concept StringBuilder in category java
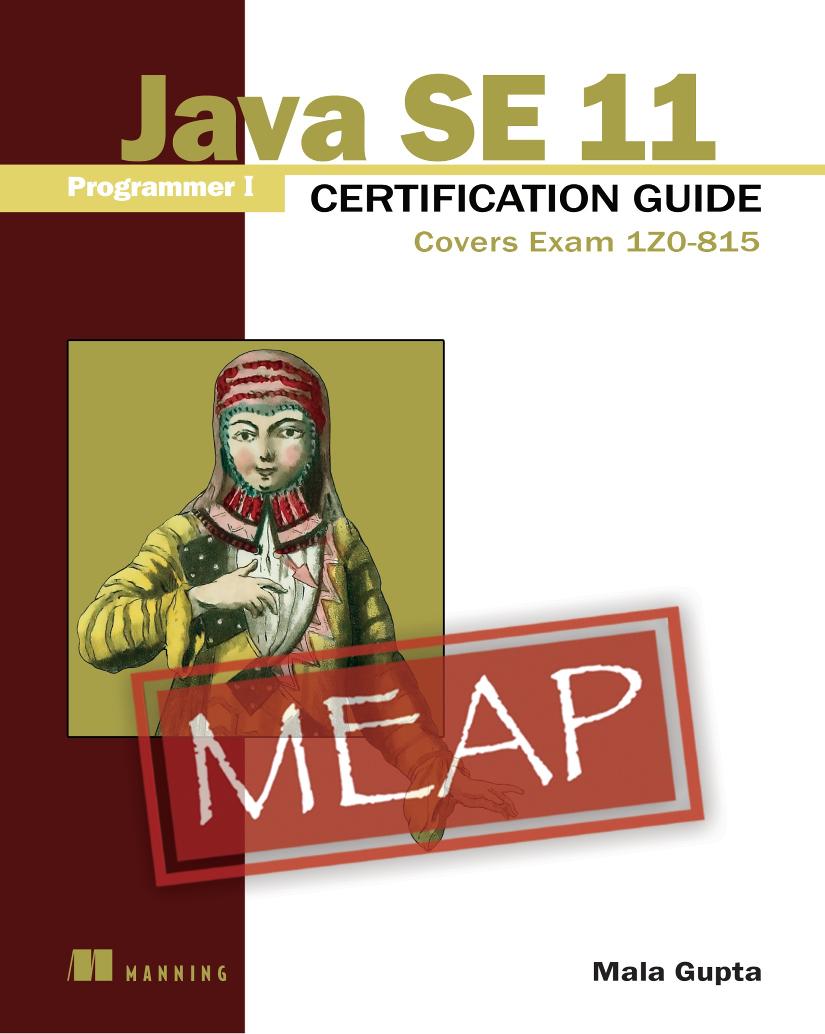
This is an excerpt from Manning's book Java SE 11 Programmer I Certification Guide MEAP V03.
In contrast to the class String, the class StringBuilder uses a non–final byte array to store its value. Following is a partial definition of the class AbstractStringBuilder (the superclass of the class StringBuilder). It includes the declaration of the variables value, coder and count, which are used to store the value of StringBuilder, encoding used to value (either LATIN1 or UTF16) and its length, respectively (the relevant code is in bold):
abstract class AbstractStringBuilder implements Appendable, CharSequence { /** * The value is used for character storage. */ byte value[]; /** * The id of the encoding used to encode the bytes in {@code value}. */ byte coder; /** * The count is the number of characters used. */ int count; //.. rest of the code }This information will come in handy when we discuss the methods of class StringBuilder in the following sections.
Note Similar to the class String, class StringBuilder also uses the concept of Compact Strings. It stores its values using a (mutable) byte[] array and uses an encoder to determine whether it should use one byte per character (for Latin1 values) or two bytes per character for UTF16 values.
You can create objects of the class StringBuilder using multiple overloaded constructors, as follows:
class CreateStringBuilderObjects { public static void main(String args[]) { StringBuilder sb1 = new StringBuilder(); #1 StringBuilder sb2 = new StringBuilder(sb1); #2 StringBuilder sb3 = new StringBuilder(50); #3 StringBuilder sb4 = new StringBuilder("Shreya Gupta"); #4 } }#1 constructs a StringBuilder object with no characters in it and an initial capacity of 16 characters. #2 constructs a StringBuilder object that contains the same set of characters as contained by the StringBuilder object passed to it. #3 constructs a StringBuilder object with no characters and an initial capacity of 50 characters. #4 constructs a StringBuilder object with an initial value as contained by the String object. Figure 5.14 illustrates StringBuilder object sb4 with the value Shreya Gupta.
