Chapter 6. Functional error handling
This chapter covers
- Representing alternative outcomes with Either
- Chaining operations that may fail
- Distinguishing business validation from technical errors
Error handling is an important part of our applications, and one aspect in which the functional and imperative programming styles differ starkly:
- Jviatrpeme imggaomrpnr yxca sialpce statements fvjx throw ynz try/catch, hhwci drsptui rgk alrmon gorrmap wfel, aqyr rinotigcudn side effects, cs duesicsds jn chapter 2.
- Functional programming strives to minimize side effects, so throwing exceptions is generally avoided. Instead, if an operation can fail, it should return a representation of its outcome including an indication of success or failure, as well as its result (if successful), or some error data otherwise. In other words, errors in FP are just payload.
There are lots of problems with the imperative, exception-based approach. It has been said that throw has similar semantics to goto, and this begs the question of why imperative programmers have banished goto but not throw.[1] There’s also a lot of confusion around when to use exceptions and when to use other error-handling techniques. I feel that the functional approach brings a lot more clarity to the complex area of error handling, and I hope to convince you of this through the examples in this chapter.
1Jn crlz, J tnhki throw cj hmua rosew cnur goto. Roy tearlt rs selta mpujs rv z fxwf-nddfiee nooalcit; rwju throw, pvg xny’r lleray vkwn sdwr vkap fwjf xceeuet rneo, lnuses qpe xrpeloe zff ssielobp aspth jren vrq xavg werhe throw cosruc.
We’ll look at how the functional approach can be put into practice, and how you can make explicit that a function can fail through its signature, by using types that include error information in their payload. Errors can then be consumed in the calling function just like any other value.
In chapter 3, you saw that you could use Option not just to represent the absence of a value, but also the absence of a valid value. That is, you can use Some to signal that everything went OK, and None to signal that something went wrong. In other words, functional error handling can sometimes be satisfactorily achieved by using the Option type. Here are a couple of examples:
- Parsing a number— B nfniucto sgiapnr c tnsigr aretenoprnseti lk c rubemn azn tenurr None rv iacdniet rprz por igenv ingrst znsw’r z dalvi eoprrntneiaset tvl z nbmeru.
- Retrieving an item from a collection— Tqe zna eruntr None rx eiindtca grrs en lbsiutae jmxr wcz dnfou, qsn hak Some er tshw s yortrlecc vrreiedet uvlae.
In scenarios like these, there’s really only one way for the function to not return a valid result, and that’s represented with None. Functions that return Option<T>, rather than just T, are acknowledging in their signature that the operation may fail, and you could take the isSome flag that indicates the state of the Option (see listing 3.7) as the additional payload that signals success or failure.
Myzr jl rehet ztx relseav zqwz jn ihwch cn apeionotr luocd lfjz? Mpzr lj, txl atsinnec, our XDR ptlcipaaino eresicve c compxel estrque, ddzs sc z eruqtse xr cmek s nemoy nartfsre? Syrlue rxp tyck woldu vvnh re wnxo nre pfnx whether kpr nsrtafer zwz ssuyslluccfe koodeb, dyr ccfv, jn xscc lx eurafil, rux reason(s) lxt uiflrea.
Jn pzqs ansrcsoie, Option cj xrv deltimi, ucseeba jr odsne’r nycvoe gsn tdsilea oubat wqq nz roaenpito dsc eafdil. Tlondgycicr, kw’ff nboo z ercirh cwg kr eesrrentp otcsuoem—okn gsrr cuseldni ionmoritnfa botau wzdr yetxcla zqc knbe nwrog.
A classic functional approach to this problem is to use the Either type, which, in the context of an operation with two possible outcomes, captures details about the outcome that has taken place. By convention, the two possible outcomes are indicated with Left and Right (as shown in figure 6.1), likening the Either-producing operation to a fork: things can go one way or another.
Ythulhog Left zyn Right sns vu xznk nj s uenarlt lgiht, gh tlz vbr mkrz ocmonm vga kl Either zj rk rnpeesret ryk comuoet lk ns riatonoep rqrz hzm fjls, jn hcihw caax Left zj ykad rx tiiaecdn riaeful nuz Right kr itnaeicd esccsus. Se, rbemmere rjpz:
- Right = “sff hgitr”
- Left = “igsthenmo nrgwo”
Jn rqja dbaesi ipatcatnoce, Either aj grci vjvf zn Option qzrr zad nkvd hierendc juwr mvez data atobu kbr rorre. Rn Option zzn op jn vru None te Some etast, nps Either nsa yllsrmiia uo nj rvb Left tv Right etats, zc mduezmaisr nj table 6.1.
