concept cross - cut concern in category .net
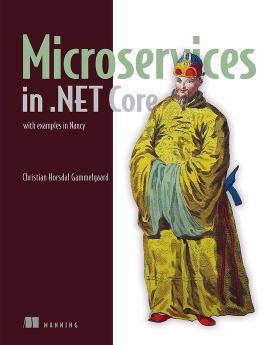
This is an excerpt from Manning's book Microservices in .NET Core: with examples in Nancy.
Throughout the book, you’ll use OWIN middleware to take care of cross-cutting concerns like request logging and monitoring.
None of these components address the cross-cutting concerns mentioned in the introduction to this chapter: monitoring, request logging, and so on. Furthermore, none of these components are good candidates for places to implement those cross-cutting concerns. Why? Because all the components in figure 8.1 implement things specific to the Shopping Cart microservice. In contrast, cross-cutting concerns aren’t specific to any one microservice. Therefore, you need to implement them in components separate from those in figure 8.1. Looking at Shopping Cart from a different angle, in figure 8.2, you see that it gets HTTP requests through a web server, handles them using its various components, and returns responses to the web server, which then sends them back to the caller.
As I just mentioned, you want to keep the code for cross-cutting concerns separated from the components in figure 8.1. Furthermore, cross-cutting concerns apply to the microservice as a whole. This means a good place for the code that handles cross-cutting concerns is between the web server and the endpoint handlers in your Nancy modules (see figure 8.3).
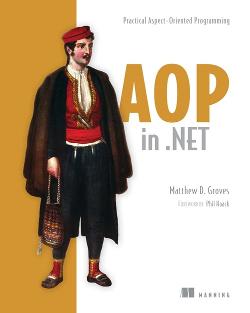
This is an excerpt from Manning's book AOP in .NET.
This decorator (and all the dependencies) are configured with an Inversion of Control (IoC) tool (for example, StructureMap) to be used instead of an InvoiceService instance directly. Now we’re following the open/closed principle by extending InvoiceService to add transaction management without modifying the InvoiceService class. This is a great starting point, and sometimes this approach might be sufficient for a small project to handle cross-cutting concerns.
But consider the weakness of this approach, particularly as your project grows. Cross-cutting concerns are things such as logging and transaction management that are potentially used in many different classes. With this decorator, we’ve cleaned up only one class: InvoiceService. If there’s another class, such as SalesRepService, we need to write another decorator for it. And if there’s a third class, such as PaymentService? You guessed it: another decorator class. If you have 100 service classes that all need transaction management, you need 100 decorators. Talk about repetition!
At some point between decorator 3 and decorator 100 (only you can decide how much repetition is too much), it becomes practical to ditch decorators for cross-cutting concerns and move to using a single aspect. An aspect will look similar to a decorator, but with an AOP tool it becomes more general purpose. Let’s write an aspect class and use an attribute to indicate where the aspect should be used, as in the next example (which is still pseudocode).
I’ll start from scratch and not use any AOP. The business requirements are the most important, so we’ll do those first. Once the business logic is working, we’ll add code to cover the nonfunctional requirements. Once we’ve fulfilled the requirements, we’ll look at possible ways to clean up and refactor the code, again without using any AOP to refactor the cross-cutting concerns.
Once more, add this attribute to your services and remove the exception handling code from inside of them. Now all of the cross-cutting concerns have been refactored. Let’s take a look at the finished product.
Looks good to me. All of the cross-cutting concerns are now in their own classes. The services are back to their initial unspoiled single responsibility state. They’re easier to read.