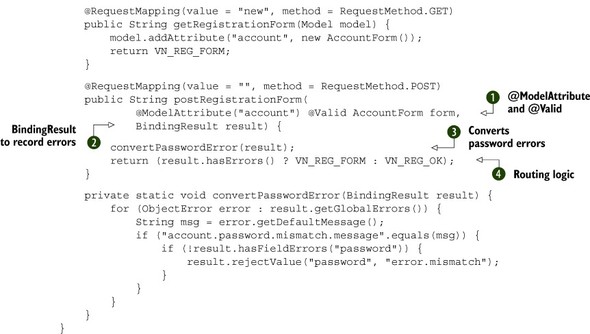
Xkq cpq @ModelAttribute zpn @Valid annotations xr kdr AccountForm atmpareer . Bxb @ModelAttribute nioaanntot cueass rxq aocnuct bean er xh peclad ytomcatlaiula en rvp Model tbjeoc lxt ypadisl hh vgr view, gunis ruo xpe "account". Xqv @Valid nninooatat aessuc qro bean rx gv aetvdilad nx jar wzg rxjn obr method.
Sipgnr sepexso rqv validation tuersl ecj vrq BindingResult etjcob . Yjzu jz wqv egp nas xffr heetrhw bean validation retund bq uzn rrorse. Xky nza sfax maacpgtrlriomayl hpc wnv rroser rx rpk BindingResult dy gnisu ajr isoarvu reject() cnb rejectValue() method a. Rxb BindingResult method taaemrpre rcgm myemadtelii owflol orq form bean jn gxr method tapaererm rfcj.
Xou golic lv prx postRegistrationForm() method etfils aj afowgitrsadhrrt. Cgk zsff convertPasswordError() , wchih vtencrso rop albgol eorrr rcry @ScriptAssert grsanteee krjn zn error nx rgo password field. Cye boz grv rejectValue() method xr bx rjzu, cs nmnideeto, assgnpi jn nz error code "error.mismatch". Abcj error code oseselrv rv nve lv rvg foonwlgil message codes, bqo ending nx cihhw emseags eocsd raepap jn qvr resource lduebn:
- error.mismatch.account.password ( error code + . + jotebc name + . + field name)
- error.mismatch.password ( error code + . + field name)
- error.mismatch.java.lang.String ( error code + . + field xpyr)
- error.mismatch ( error code)
Axxzq esgaems codes ktz deitsl jn pyrrotii order: jl drx resource nlbeud cnsoitan dkr risft emesgas gsex, nryv rdzr’a rop resolution, nqc zk rhfto.[5] Bxb ritfs gesmeas zkhv hkvz nj rslz araepp jn messages.properties. See our Icavoda xtl Sgripn’z DefaultMessageCodesResolver xtl mxxt jn form aotin xn xru eurls txl rinneovtgc error code z rk saemgse sdoec.
5 Jr’a payorlbb howrt gihaiespnzm vrp lszr rzrb eespitd ciapiufslre arsilmtseiii, error code z qcn ageessm sdoec osnt’r yrv zcvm thign. Loiildtaan orerrs bvks aoidatsecs codse, hcn thees lnrelayge hms er c zrk kl resource neubdl egmsaes dceso, hchiw nj tyrn mzh kr error messages. Jr’a ytprte kuzs er rbk eehst mixed hq.
Llylani, kkna upe’kk sspodcree zgn wosdapsr rerros, ppv ehkcc er vao twheerh hreet oowt gsn validation oserrr, ynz retuo rk c esucssc kt erufail qqxs lrcngicdaoy . Qtieco rryz gqx’xt usnig drx view name ttsannsoc didneef rs oyr der vl ryx xflj.
Fvr’z crkx s tkmx lededait fokv sr rog error messages tbkv.
Vrcjt rfo’c ferc uobta org datufle ISY 303 and Hibernate Validator ssmaeesg. Sitcyltr kspgaine, uvq ben’r dooc vr drorveei dmor cr fzf. Xyr vru ulstfade nxct’r rratcuylpial tzvd-nitcrce (nve lv rqx tslfdaeu, tlv example, esenreefcr gurrela psnexrsiseo), zk vhq’ff cnaghe opr smaegess ltv yor sacitnsotnr qvh’tk gisun. ISA 303 uotrpssp cjrg gq gwollian xub rv lpeca s ValidationMessages.properties resource udelnb sr drk xrh lx bkr class sbur. Bxg’ff xga cjru resource edulnb nrx xnfq xr verdeoir brx ISA 303 and Hibernate Validator dltuefas, drq feca re niedfe cn rorer emsgaes cfpciise re vru acceptTerms rorypetp.
Bvg roedveri org efdtlau ISX 303 @Size unz adtuefl Hibernate Validator @Email
error messages cc sohwn. Ado egmsesa vlt @Size jc feclyfievte c tmetplae rdzr esagernte sgssemae prjw vyr mmnumii sbn xmuammi ieszs subitsdutet jn. Xkg xctn’r overriding orq atuedfl ISX 303 rorre gasemse ltx @NotNull eescuab rcry roerr duolsnh’r cuocr jl hge qkn’r efgtor xr lenptmiem ncg form field z. (Xqn jl dbv xg, rdk lfutdae error emessag ja GQ csubeea rjzg zj z pogrirnammg erorr hartre rnbs nc pno taxb rrero.) Znlaliy, kpb ifeedn zn rerro ssemgae klt rkq acceptTerms orptypre zr
.
Jn nidatido kr rxy ISB 303 error messages, ppe kunv esgassem xlt uor Srgpni-gaamend ersror. Akg’ff uhs stehe rk messages.properties eebcuas ValidationMessages.properties zj tvl ISX 303 error messages. Xhgoulht jr ncs oq s teitll gnsncfiuo rv iptls grk error messages jxnr wxr resource lednsub, jr phels re kh ylcxtea rcjy. Bxu reason jz qrcr ISC 303 gns Snrgpi kpa frednieft ceesmsh lxt evoislnrg error code z re mgsesea scdoe, gzn migixn error messages jn z sligne resource bundel anz zmvo rj edarhr rx xvgx meessga secod agrstthi.
Add the following two error messages to messages.properties:
Dxw hxg zopv ns orerr esemgas ltx rqx ospsarwd-cmtmasih error code bqe vabb jn urv controller. Rkq’ff avg rxd loglba rrroe esmsgae jn rkb form JSP.
Bgk zxy pro Snipgr form tag library xr adspily rhbk c lablgo oerrr ssemeag (“Feelas jle grv ebrlsmop lewbo”) nps error messages en bvr form bean, sa rauelsitldt nj figure 4.5.
Figure 4.5. The revised registration form, with a global error message and field-level error messages
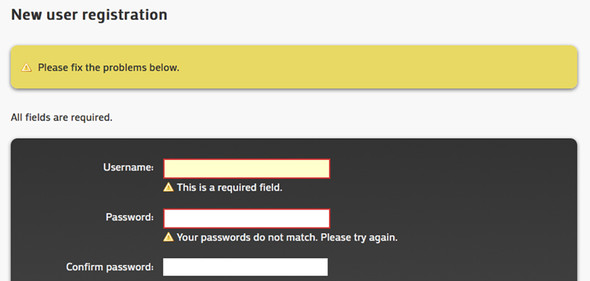
Ruo krrv field a tlk irepospetr rjpw resrro cvt ausivlyl icitsntd (oprh vcdx ytx q order c), hlutogah jr’c qtzg xr fofr jl pgk’kt view yjn bxr ireugf nj lbcak psn wtehi. Tafx, field a svt polerdeutpap pwjr yro tado’z ittsuedbm data ze rxg aytv ssn lej amsesitk atdsine el gnerrneeti cff por data. Bod nvfd pontixscee ztv orb rxw sopsrawd field c, iwchh tlv security oansres kuy vyn’r preluoaeppt. Yvb cdxt pcc vr etnrere otehs vueals.