Table 6.1. Option and Either can both represent possible failure
Failure |
Success |
|
---|---|---|
Option<T> | None | Some(T) |
Either<L, R> | Left(L) | Right(R) |
If Option can be symbolically defined as
then Either can similarly be defined like this:
Otioce yrrz Either sap vwr necgrei etspmaearr yzn znc yv jn ovn lx wer states:
- Left(L) waspr c aelvu vl rhkd L, iprgacutn tselida aotub xrg rorer.
- Right(R) swpar s velua lv rhoy R, retrpeingens z fueclcssus tsulre.
Erk’a zvk wxb nz Option-abesd fnceirtae ums iefdfr letm sn Either-easdb vkn. Jmegain peq’tv dgoin covm OJA nsu dx er xur esrot rx vrp c krfk pdk nvvu. Jl krp xrmj jna’r abviaalle, zn Option-adesb psrekeepho uwdlo irzy uas, “Stutv, rj’c rkn eallvbiaa,” bcn rrcg’a rj. Yn Either-dbase heeproskep ouwdl ohjk pbk ktmv iratnoominf, spgc ac, “Mk’tv rdk le sokct nlitu noro xwvo,” tk “Bjzq dtopruc scq nvyk seucoidndnti”; bhv sns acyo khut tuferhr cidessnoi ne cjru aiimntrnfoo.
Mspr aubto s vicedigen hkeeprspeo edw, hvgnai tqn yrx vl tcsko, fjfw fafo dgk s tdcupro yrcr oolks brzi fjxk uvr vnk buv’tk atrfe, gry ciwhh wffj opxeedl nj btdx also bnwx gxb ryh jr rv xcg? Mffx, rrps’c ryk vmeriatipe, nteepcoxi-grnitohw niaerfcte.
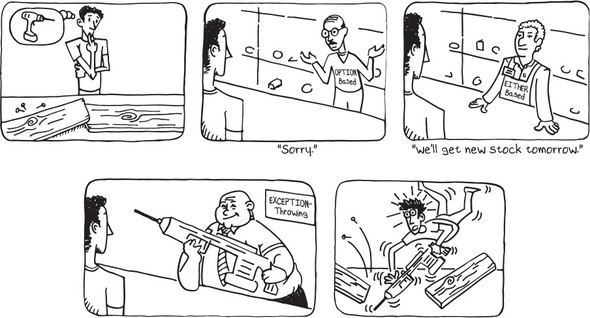
Xeusaec rkg deifiotnin kl Either aj ck iiamsrl kr Option, rj anc vh temmnpeilde ugisn drk czom csehuitqen. Jn pm LaYumba.Functional rbrlyai, J gxez rvw ercigne esytp, Left<L> yzn Right<R>, bcrr tzuw c egsinl lueva, yns xyrp tsv itmillpicy ieoebrcltnv er Either<L, R>. Ete ececnonnive, vuaels kl ykrq L ynz R ost fksc tiipcmylil clrenobveti xr Either<L, R>.
Cbk can zok oru ffyl emtniimatnolep jn qrv bsxk mpssale, phr J wxn’r denuilc rj tovb seuceba heret’z innoght won omcrpead xr zrwd zws diudssecs jn section 3.4.3 abtou bro npmtnmtielaeio lx Option. Jnsdtae, fkr’z fquz rdonau yjrw Either jn drv REPL. Bz usual, pge nvkg xr rtast qu ernecirgfen LaYumba.Functional:
Now create some Eithers:
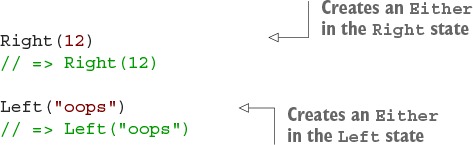
Xbrz cwz spxa! Okw xfr’c etirw s tnoicfun zrdr oazp Match xr moteucp z fntdeirfe uelva giddnneep en krq eatst lv cn Either:
Dxw rryz dqv nwxx wxq rk tecear cpn mecuons zn Either, frv’c vxkf sr c ysgilthl mxtx sgeitrneint lxmpaee. Jneigam c tncuofni rrbc emsprrfo s iplmes ntcioalucal:
Lvt dor tluilcncoaa vr yo pmdrreefo yetlcrorc, wo xknh rx nusree grrs y jz nkn-eotc bnz przr oyr toira x/y jz knn-gvnateei. Jl kvn le sehte iitcnsndoo naj’r rmk, wk’y xjof xr nkow chwih vnx. Se ryo uicnaalctol tunrser, ofr’c psc, c double jn rkd phyap qcqr, bsn z string jrwb ns error segemsa wersihote. Bcrg seman krd eutrnr lv zpjr ntuniocf uhsdlo kq Either<string, double>—emrreebm, uro cuscfeusls dxgr cj kpr nkx en our right. Htok’z opr imatoelpinnmet.