Figure 5.14 The StringBuilder object with character values and their corresponding storage positions.
![]()
It is interesting to note that when you assign an initial value of say "Shreya Gupta" to a StringBuilder instance, the underlying array used to store it is created with bigger capacity (16 more for LATIN1 values and 32 more for UTF16 values). For the following code:
The classes StringBuffer and StringBuilder offer the same functionality, with one difference: the methods of the class StringBuffer are synchronized where necessary, whereas the methods of the class StringBuilder aren’t. What does this mean? When you work with the class StringBuffer, only one thread out of multiple threads can execute your method. This arrangement prevents any inconsistencies in the values of the instance variables that are modified by these (synchronized) methods. But it introduces additional overhead, so working with synchronized methods and the StringBuffer class affects the performance of your code.
The class StringBuilder offers the same functionality as offered by StringBuffer, minus the additional feature of synchronized methods. Often your code won’t be accessed by multiple threads, so it won’t need the overhead of thread synchronization. If you need to access your code from multiple threads, use StringBuffer; otherwise use StringBuilder.
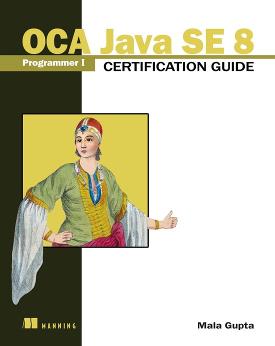
This is an excerpt from Manning's book OCA Java SE 8 Programmer I Certification Guide.
Explanation: The correct way to create an object of class StringBuilder with a default capacity of 16 characters is to call StringBuilder’s no-argument constructor, as follows:
In contrast to the class String, the class StringBuilder uses a non–final char array to store its value. Following is a partial definition of the class AbstractStringBuilder (the superclass of the class StringBuilder). It includes the declaration of the variables value and count, which are used to store the value of StringBuilder and its length, respectively (the relevant code is in bold):
abstract class AbstractStringBuilder implements Appendable, CharSequence { /** * The value is used for character storage. */ char value[]; /** * The count is the number of characters used. */ int count; //.. rest of the code }This information will come in handy when we discuss the methods of class StringBuilder in the following sections.
The classes StringBuffer and StringBuilder offer the same functionality, with one difference: the methods of the class StringBuffer are synchronized where necessary, whereas the methods of the class StringBuilder aren’t. What does this mean? When you work with the class StringBuffer, only one thread out of multiple threads can execute your method. This arrangement prevents any inconsistencies in the values of the instance variables that are modified by these (synchronized) methods. But it introduces additional overhead, so working with synchronized methods and the StringBuffer class affects the performance of your code.
The class StringBuilder offers the same functionality as offered by StringBuffer, minus the additional feature of synchronized methods. Often your code won’t be accessed by multiple threads, so it won’t need the overhead of thread synchronization. If you need to access your code from multiple threads, use StringBuffer; otherwise use StringBuilder.
4.2.2. Creating StringBuilder objects
You can create objects of the class StringBuilder using multiple overloaded constructors, as follows:
![]()
constructs a StringBuilder object with no characters in it and an initial capacity of 16 characters.
constructs a StringBuilder object that contains the same set of characters as contained by the StringBuilder object passed to it.
constructs a StringBuilder object with no characters and an initial capacity of 50 characters.
constructs a StringBuilder object with an initial value as contained by the String object. Figure 4.13 illustrates StringBuilder object sb4 with the value Shreya Gupta.
Figure 4.13. The StringBuilder object with character values and their corresponding storage positions
![]()
When you create a StringBuilder object using its default constructor, the following code executes behind the scenes to initialize the array value defined in the class StringBuilder itself:
![]()
When you create a StringBuilder object by passing it a String, the following code executes behind the scenes to initialize the array value:
![]()
The creation of objects for the class StringBuilder is the basis for the next Twist in the Tale exercise. Your task in this exercise is to look up the Java API documentation or the Java source code to answer the question. You can access the Java API documentation in a couple of ways: