concept ActivatedRoute in category angular
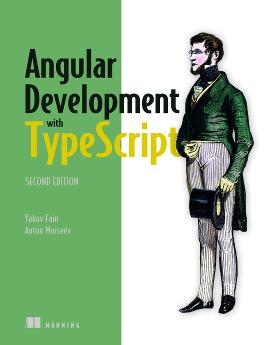
This is an excerpt from Manning's book Angular Development with Typescript, Second Edition.
Let’s get familiar with the main concepts of implementing client-side navigation using the Angular router. Routes are configured using the RouterModule. If your application needs routing, make sure your package.json file includes the dependency @angular/router. Angular includes many classes supporting navigation—for example, Router, Route, Routes, ActivatedRoute, and others. You configure routes in an array of objects of type Route, as in the following listing. Each of the elements in this array is an object of type Route.
If a parent component can pass a parameter to the route, the component that represents the destination route should be able to receive it. Instruct Angular to inject the instance of ActivatedRoute to the constructor of the component that represents the destination route. The instance of the ActivatedRoute will include the passed parameters, as well as the route’s URL segment and other properties. The new version of the component, which renders product detail and is capable of receiving parameters, will be called ProductDetailComponent, which will get an object of type ActivatedRoute injected into it, as shown in the following listing.
Listing 6.8. A fragment from ActivatedRoute
export declare class ActivatedRoute { url: Observable<UrlSegment[]>; queryParams: Observable<Params>; fragment: Observable<string>; data: Observable<Data>; snapshot: ActivatedRouteSnapshot; ... readonly paramMap: Observable<ParamMap>; readonly queryParamMap: Observable<ParamMap>; }
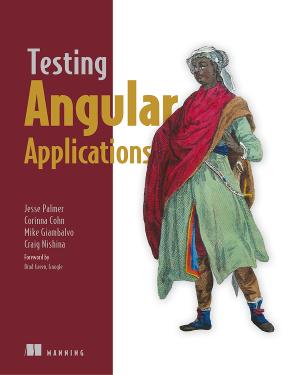
This is an excerpt from Manning's book Testing Angular Applications.
Resolve
—If a user is allowed to activate the attempted route, theresolve
method is used to load data prior to activating the route itself. The data is then available from theActivatedRoute
service in the routed components.
In an Angular application, you implement deep linking through route parameters. The router captures these parameters and makes them available to any component that needs them through the
ActivatedRoute
service. Whenever a user navigates to a different route, theActivatedRoute
service makes information about the route change available to components that use the service.One of the most basic uses of
ActivatedRoute
is to pass along a unique identifier for further content lookup. For example, in the Contacts app, theContactEdit
component usesActivatedRoute
to get the identifier for a contact. When the user navigates tohttp://localhost/edit/1
, the router compares the path to the router configuration and extracts the last part of the path to be used as the value ofid
. After that, the router publishes this value toActivatedRoute
, which sends the update to all subscribing components.The test in this section will use a simplified example of how to test a component that depends on
ActivatedRoute
. Components can subscribe to the values thatActivatedRoute
publishes either as an observable or as a snapshot, an object holding the last updated values for all parameters. Subscribing to an observable is a good choice for a long-lived component that needs to update regularly based on route changes. (See chapter 6, section 6.6.) Using the snapshot is simpler and is a good choice for when a component only needs to use route parameters when it’s constructed. The testing setup for either is similar, but this example will use a snapshot.The example in listing 7.6 is simplified for illustration purposes. Normally, a component for editing data would have a form and controls for modifying and saving the data, but here you’re focusing on loading the Contact ID from the
ActivatedRoute
service and using it in the template.Listing 7.6 Simplified
ContactEdit
component usingActivatedRoute
@Component({ selector: 'contact-edit', template: '<div class="contact-id">{{ contactId }}</div>', #1 }) class ContactEditComponent implements OnInit { private contactId: number; constructor(private activatedRoute: ActivatedRoute) { } #2 ngOnInit () { this.contactId = this.activatedRoute.snapshot.params['id']; #3 } } #1 Shortened template for illustration purposes #2 Injects the ActivatedRoute service during construction #3 Assigns the Contact ID on initialization