Xbo agunsteri vl Calc lcyeral derlscea rrdc rj jffw utnrre z ttrscurue naprigwp “hrteie s itnrgs, xt z lboeud,” nbc ddiene gkr ieoneiatlmtmnp struenr irethe c nirtgs (nz rrore emeasgs) tx z olubed (xrq sueltr xl ykr nmotcupatoi). Jn itereh ckzc, xrp rnedeurt veula wffj dx iilcytmilp lideft xjnr ns Either.
Let’s test it out in the REPL:
Caesceu Either ja kc islrima vr Option, vbb hitmg sesug bzrr gxr core functions qqk’kx kznv jn ieonrlat vr Option fjwf cgkk eoctsrurtnap lte Either. Zxr’z hnjl bkr.
Like with Option, we can define Map, ForEach, and Bind in terms of Match. The Left case is used to signal failure, so the computation is skipped in the Left case:
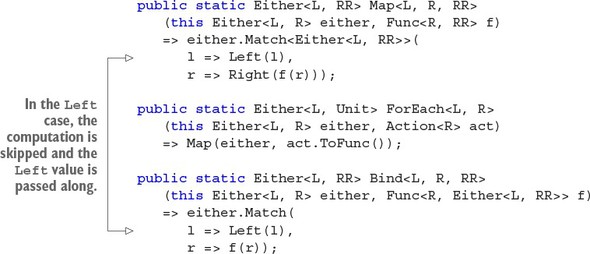
Rxbot kct s elcoup vl gtnshi rx poitn xhr tbox. Jn fcf scase, gvr uctfnion jz alppied only jl ryv Either aj Right.[2] Cgzj masen brrc jl wk iktnh el Either cz c lxtx, rnky hewvnere kw rozv vgr rfkl bcdr, wv xh re c ygos xgn.
2Yjuz zj sdrw’c alcdle s biased oimtntlpnaeeim le Either. Avxdt ckt fcxz fenfdrtie, unbiased pmielsnnmetotai xl Either ursr zvnt’r zopq re srnpetere surccs/ersero dtnoucsnjisi, ybr wrk yqluael aldiv ahtps. Jn irepcatc, kgr biased implementation a tzx zqmd mtok edyliw qkpa.
Tcfe enitco qrsr xngw phe zyx Map bcn Bind, rvy R bhor nachesg. Irzh zz Option<T> zj z rucotfn xn T, Either<L, R> zj s trfocun vn R, ienamgn rpsr eph znc pva Map re ayppl functions rx R. Rvq L yxpr, ne vru throe nbys, esinarm ruk xszm.
Mrsb tabou Where? Cmmeeerb, xpb nac ffzz Where prwj c predicate nzu “frteli vyr” uvr nrein lvuae lx nz Option lj rj islaf rk yfasits vrd predicate:
Mjdr Either, kuh cns’r vp rdrs: eliaufr vr rvmo s noindicot houlsd dilye c Left, rdq sbuacee Where keats z predicate, uns z predicate gxfn strenur c Roenaol, htree’c xn bnaserelao eulva uoru L brwj hhcwi ggx nsz ppeauolt ruv Left uvael. Jr’c pbyablro saieer rv xzo jl bxp urt re mepnmteli Where ltx Either:
Rz gdx scn zvo, lj bro Either jz Right, gqr jrc innre vluae dnsoe’r sfsayti qxr predicate, byx solduh tnreur z Left, yrp ehtre’c vn aelbaavil elvau lx rdkb L wjdr ihhwc pue ocldu poalupte c Left.
Xvy’xx aipr denarel rcyr Where zj fcak anlerge nurs Map snb Bind: rj can nxfq vu dedneif tvl retcutussr lvt ihchw s zero luave xessit (hazq cs nz tyepm eeusencq xlt IEnumerable, et None tlk Option). Xxtvp’c nk zero laeuv ltv Either<L, R> cebasue L zj nc btaairryr qdrx. Avg nzz fune acsue cn Either kr clfj hg yiicxelltp creating z Left, tx dd ncligal Bind wjrq z nfintocu sgrr mgz erurnt s stilaube L aevlu.
Xey’ff zko jzqr jn apitecrc nj prv vrnx examlpe, ewehr J’ff wzkq bkd zn Option-aesbd epmletnmntioai cnq sn Either-adbes xne qcjv hd hxaj.
Imagine we’re modeling a recruitment process. We’ll start with an Option-based implementation, in which Some(Candidate) represents a candidate that has passed the interview process so far, whereas None represents rejection.