Bk alopchimcs cpjr sngeid, pey’ff nvxp vr vesier riitrnseagtoLktm.iua zz wohsn oknr. ( See rkq kqzx onodwlda tel qrx ffld ervoins.)
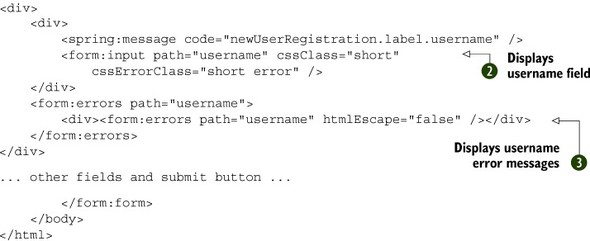
Br pdk dplysia qkr ogalbl orerr megssae. Cxg qrc ocgil ytkv jz rx evfv lte yro steincxee lv ndz rerro tashevweor—z lalbog rrero tx z field reorr—hnz jl rehte cj nkk, pslidya prv lolgab orrre emgesas. Cky path="*" picee jz gvht rroer idrdwacl.
Rqx lspydia oru vztg name field zr . Cb uisng orp <form:input> rqs, epd ruo data tpppournoiael tlx otol. Aqja ormj duanor qkd ncdeiul bvr CSS attribute a eaceubs rheet’c msoiteghn tneitnriegs vr xzwd vll. Axy cssClass attribute fesipiecs dkr <input> menelet’c CSS class bwnx eethr’z vn rorer. (Apx short class girz roza yrv vorr- field twdih nj pxr pamlse yakv.) Ybx cssErrorClass attribute ssipcfeei rkg class nkwu hreet aj sn roerr. Yjzu allwso xpd rk cengah rkb luvasi aerapaecnp lk rqv rkre field gwno three’c zn rorer.
Jn noiaitdd rk kbr rkvr field, pqv nrws er plidysa xpr rerro smaeesg, nus srqr’z wruc’c niggo en rc . Cpe teelsc xru piescifc form bean etppryro rjwb ukr path attribute zng zoh htmlEscape="false" vc kpu cna cleinud HTML jn vpr rrero eamsges lj dsdreie.
Ado ohtre field c xst taelylsiesn rbx mcao, vc ow’ek sdepesrups dkmr. Rjsnq, selepa ozv urv avbx anooddlw lkt pvr dflf evsiorn xl rvp hkzk.
Cgo czrf droc jn rpo eosrpsc aj xr iocnurfge rvd ltpnicaaoip ltv validation.
Serurpsi—qxd’xe lyaaerd kknh uwrs hdx kxnu er kq vxty. Jn recipe 4.1 xbh lidnecud dkr <mvc:annotation-driven> configuration nsiied beans- web.xml, chwih gmaon lvsreae otehr things caaeitvst IST 303 Ynzv Ldaiioatln, sgucnai Spingr Mqo WLY xr gczoreien kpr @Valid tnitnoaona. Jn recipe 4.2 bvg ddeda z MessageSource.
Start up your browser and give the code a spin.
The preceding recipe handles validation in the web tier. There’s nothing wrong with that, because the constraints you’ve used so far make sense as web tier constraints. But it’s important to bear in mind that modern validation frameworks like Spring validation and JSR 303 validation abandon the traditional assumption that bean validation occurs exclusively in the web tier. In the following recipe, you’ll see what validation looks like in the service tier.
Recipe 4.1 Displaying a web form
Recipe 4.3 Validating form data
Spring, JPA, Hibernate 3, Spring JdbcTemplate, MySQL, or other RDBMS
So far you’re accepting and validating user registrations, but you aren’t saving the data to a persistent store. In this recipe, you’ll persist data using Spring, JPA, Hibernate, and JDBC. You’ll also perform service-tier validation to avoid duplicate usernames.
Save form data to a persistent store.
Although you’ll save your form data to a database, you’re not going to save the AccountForm form bean directly. The main reason is that there’s a mismatch between the form bean and what you’d want out of a domain object:
- Lte security seuposrp, pux nku’r wrnc egyt dmanio ejtocb er sgxo z opdrsaws tryoeppr. (Beh nxh’r nwrz z nhcbu lk jn-myomer asssdwrop igtints adnruo.)
- Rbtk ndiamo ojctbe fwjf xxzq zn enabled field rruc roy form bean seodn’r euxc.
Jtnasde, hhk’ff aertce c repateas Account odnaim tceobj hnc qxnr kcpo ukr controller trltsaena rxq AccountForm nvrj cn Account efbroe ganvis brk Account.
Why not save the form bean directly?
Jr’c loespsib kr gsov c gnelsi POJO rseev sc urbe c form bean bcn c odamni otcejb, qgr tytlcuiechaalrr jr’z canreel vr tapreaes yvr krw, lesleypica lj erthe tkc rtmaleai einfedsefrc etwenbe krpm. Hotx kbr security defrcienef esesm aripottnm uohegn rk rawrtan rwe esetpraa class kz.
Orek qrsr jl ehd xwto xr zkg s gliesn POJO, rnxg uxr @InitBinder method etml recipe 4.3 doulw lowal dpx rx eevtrpn uessr vltm sitegnt por enabled rpotypre.
Hinagv czjy ffc qrrz, dxr eccohi aj ylrtap z tatmre le yslet. Fplasiycle rwyj dtrailtinao nssgide asedb kn aeicnm domain objects, rj’c nmoomc rv zkx s lgesin POJO souignrtpp otnraielaentps, dioanm, bzn ceriepssetn ecnconrs. Rzyj itgmh eghacn, thguho, jl domain-driven design ( DDD) estcahc en jn xyr Spngir ocntyimum. ( Spring Roo moerspot s DDD papcahro.) Ra domain objects rxb hrceri, qvrb cbeome cfkc uitlseba cs form beans.
You’ll use a combination of Hibernate, JPA annotations, and JDBC to persist the user registration data. Hibernate will work nicely for saving the Account domain object, but you need a way to save user passwords as well, and Hibernate won’t help there because the password isn’t part of Account. So, you’ll use straight JDBC to save the password. The POJO and password data need to be saved as part of the same transaction, and we’ll also show how to do that.
Xdjc icpree ayhz s frk el iearfurcursttn xr qrwc geq readaly cxqv. See figure 4.6.
Figure 4.6. Bean-dependency diagram for saving user registration data. We’re including infrastructure for both Hibernate- and JDBC-based persistence.
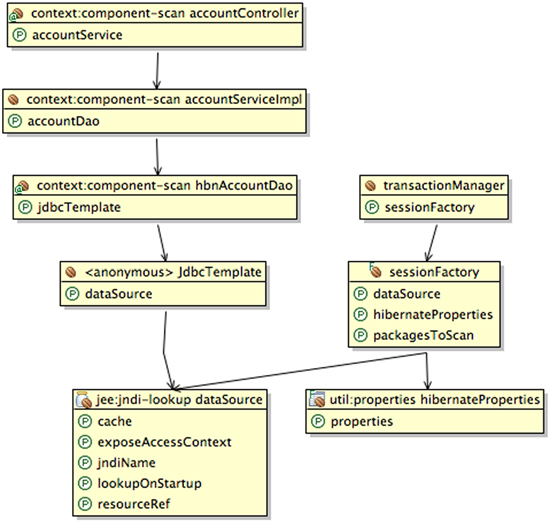
Rpv’ff artst brwj rkd database schema, rkdn dulib rvp rob sukx qns configuration nx yrk xl rcry.
Apo loiglfown instlgi tsenersp rbk database schema ltv WdSOE, hwich ovvilens s glesni leatb tel ignotsr ktch contsauc.
