concept Either in category functional programming
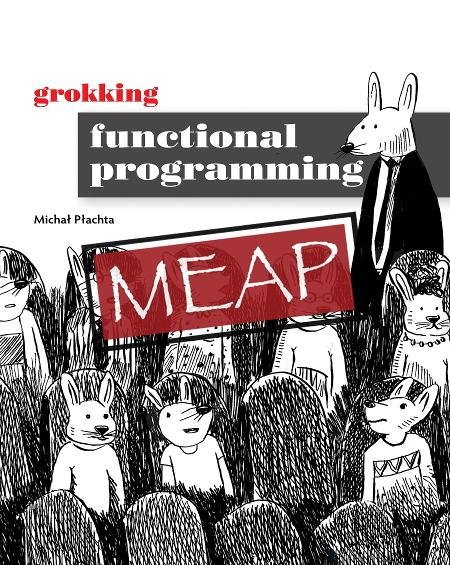
This is an excerpt from Manning's book Grokking Functional Programming MEAP V11.
This new type is called Either, or more specifically Either[A, B]. Just like Option, it has two subtypes:
![]()
As you can see, these data types look pretty similar. Particularly, both Right and Some look identically and it’s not a coincidence, because they are used to convey the information about the “successful case” when these types are used for error indication. The main difference is with the second subtype: None is just that—a single value. Left, however, can “hold” a value, just like Some and Right! So in Either, we can specify a value that will be present in the result for both successful (Right) and error (Left) cases. Let’s see the comparison:
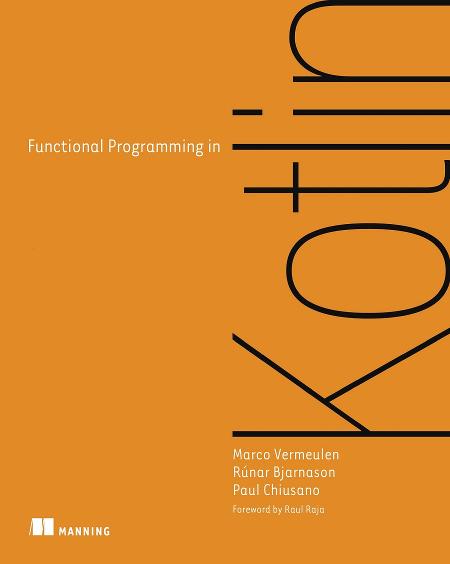
This is an excerpt from Manning's book Functional Programming with Kotlin MEAP V07.
For the same reason that we created our own
List
andTree
data types in chapter 3, we’ll create two Kotlin types,Option
andEither
in this chapter. As before, the types that we are creating are not present in the Kotlin standard library, but are available in Arrow, a supplementary functional companion library to Kotlin. It is worth taking a look at their documentation, which can be found here: arrow-kt.io. The purpose of this chapter is to enhance your understanding of how these types can be used for handling errors. After completing this chapter, you should feel free to use the Arrow version ofOption
andEither
. Even though the semantics of the Arrow versions might differ somewhat, the idea remains the same.
We can craft a data type that encodes whatever information we want about failures. Sometimes just knowing whether a failure occurred is sufficient, in which case we can use
Option
; other times we want more information. In this section, we’ll walk through a simple extension toOption
, theEither
data type, which lets us track a reason for the failure. Let’s look at its definition:Listing 4.5. The
Either
data typesealed class Either<out E, out A> data class Left<out E>(val value: E) : Either<E, Nothing>() data class Right<out A>(val value: A) : Either<Nothing, A>()
Either
has only two cases, just likeOption
. The essential difference is that both cases carry a value. TheEither
data type represents, in a very general way, values that can be one of two things. We can say that it’s a disjoint union of two types. When we use it to indicate success or failure, by convention theRight
constructor is reserved for the success case (a pun on “right,” meaning correct), andLeft
is used for failure. We’ve given the left type parameter the suggestive nameE
(forerror
).[23]
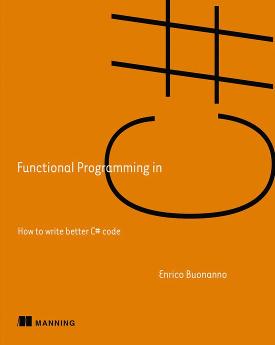
This is an excerpt from Manning's book Functional Programming in C#: How to write better C# code.