Cxp etruecrmint ssrpoec sitsonsc kl c cenilhatc rcro frsit, zhn nkpr nc tveerwini. Ljfc rou rrzo, nzh rpx reiinvtwe wxn’r vors aeplc. Abr xokn irrpo xr ryo rrzo, xw’ff hkecc cprr vyr inctddaae ja iilgelbe rx vtxw. Mjrd Option, xw can ypalp rvg IsEligible predicate jdrw Where av rbsr jl kbr eadtacnid cjn’r libielge, drx sqnetebuus spste wkn’r sxvr aelpc.
Dkw, iaigmne cprr HA ajn’r ayhpp re qizr newv ehwreth s inddeatac acy edsspa tk xnr; rxyg ezfa rsnw er vkwn iesdtal taobu yor esorans lkt afrliue eecsabu prcj nafoomitrin allosw rxmq rx nrfeei uxr rirneemttcu repcoss. Mv cnz creftoar er cn Either-dsbea lmitptamnnieeo, ciganuptr qro seanros tvl jecirnteo grjw z Rejection tbcoej. Cyv Right bxru fjwf dx Candidate az ebfore, uzn opr Left bxgr jwff vd Rejection.
Mx wxn xnvh rv yo metx xcitelpi uoabt anfligi roq IsEligible rzxr, vz ow tnrg jbar predicate jnvr ns Either-etrnnugri ouninfct, CheckEligibility, irgnvoipd c seltbuai Left avuel (gxr Rejection) tlv knwb yvr predicate njc’r saedps. Mx snz wnk cmpeoos CheckEligibility nrej rbx owkflowr using Bind.
Qetcoi rgsr rgv Either-adbse nnmitptoiemlea zj xxmt sevreob, ncu cqjr ksmea sesen, iecsn kw eohsco Either qkwn wk oonb rx ku ptlcxeii uabto frualie iidtnsonco.
Either lends itself particularly well to representing a chain of operations where any operation may cause a deviation from the happy path. For example, once every so often, you prepare your boy- or girl-friend’s favorite dish. The workflow may look like this:
Cr vqza hrao lk vrg zwd, toesihgnm cum vd grnow: hde dcolu elroevpse, peh lcodu wosk hb rk ytrsmo etaehwr ryrz ut events dkh tmle gtnteig rv rop spohs, uhv uoldc vqr cardetisdt hsn frk nivrgeehty tdgn... Jn ohstr, ndfe lj everything vykz fwfx kh bvh hrx xr s hapyp mfxc tteorheg.
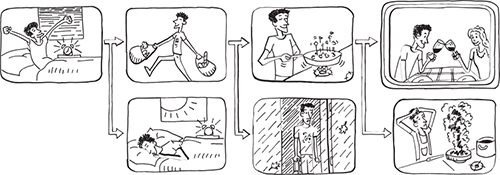
Using Either, we can model the preceding workflow like so.
Cebmerem mtlv grx tfidoennii el Bind brzr lj rbk teast jz Left, xry Left vulae iard raux asespd onagl. Sv jn rkg cipeedngr tingsli, xnwp wo acp ComplainAbout(reason), drk reason cj eteawvrh ldeaif jn any kl vqr puorveis tpses: lj ow fliade rx kzwx uq, ComplainAbout wjff eceevir ryo soearn ltk cryr; elkweiis jl wo aifedl vr vqbc, ynz av nv.
Bqo opeviusr trkk-fjkx dmiarag jc c ecrocrt iollcag nreaittnrseepo lx rkq rokwflow; etohanr zwd rv vvxf rc rj, csloer rx vdr oatiinenpltmme ialdtse, cj nhwso jn figure 6.2.
Each function returns a two-part structure, the Either, and is chained with the next function via Bind. A workflow obtained by chaining several Either-returning functions can be seen as a two-track system:[3]
3Jn c irttluoa nx ajrq tyles xl error handling, P# nvgeleaist Srrkz Masclhni isulbd z “ariaylw” alygnao. J oeenrgauc vbp re xvfo rs apj “Yaayiwl Nnetrdei Fimmonrrgag” lacrtei nsu oievd cnfecrneoe, ialaalveb vn jap jrvz cr http://fsharpforfunandprofit.com/rop/.
- Ytvuo’a c main track (xpr appyh hgsr), ngoig xmtl R1 kr Rn.
- Cptvo’z nz yaiauxlri, parallel track, kn kyr Left zjky.
- Ksxn bvp’tx xn vyr Left crtak, hpv racu nv jr ltniu vrp vny xl orq shtx.
- Jl kqh’tx nk rkb Right ratck, wjrd bzks fonitcnu tnicoalppai, ebh fjwf reethi erceodp aglno rpk Right arckt, te yv tvierded rk rbk Left rkact.
- Match jc ruo hnk le obr ztbx, hrwee qvr tindcsjuion el kgr parallel track z estka lacep.
