concept array in category angular
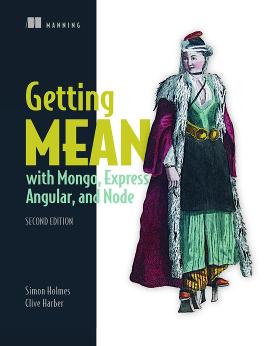
This is an excerpt from Manning's book Getting MEAN with Mongo, Express, Angular, and Node.js 2ED.
In terms of JavaScript data, a repeatable pattern lends itself nicely to the idea of an array of objects. You want one array to hold multiple objects, with each object containing all the relevant information for an individual listing.
Array— An array of a given type of data.
Listing 8.10. Using *ngFor to loop through an array in home-list.component.html
<div class="facilities"> <span *ngFor="let facility of location.facilities" class="badge badge-warning">{{facility}}</span> </div> !@%STYLE%@! {"css":"{\"css\": \"font-weight: bold;\"}","target":"[[{\"line\":1,\"ch\":8},{\"line\":1,\"ch\":52}],[{\"line\":2,\"ch\":17},{\"line\":2,\"ch\":29}]]"} !@%STYLE%@!
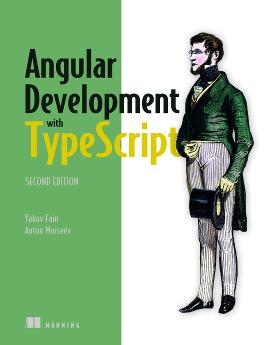
This is an excerpt from Manning's book Angular Development with Typescript, Second Edition.
For example, with the structural *ngFor directive, you can iterate through an array (or other collection) and render an HTML element for each item of the array. The following listing uses the *ngFor directive to loop through the products array and render an <li> element for each product (assuming there’s an interface or Product class with a title property).
In the following code snippet, we pass arrow function expressions as arguments to an array’s reduce() method to calculate a sum, and filter() to print even numbers:
Listing A.5. Rest operator
// ES5 and arguments object function calcTaxES5() { console.log("ES5. Calculating tax for customers with the income ", arguments[0]); // income is the first element // extract an array starting from 2nd element var customers = [].slice.call(arguments, 1); customers.forEach(function (customer) { console.log("Processing ", customer); }); } calcTaxES5(50000, "Smith", "Johnson", "McDonald"); calcTaxES5(750000, "Olson", "Clinton"); // ES6 and rest operator function calcTaxES6(income, ...customers) { console.log(`ES6. Calculating tax for customers with the income ${income}`); customers.forEach( (customer) => console.log(`Processing ${customer}`)); } calcTaxES6(50000, "Smith", "Johnson", "McDonald"); calcTaxES6(750000, "Olson", "Clinton");
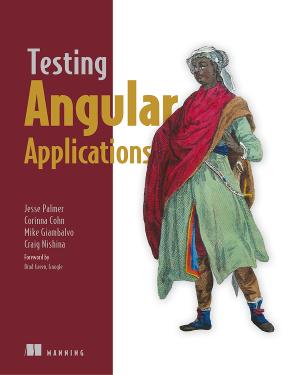
This is an excerpt from Manning's book Testing Angular Applications.
For the last test, you’ll make sure that you can add contacts to the list. To do this, create a new contact using the
Contact
interface and add it to an array calledcontactsList
. Finally, set thecontacts
property ofContactsComponent
to thecontactsList
array that you created. To do this, add the following code after the previous test:
Interacting with a list of elements is similar to interacting with a single element. Finding web elements is asynchronous, whether it’s a single element or a collection, so the result is a promise. A common gotcha is to try to iterate over the collection of web elements with a
for
loop. You can’t loop through a promise, so instead you’ll use the Protractor API methods forelement.all
. For the Contacts app, you can callelement(by.tagName('tbody')).all(by.tagName('tr'))
to get the array of table row web elements. In the following sections, we’ll cover several methods that will help you.
Listing 8.11 Filter for a contact—e2e/contact-list.e2e-spec.ts
import { browser, by, element } from 'protractor'; describe('the contact list', () => { it('with filter: should find existing ' + 'contact "Craig Service"', () => { let tbody = element(by.tagName('tbody')); let trs = tbody.all(by.tagName('tr')); #1 let craigService = trs.filter(elem => { return elem.all(by.tagName('td')).get(1).getText() #2 .then(text => { return text === 'Craig Service'; #3 }); }); expect(craigService.count()).toBeGreaterThan(0); #4 expect(craigService.all(by.tagName('td')) .get(2).getText()) #5 .toBe('craig.services@example.com'); }); }); #1 Finds the array of table rows that represent contacts within the table body #2 Uses the second table column to compare the contact name #3 getText returns a promise of the Boolean evaluation where text === 'Craig Service'. #4 Checks to see if craigService exists #5 As an additional check, verifies that the third column is the correct email address
8.6.2 Mapping the contact list to an array
Let’s consider a different scenario where you need to test all the contacts on the contact list. Instead of writing a filter function for each contact, you could use the
map
function. Themap
function converts the web elements returned from theelement.all
to an array shown in figure 8.6.Figure 8.6 Convert the web elements to an array using the map function
![]()
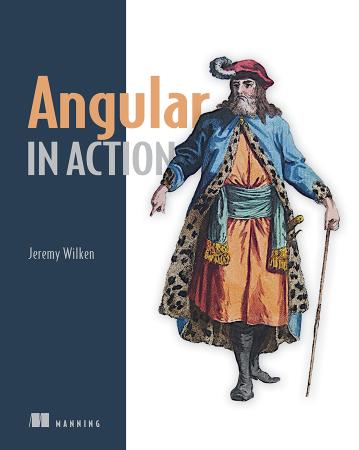
This is an excerpt from Manning's book Angular in Action.
The basic value proposition of TypeScript is it can force restrictions on what types of values variables hold. For example, a variable may only hold a number or it may hold an array of strings. JavaScript has types (don’t let anyone tell you otherwise!), but variables aren’t typed, so you can store any type of value in any variable. This also gave birth to the many types of comparison operators, such as
==
for loose equality or===
for strict equality.
Table 7.1 Route properties used in this chapter and their purposes
Property Accepted values Purpose path
A string, or wildcard matcher ** Defines the URL path to use for a route; it’s appended to any parent paths if routes are nested component
Reference to a component Identifies which component is tied to a particular route redirectTo
A string of another valid route Redirects a user from one route (the path) to the route defined in redirectTo
pathMatch
‘full’, ‘prefix’ Determines the matching strategy, whether to match a partial or full URL for a route children
An array of routes List of routes that are loaded as children of this route outlet
String of a named outlet Tells the route to load in a specific router outlet loadChildren
A string with a path to a module Allows you to lazy load a new module when a particular route is requested canActivate
Array of references to guards Allows you to prevent a route from being used under certain conditions, such as not being logged in