There are a couple of obvious shortcomings in the overall solution. First, we’re effectively using Option to stop the computation if something goes wrong along the way, but we’re not giving any feedback to the user as to whether the request was successful or why. In chapter 6, you’ll see how to remedy this with Either and related structures; this allows you to capture error details without fundamentally altering the approach shown here.
A classic functional approach to this problem is to use the Either type, which, in the context of an operation with two possible outcomes, captures details about the outcome that has taken place. By convention, the two possible outcomes are indicated with Left and Right (as shown in figure 6.1), likening the Either-producing operation to a fork: things can go one way or another.
Although Left and Right can be seen in a neutral light, by far the most common use of Either is to represent the outcome of an operation that may fail, in which case Left is used to indicate failure and Right to indicate success. So, remember this:
There are a couple of things to point out here. In all cases, the function is applied only if the Either is Right.[2] This means that if we think of Either as a fork, then whenever we take the left path, we go to a dead end.
2This is what’s called a biased implementation of Either. There are also different, unbiased implementations of Either that aren’t used to represent error/success disjunctions, but two equally valid paths. In practice, the biased implementations are much more widely used.
Write a ToOption extension method to convert an Either into an Option; the left value is thrown away if present. Then write a ToEither method to convert an Option into an Either, with a suitable parameter that can be invoked to obtain the appropriate Left value if the Option is None. (Tip: start by writing the function signatures in arrow notation.) Take a workflow where two or more functions that return an Option are chained using Bind. Then change the first of the functions to return an Either. This should cause compilation to fail. Either can be converted into an Option, as you saw in the previous exercise, so write extension overloads for Bind so that functions returning Either and Option can be chained with Bind, yielding an Option.
Write a function with signature that runs the given function in a try/catch, returning an appropriately populated Either.
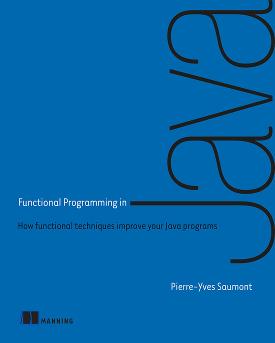
This is an excerpt from Manning's book Functional Programming in Java: How functional techniques improve your Java programs.
Designing a type that can hold either a T or a U is easy. You just have to slightly modify the Option type by changing the None type to make it hold a value. You’ll also change the names. The two private subclasses of the Either type will be called Left and Right.
Now you can easily use Either instead of Option to represent values that could be absent due to errors. You have to parameterize Either with the type of your data and the type of the error. By convention, you’ll use the Right subclass to represent success (which is “right”) and the Left to represent error. But you won’t call the subclass Wrong because the Either type may be used to represent data that can be represented by one type or another, both being valid.
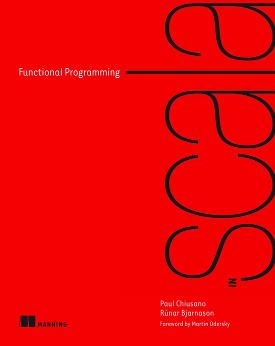
This is an excerpt from Manning's book Functional Programming in Scala.
For the same reason that we created our own List data type in the previous chapter, we’ll re-create in this chapter two Scala standard library types: Option and Either. The purpose is to enhance your understanding of how these types can be used for handling errors. After completing this chapter, you should feel free to use the Scala standard library version of Option and Either (though you’ll notice that the standard library versions of both types are missing some of the useful functions we define in this chapter).
We can craft a data type that encodes whatever information we want about failures. Sometimes just knowing whether a failure occurred is sufficient, in which case we can use Option; other times we want more information. In this section, we’ll walk through a simple extension to Option, the Either data type, which lets us track a reason for the failure. Let’s look at its definition:
Either has only two cases, just like Option. The essential difference is that both cases carry a value. The Either data type represents, in a very general way, values that can be one of two things. We can say that it’s a disjoint union of two types. When we use it to indicate success or failure, by convention the Right constructor is reserved for the success case (a pun on “right,” meaning correct), and Left is used for failure. We’ve given the left type parameter the suggestive name E (for error).[4]
4 Either is also often used more generally to encode one of two possibilities in cases where it isn’t worth defining a fresh data type. We’ll see some examples of this throughout the book.