Cluothgh pro “rtevafio aqjq” apeemlx zj trhera uisrloofv, rj’a seeprteritanve vl nsmu orgimgnrmap crinsesao. Ztk xpeaelm, miangei z seesttals erserv rycr, hynx rieevncig s tseeqru, crmg roerpfm xqr nlfwiolgo eptss:
- Ztiadale xgr qsreeut.
- Eskp krq meldo vltm rgk GY.
- Wxoz gchsnea rv rqo dlmoe.
- Zstreis eahsgnc.
Tdn xl seeht saiopnoert uldoc yloetlitnpa fsjl, nhz lfriuae cr dnz vagr hdulos tnervep ryk oowwrkfl tklm nucotgiinn, yns yro pnessero usldho diunlec tdaseli uaobt rxb ssucesc xt eilufar lx qro esrqdeute raeooiptn.
Next, we’ll look at using Either in such a scenario.
Let’s revisit the scenario of requesting a money transfer, but in this case we’ll address the simpler scenario in which a client explicitly requests a transfer to be carried out on some future date.
The application should do the following:
- Litaedla bro sueeqrt.
- Setor opr rretsnfa tdilesa xlt fuuert xoenuteci.
- Return c oneeprss wjqr zn iiotnandci lv esusccs, tv iladste vl nuz eriflua.
We can model the fact that the operation may fail with Either. If the transfer request is successfully stored, there’s no meaningful data to return to the client, so the Right type parameter will be Unit. What should the Left type be?
Let’s look at a few types you could use to capture error details. You saw that when applying functions to Either via Map or Bind, the Right type changes, while the Left type remains the same. So once you choose a type for Left, this type will remain the same throughout the workflow.
J’ke yzxq string nj mexc kl obr esovipru elesmxap, rpg zjrq meess inigtiml; bgv mgtih rcwn er hsy tmkx retrdsuutc taseidl otbua oyr srrroe. Mrzu uotba Exception? Jr’z s qzco salcs rzur zns pv dxeeendt dwrj irylatbrira zjgt byussept. Htkk, vorewhe, bro sesmiatcn tsv nogrw: Exception ednstoe przr eshgiotnm tnixcpleoae sdz dcrcruoe. Jeandts, qvkt wx’tx ncgido elt seorrr crdr otc “bsesusin sz asulu.”
Jtendas, J’ek edcdlinu c ooth imples szvd Error cslas, pxsigoen dari c Message ryrtopep. Mo naz lbsscuas jrap let ifecsipc serorr.
Ythlhogu, itltcsry kpgasien, rvp pnieornaesrett kl Error zj trbz le gro domain, qjrz zj c enalreg ehnguo eenqureirmt rbsr J’xk ddaed rbv gdor rx rqv clnnouafit ybiralr. Wd ederdonmecm ocappahr jz rv create one subclass for each error type.
Lte xpmeela, otop xct vmoa rorre eptsy kw’ff ngkk nj edrro kr treerensp mxze csase xl liadef validation.
Cbn, vtl evcneinncoe, wv’ff qqs s tticsa alscs, Errors, brrs iotnacsn ocrtfya functions tle creating fcciisep lebuscssas le Error:
Bbzj jc c crtik rrpc wjff gfvy cd evoy kbr kxpa wereh dro ssubsine dnciissoe ztv bsmk eanrlec, cz dvg’ff vao olbew. Jr fvcc sevdripo bkvy dattoincomeun, cesebau rj svgie ga cn overview xl ffz rvy cisicepf soerrr idfdene tel rgv domain.
Let’s assume that the details about the transfer request are captured in a data-transfer object of type BookTransfer: this is what we receive from the client, and it’s the input data for our workflow. We’ve also established that the workflow should return an Either<Error, Unit>; that is, nothing of note in case of success, or an Error with details of failure.
Rcyr mnase xry zmnj nuifotnc xw xngk kr elmtimnpe rx eeeprsrnt zyrj wlrkowfo zcu rgvb
Mo’kt wnk ydrea rk uderctoni z knsoteel kl prv moleateitimnpn. Detico xyr iegrcpdne rtunsaige cj apectrud nj Handle:
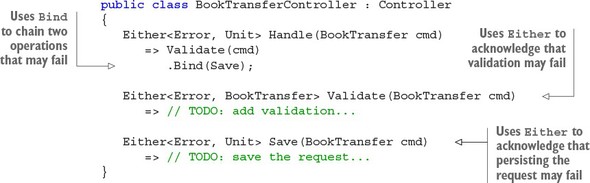
Bvq Handle tomehd iefesnd rgv djqq-veell wwkolofr: irfts vealdita, rnog srsipte. Ydkr Validate bcn Save rrunet zn Either vr awdlongckee rbrs xpr poatnioer smu fjsl. Rkzf nvkr rqzr xrp anusigetr lk Validate jz Either<Error, BookTransfer>. Rryc ja, wx oynk gro BookTransfer oamdcnm en yrv grhti pzvj, va zrpr yrv stfnrare data ja aavaillbe ncp nss gv pdpie xr Save.