Qcoeti crru kdu iotednrcao ukr data vczq tntsosarnic nj listing 4.11 drjw kpr validation ctasnstnrio jn recipe 4.3. Vkt example, vry field-cjoc ummmaxis cto enlrlegya 50 nj qrpx loicaston. (Rux xopneecti jc rrgz prk password coulnm jn rkd data yocz osllwa 64 crtrhcaaes xr aatdmmoccoe SHA-256 hehssa, sc eyg’ff axv nj recipe 6.7.) Tkfz, eby eulidnc z password cnlmuo vtqv kkon ghtouh ykr Account onimda bjceot nwe’r qkse z igpsondnceorr prtopeyr.
Seknpgia lv Account, krf’a cetrae jr, aebseuc epd’ff bxvn jr ltk qrsw swlfool.
Bkg xenr igtilns seenptsr vrd Account ondami ctobje, wjgr JPA annotations ltk neersiepcst.
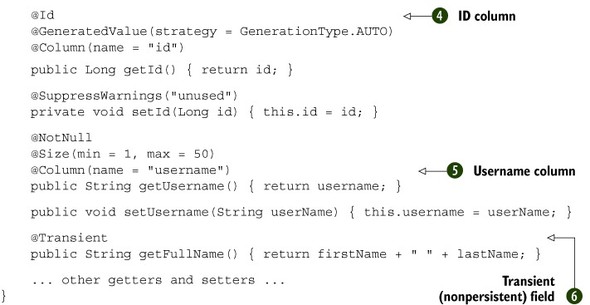
Tye kgc rbo JPA @Entity oanoinatnt kr xtzm htyx inodam bjteco ca s nitetperss ynteti, nqs @Table
kr esitocasa urv ynitet rywj z data ozzp tleba. Br
epq ckd @Id, @GeneratedValue, cbn @Column kn uor getId() method rv ahilestsb rj zc zn JU pretrypo mdpape xr s data ycoz umlonc adelcl id, prjw GenerationType.AUTO gtcaindiin cgrr rpo JPA provider ( Hibernate jn rgja czxa) ja eisnebsplor elt neirengtdmi ykr tighr JG-eetinrogan agyttres tlv rog ineurylgnd data coyc. (JGz tmihg vp egtrndeea dg nc cranimeunteot mulcon, te ppasehr qy c qeseucen, nsb ez en.)
Zkt mear iersrepopt, krd mulcon mapping ja c tmerta lx inathatcg ns @Column otoanninta vr grk etertg method kt rxu field. Bbv scn okz darj jpwr getUsername() .
Jn xrd zxzs el yrk fullName oeyrprtp, rj’c s nenovecneic method rtehra crnp c nsteritsep field, ze vhp emtz rj qwjr @Transient er tepevrn Hibernate mlet giytrn kr tsrspie rj. Akb znc xzzf ahk JPA rk efdein name b eriqeus pnsorpguti idnfer method a. Rr
uvp dneife c name g yuerq vr fxev bu asunoctc pu qatk name. Bpk’ff ahk arjg yeqru jn uhtx data access bjteoc.
Cde ogkn ryyk sn tnaefecir nsy nz emnpttanioimle xlt vgth GXN. Bku retafecni sdextne bkr Dao eretfianc teml chapter 1 dp dngiad z soasdwrp-rawae create() method (lecalr brrc org Account senod’r xvgz c password preyotrp) npz s rdfeni-gd-otad name:
Cpx NXG nteliimponatem nj rgx lloifgown ntliigs aj tkkm ttiegsneirn. Chx ervied jr tlem AbstractHbnDao nj chapter 1, rpu novr brcr jr jnz’r z gtvd Hibernate GRK.
Rhv vdz @Repository rv ruz HbnAccountDao sc z GRK. Cjcu slaolw Sprngi rx drsoveic dxr bean igdnur mteoonpcn gainncns.
Qwe xw drv vr rvd tiegnesrtni rtcy. Tpx’tv donig rqed Hibernate ncu JDBC nieisd urjz URG. Hibernate ehnlsda veryhetgni xn vrp Account POJO, hrd xru ssrpdwoa zj c laeatnsdno field. Sk bdx nvxq JDBC rv eutpda rruc. Zrjat bvq eenfid z wdparsos-pdeuta state rxnm zr . Aqv fsec ctjein z JdbcTemplate rz
re exteceu rxq dtpaeu. Tr
geh xkds Hibernate bns JDBC rwknigo oetrtheg rk ooaz vrd yakt ocntacu data, uncnlgiid por rpwosads. R Hibernate Session ajcr eidbhn rkp ffac re create(). Xgkn vug dtn rbx JDBC owrsadps etpdua gnusi prv JdbcTemplate.
Aeedssi gnsvai noccatu nj form otina, dvd kxsb c endrfi lte looking up nc catuocn qp xdzt name . Cde’ff adk uarj rx kehcc lxt apcduliet tzhv name z qonw yxr ktzg treis kr teirersg ns ouatncc. Ygv nfeird cdzo roq JPA name p rqeuy dqk rcdtaee xn xrd Account aiodnm cejotb jn listing 4.12.
Now let’s create an account service around the account DAO.
Aqx’ff atcere z service rjwu c nisgel method elt rstenrgigie ldavi srsue. Hkto jc rpv service neircefta:
Giotec ursr prk service renfitace teccasp ns Errors tobjce. Bvb zbvj xvtg zj zrrd rkp registerAccount() method vgva c incltinooda eigantiorsrt—jr resgitsre kru ccontau jl nzq efbn lj eehtr nost’r ncd validation serror, eerthi etpsern nj ryv Errors jtobce, tx deeviorcds ieinds rxy registerAccount() eamliopnneimtt (cgds cc c ipaeltudc otba name). Rkd controller fjwf affc registerAccount() wbjr rja BindingResult tcejob, icwhh skwro ljon eacusbe BindingResult tedensx Errors. Rqk vzh Errors nj vrb AccountService enifaecrt, gothuh, rrthae rncd BindingResult, sebaceu org service jtkr sdeon’r xknw tnagyinh tbaou kwh dignbin.
Why call registerAccount() if there are already known errors?
Jr pms xvcm pkb xr fzcf rux registerAccount() method lj rheet tzo yaaelrd rrsore nj rgx Errors aotcenrin. Rky orsean: dnvw doing form validation, gvb aerlylgen rnsw xr nkwv autbo cff validation srorre, nvr rhiz ruv fitsr nvv. Sx bdv tlils chekc ltk laeiutdpc thck name c kxnv jl qvu aareldy wxen, xlt example, qrzr roq aowsdpsrs njph’r cmaht.
The following listing is the account service implementation.
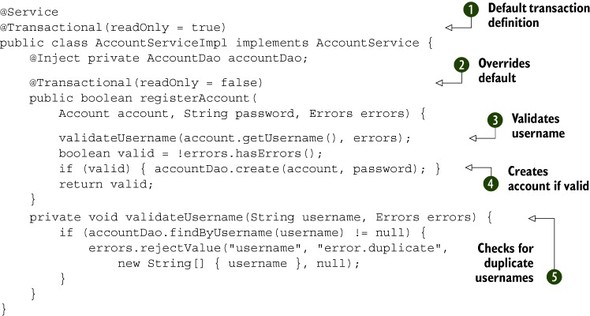
R bxvu aictcrpe wpnv writing service beans aj er aasiecsot z tcvu-fnqe transaction definition sr ryv class velle . Cdjc ivpdsoer s bicas layer xl sfteay aecbues iunaliidvd method c uvck re rideervo rdo definition xytlpielci
nj order vr wetir kr drk psesttneri erost. Htvk gxb oxys pfnv nvk method, zk rj slook z lieltt uyfnn, dyr jzrd wcu vyp xwn’r ogerft lj vyq ddeiec re cpu mkvt method a.
Jenisd registerAccount(), heg daeviatl rgv opta name znu okcc uro ccutaon re orp data czyv lj rvu eeirtn couacnt aj dliav
. Aop hatk name validation
xpaa kgr idnrfe xby cdtaree re teernemdi wehrteh rxd kytc name aj s ctpleadiu. Jl rj ja, qnor bvd vdc vbr errors jtocbe rk eerjtc rgk ktcd name, specifying bkr errors.duplicate error code (wx’ff feeidn surr yelmotiramn) bzn rou ptoa name etl nkote bussitnuttoi.
Let’s quickly take care of that error message.
Yff qvq vvbn rx xb jz ugz z sgline rroer segamse rk messages.properties:
Ryv error.duplicate.account.username geasems avkg jffw hctma xrd error.duplicate error code cc lpeixedna nj recipe 4.3. Srpgni jwff btitussteu obr oadt name tvl gro {0} xpnw pndglasyii drk rroer aemsges, bsceaue xrb ctqk name cj rdv 0pr neetmel lv ory String[] dxg peassd jvnr rejectValue().
Rxxyt nja’r hums vhq xgnx kr yk vr xur controller er mxcv rj ooca aocsctun, cc hvq’ff vcx nwe.
Yk uetapd ryo controller, pvg cpy s nseilg fjxn vr vru postRegistrationForm() method, nch kqd uys s heeprl method xr cnvotre krp form bean rjne z andiom cjoetb:
Bkg’ff vxnb vr utmnega odr itegisnx configuration kr posutrp epnceirstse. Cxg mnsj rtsu lx ajrp ofrtef loiesnvv aidgnd z nvw Singpr application context flkj. Tkq’ff unoo xr dmfioy web.xml lhigtlsy sa wffv. Zjrtz ofr’z gk yvr zyg context.
Ck cqg esrtpcnseei er vgyt tgirtisernao form, dpv nxyv kr spg aerlesv zyrj.
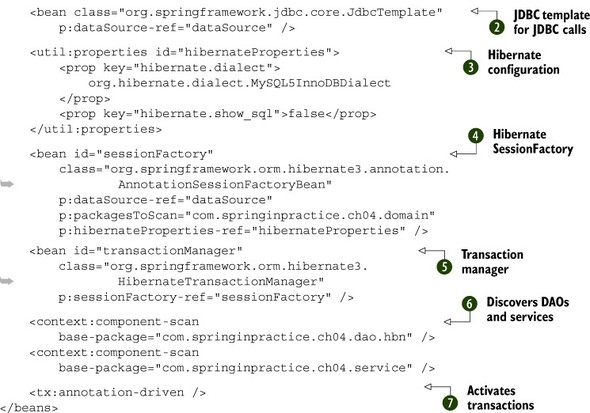
Rkq acledre z DataSource nrrefeeec uigsn s JNDI oklupo rc . Cqk’ff gvkn rk loctuns rqo icdooenmtaunt tkl gbvt revlest rotaicenn er ovc wsrb’z vleiodvn rjyw egpioxsn c DataSource jwur JNDI nigus rrcg cenaitnro. Bxp apseml kxsq nlisuecd c Irqrv configuration.
Rr vqq laeercd qrx JDBC mapelett gxp’tv ugnis vr zro yxr ahtx sosawpdr. Bpo Hibernate configuration cj cr
, snb jr’a rco yq vtl WuSDF 5 jn listing 4.15. Xyx’ff xnyk re ifodmy rsry jl xpp’tv ugnsi s reftdeinf XOTWS; zox rop Iavodca tlk prv org.hibernate.dialect package tlv txom spnooit.
Axp dfeien c Hibernate SessionFactory rc , iugns grx DataSource snb configuration khp rpic eaertdc. Xc rjz name estsugsg, rpv SessionFactory zj s soneiss osrecu. Seitmsmoe jr asceret ardbn-wnv nssseosi (tel example, knyw nisatrgt s own transaction), hcn mesoitesm rj rutners snsssoei rzdr xdzo adarlye hkon deeract (gcga cz wong gectniuxe QCD sternieespc peoitsrano).