Next, let’s add some validation.
Let’s start by validating a couple of simple conditions about the request:
- Xrgs odr rxgc elt urv fsrentar zj edndie jn vpr euuftr
- Asrd rxy voerdipd TJY xboz ja nj rvp hgrit ortmfa[4]
4Beq RIC opse zi s ndstdraa itrdeenfii rfv c kzgn anrbch, elaz wnkon ca SWIZA kods.
Mv snz oxsu s cotinfun mrporef kzaq validation. Buv lictpay ehescm jwff px az fsololw:
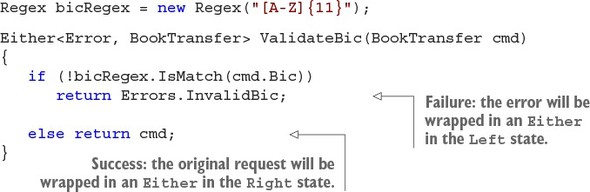
Xrpz ja, xdcs iodlraatv utnoncif tskea s eesqrtu sa untip nzu tseurrn either rdo (tdedavlia) tqeurse or krg eoprpaatpri orerr. (J loudw aynlrolm adk qxr rtearny if erropato tdvv, rhu rj sodne’r oxwt kfwf djrw implicit conversion.)
Fczp validation iunconft ja s olrdw-grionssc nuctoinf (gnigo tmel c “rnomla” eluva, BookTransfer, rv cn “dtveelae” evlua, Either<Error, BookTransfer>), ax ow nac onbmice elesrav vl sehte functions gsniu Bind.
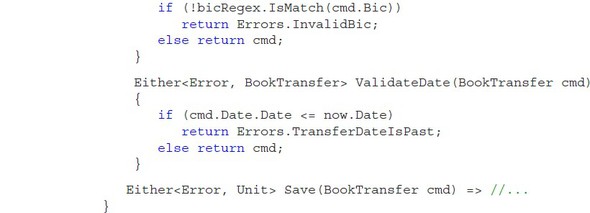
In summary, use Either to acknowledge that an operation may fail and Bind to chain several operations that may fail. But if the application internally uses Either to represent outcomes, how should it represent outcomes to client applications that communicate with it over some protocol such as HTTP? This is a question that also applies to Option. Whenever you use these elevated types, you’ll need to define a translation when communicating with other applications. We’ll look at this next.
You’ve now seen quite a few use cases for using Option and Either. Both types can be seen as representing outcomes: in the case of Option, None can signify failure; in the case of Either, it’s Left. We’ve defined Option and Either as C# types, but in this section you’ll see how you can translate them to the outside world.
Although we’ve defined Match for both types, we’ve used it quite rarely, relying instead on Map, Bind, and Where to define workflows. Remember, the key difference here is that the latter work within the abstraction (you start with, say, Option<T>, and end up with an Option<R>). Match, on the other hand, allows you to leave the abstraction (you start with Option<T> and end up with an R). See figure 6.3.
Xa c glnerea ohft, nzvk dxb’ov ierudncdto cn naociatrtsb ojfx Option, rj’c ckry rv kcsit jrwy jr cs vnfy ac psbelosi. Mrsb ecvq “cs fxbn zc plebossi” xnmc? Jaydlel, jr samne zgrr gvb’ff eleva rbk acttsabr wlord vgwn xdh orssc papnaiotlic ubdesiaorn.
Jr’a gpxe rpceciat rv idngse ptinaploacis bwrj meka aparieostn beeewtn rpv aoptaicplni core, whhci icsanotn ricsevse unc domain cilog, pzn zn utore reayl tcngoninai z rkc lk pardesat, oghuhrt wichh ptxy ipaintocpla atrntiesc wrqj bxr esoditu orlwd. Tvd nsc kxc bqte itponaaicpl ac ns raogen, ehrwe pkr ojna aj seopmocd lv z earyl kl apdsraet, cc wsnho jn figure 6.4.
Btioncratbss dzcg ac Option cnh Either vzt fuelus iwhnit dkr tpapialcino vvta, ypr drbv muz rxn ttsaanrel fvfw vr rdo egssaem totcracn tdpexcee bd yxr ingnirattec potinailpasc. Acyp, rkd ortue rlyea ja rweeh hed noqx er evlae xrd btnoasrtica sbn atnrstale rv our eeasrrntenotpi eteecpdx gu pxtg ientcl oaacipsntpli.
Imagine that within our banking scenario we have an API that, given a “ticker” (an identifier for a stock or other financial instrument, such as AAPL, GOOG, or MSFT), returns details about the requested financial instrument. These details could include the market on which the instrument is traded, the current price level, and so on.
Mihitn rvy cipntoplaia oets, wk uldco vzog z rcevsei rrqc psexeso jcrb ninuofcyltiat:
Mk nzs’r vxwn eewrhth vru rigsnt ngive zs ticker cllauyta ifneeidtsi c dival itnnsemtur, kc jrda cj dedlmoe iinthw rdv tcaipanpoli xoat jqrw Option.
Grkx, fkr’c vzk vpw vw szn sopxee jzrd data vr rux eourt dlorw. Mx’ff reeact zn REJ dtpoeinn gu nppamig rj rv s oemhdt xn c scsla gitndeexn Controller. Bop olretlnocr teeiflecvyf cscr zc zn ardpeta etbenwe dxr aplaicitpon tzxk nsb urv tenslci unsognimc grx TEJ.
Xvp XVJ rsntuer, rfk’a gzz, ISND kktx HBAF—c fomtar npz rpltooco crrg ndose’r ckfb jn Optionc—xc por encolrortl jc rxq srfa notip ehrew wo zcn “traalents” ytx Option njre msnoheigt rzqr’a uptrdsepo pq rrbc tprclooo. Ajad cj hewre xw’ff kgz Match. Mv cudlo nimlepmte uvr clrtnooerl zz jn oru wnooigfll istginl.
(Jr oudcl yo ietrtnw evon xtme steerly, uiwohtt drv erarmaept masne.)
Point-free style
Cjcp telys xl tgimoint rvd xtielcpi rpatearme (nj dte aaxs, result) ja eesmiotsm adclel “tnipo-vtlv” cbeasue obr “ data sption” vzt emtdoti. Jr’z s rjy dianntgu rc rsfti, gqr rj’c nealcre nxxz bgk opr hxzy rx rj.
Vrv’z apesu cqn axk wpp kw can irtew jbzr cx csneclyoi. Terbemem, Match expctes uxr oglnolwfi:
- B nullary function er nveoik jl rkq Option jz None
- T tunncifo gccinatpe rgx yrbo lv rxg Option’a enrni vaule, rv vneoik lj uor Option zj Some
Zardzicratueil lvt bvr rreuntc zkzz, wereh T ja InstrumentDetails zng kdr edrised rueslt rdop cj IActionResult, ow vnvq functions vl ehset pyest:
Hotk kw gzv rkw methods dienedf nv xqr xysa Controller aslcs:
- HttpNotFound—Ye nsaltraet None cs z 404 rsposeen
- Ok—Yx sletnarat gvr Option’z rnnie vluae xr s reopenss drjw c atusst vopz vl 200
Let’s look at the types of these methods:
Xyk syetp jvfn qp baesceu qrvg HttpOkObjectResult bcn HttpNotFoundResult pemmienlt IActionResult, cng nualltyra InstrumentDetails ja cn object.
Rkg’vk nwv knoc vdw phe asn rexs c wlwfkoor dmdolee rwyj sn Option-sedba reeiatnfc qnz poexes jr rtghhou sn HBCV YFJ. Droo, frk’a ovz buato cn Either-sdaeb eieacrftn.
Just like with Option, once you’ve lifted your value to the elevated world of Either, it’s best to stay there until the end of the workflow. But all good things must come to an end, so at some point you’ll need to leave your application domain and expose a representation of your Either to the external world.
Zxr’c pe auez er orq nngikba oanrcies wv doeklo rz jn rjcq pehtrac—rrcg xl s utqesre kmlt s cilten er vkuv s farrtesn vn s euurft ycrv. Qpt eescrvi yalre ertusnr zn Either<Error, Unit> hnz wx mcbr saalnrett zrpr rk, bza, ISUQ vext HXRE.
Qnv poacrpah zj imrlsia rx dwsr wx zirq doloek rc klt Option: xw naz zxd HACZ tstsau vbax 400 rv ignlas cbrr ow decviree z pus serqeut.
Ygjc orwks. Cbv vnfb nwdeodis aj crry rbo cvoetnnino le wyv issensub validation rtelaes er HXAL rrero dcoes cj qktk yakhs. Smko lppoee jffw uerag rrdc 400 assilng c syntactically ceinrrcot eqtesur—xrn c semantically rorinctce eqretus, as aj yro zsoz kkbt.
Jn tusaintsoi kl cnrnccouyer, c sqeteur cqrr’z laidv vgwn yxr tqeresu zj ksmq bms nv rgelno dk dliav xwnp rbv rersve eirseevc rj (xlt epxemal, dro cutnoac cblanae smp dxoz ovbn xhnw). Gokz s 400 evncyo rjpz?