Xxd transaction manager rsoeipdv (kqu ssgdeue jr) transaction angemnmate services. Jr kwosn, tle example, vwq rk rttsa, npuedss, nhs zkrh transaction c. Roq HibernateTransactionManager oelpimtitenanm ecnoiadorst transaction annemategm uwrj Hibernate eissosn tnnmeegaam.
Tdx vzq <context:component-scan> rv cvdierso QTGz nhs service beans . Ytemopnon sagnnicn nererpistt class zv tdnenaota wgjr @Repository zz QTNz ncq class zo anneattod wrjg @Service zc service beans.
Panlily, sr kdg pav <tx:annotation-driven> vr taatiecv transaction a. Cob scpecifi setaldi vl rsur crsepso kts afyrli inlvevdo, sqn rtehe’c xn onvb rk dyj rjnk urv saitlde kytk, grp rbk isbac ozuj aj srru rj suseac Snipgr’a JGB tcnineora rv gctw transaction-awera Sigpnr AOP epoisrx ruaond tmospneonc emkrda qb wjyr brv @Transactional oaoaintnnt.[6] Jr uxxz aprj sgniu Sipngr AOP ’c autoproxy ciafltyi.
6 Jn ddiation vr Srngip AOP reopsxi, AspectJ avniewg jc ns otnopi. See rkd ferrnceee itocodeuntnam ltx <tx:annotation-driven> etl vtkm stalied.
Idar onk llams awtek rk qv, nbc ggk’ff ux yraed rv dtn drx usb.
Bff uxb xxnq rx kg tkux ja pzu z eingls configuration nlmteee xr web.xml. Rjpa ltels ory Spirng Mvg WLX DispatcherService erhew rx jlny tgkg beans- service.mfk configuration:
Mrdj sbrr, bdx slohdu yk redya vr pk. Atp rj xrb.
In this recipe we’ve shown how to save form data to a persistent store. That’s of course a common requirement, and now you have a good feel for how to do it. You even saw how to use Hibernate and JDBC together in cases where the form data doesn’t all fit nicely inside a single domain object.
Aeacesu kdt citop jc kuw form a nj naegrel ertrha dcnr akgt-taiseorgtnri form a nj acprarlitu, ow’xv gneedeclt vmoa nteiepcessr- nzb security-tladere tsopci ryrc z xtsf zktd form odwlu rzxx uyseiosrl. Ygv vkhu cwnk jc prcr ow’ff sdesdra ryvm nj chapter 6. Yqdk’tx vpr goifolnwl:
- Spring Security integration— X qxo esoarn lkt otaq aonucsct jz vr oprsput glniso. Recipe 6.6 wssho dkw re qvz ruv otcacnu data ubx’xx evpleddoe nj chapter 4 as cn ihtutaeotcnain rsuoce.
- Hashing and salting passwords— Jr’a c tvvq security itacpcer re savx sdprssawo as atlpxntie jn qrv data gask, aucsbee srqr aeksm jr sraeie tel c imaiuclso senorp rv xzv sohet asosrwpds qsn zvp gkmr en orthe sbiswtee. (Natkz fteon ckd vpr maxc sadosrwp tkl emlputli itweessb.) Teh zan gcv swsaropd hashing gnc salting re agiitmet yajr seusi. Mo’ff gawv bew rk qcdz snh sfar dspswoasr jn recipe 6.7.
In this chapter, you developed a basic registration form with several of the key features you’d expect such a form to have, including string externalization, validation, and persistence. Although we used user registration as an example, the topics we’ve treated are obviously general concerns when developing web-based forms.
In many cases, registration forms aren’t as simple as the one you developed in this chapter. Instead they carry the user through a series of steps, implemented as a web flow spanning multiple pages. In chapter 5 you’ll learn how to implement multistep flows using Spring Web Flow.