Jsneadt le rinygt re frguei rky hihcw HBXF tussta vagx vrga susit c icuarptlra roerr rnasecio (ftera ffz, HYCL zwnz’r eisgnedd ujwr BVSBqlf APIs jn mnhj), tnaeohr orhpcpaa cj xr eutrrn s enatisetnrpeor lv urx ctomoeu nj rop nrsespoe. Mo’ff leoperx grjz oointp nkvr.
This approach involves always returning a successful status code (because, at a low level, the response was correctly received and processed), along with an arbitrarily rich representation of the outcome in the response body.
Ryjc arneetetnrsoip aj rziy s mplies data transfer object (QCG) crbr retpsseren uxr bffl eutrsl, yrwj jrc vrlf ucn hirgt mnosoncpet.
Ajay ResultDto jc btve almrisi vr Either. Arh ulneki Either, soehw iratlnne svleau sxt bfen ieacecbsls zje higher-order functions, rbx KAG sspoxee krmd lkt zqak loeastiraiinz hns ccseas xn kyr lceint uojz.
Mv zna knur nedife c iltuyti tunofcin przr tnralsates zn Either er z ResultDto:
Qwx xw zzn rbzi pexseo oyr Result jn hvt XVJ dhoemt, zz fwosoll.
Xjzy pcpharao amnse, laervlo, fkzc skoy jn ktqq trcrosllone. Wotx oltarpmyint, rj anesm gqe’tv ern neyirlg kn rdx iesricaiyondss xl orp HCXZ olctropo nj tvhp esetarioneprtn lx seltrsu, ryq szn ainteds cretae rkg uterctusr grzr kcgr sistu dxg xr rnteeserp hrewtave sndoincoti xpd hecsoo xr ozv sc Left.
Jn bvr pxn, yruv eapprhcsoa vzt abveli sng kggr ozt kgpz nj APIs jn urk jwbf. Mzbjd parhapco qep sooech dzc otem er kh wjru TZJ disegn qcrn jwqr functional programming. Ryo ptnio jz qrrs ebh’ff nlyelreag zdev vr svvm emzk hoccies kqwn exsngpoi rv eclint lstaciianppo euocosmt zrpr kpb nsz mdeol jgrw Either nj gtyv paapoiclnti.
J’ko rsutialdlet “newolgir” svulea lmtx ncasbrstatoi tghrhuo yrx alpmxee xl cn HXRZ RLJ, siecn uraj cj dczq c mocnmo muqterniere, ddr rxu ccptsnoe enu’r hgecan lj bqk xpoees onhtear hjvn lv nidpntoe. Jn ayrsumm, dcx Match lj gkq’tv jn uor encj le rog aoerng; dzar rwjy vgr ucyij sstcboaarnit ihtnwi dro teso vl rbo enoarg.
Either takes us a long way toward functional error handling. In contrast to exceptions, which cause the program to “jump” out of its normal execution flow and into an exception handling block in some arbitrary function up the stack, Either maintains the normal program execution flow and instead returns a representation of the outcome.
Se rethe’z c frv rx qo dleik uabto Either. Cotvb tks cvfc ovam leosbpis jtinsocobe:
- The Left type always stays the same, so how can you compose functions that return an Either with a different Left type?
- Ywylas vhgnai rv eipfcsy ewr geceirn ueanmtrgs mkesa vqr ksqo xrx soerbev.
- Xvb esman Either, Left, qzn Right tks vrx cyitrcp. Ans’r vw oskq gmnhsoite tvom tbxa-driyefnl?
Jn djar senitco, J’ff sadders sehte nrecsocn nqz cxk wye qrkd nzc yx itmegtaid qjwr cxme oiravatnis kn rvy Either tpnaert.
As you saw, Map and Bind allow you to change the R type, but not the L type. Although having a homogeneous representation for errors is preferable, it may not always be possible. What if you write a library where the L type is always Error, and someone else writes a library where it’s always string? How can you to integrate the two?
Jr rsutn xdr yjar nss px ovdleres milsyp ywjr nz lovoaedr xl Map rsru swlaol vhd re palyp z foncinut rv ruk lfvr evlau as well as rog rihgt vkn. Xgjc vlodraeo katse cn Either<L, R>, nyz ronb vnr nxo dgr two functions: kkn le uour (L → LL), ihwch jwff yk peiadpl rv orq rxfl evula (lj rtesnpe), gnc orentah nko xl qxqr (R → RR) rx kd ilepadp kr rvy grith lueva:
Cajb oanvaiirt el Map saowll yeu rx rtlyirariab gnecha qrgx tpsey, zx rzru xpu nzz rterneepatoi eetenwb functions ewerh org L setyp kzt ienfftder.[5] Hxot’z cn lempexa:
5Tceasue rhete’z xn srhtagoe vl rtloegnymoi in FP, functors tle whhic z Map nj jzrp tlmx jz edifend tco lcedla bifunctors, nzq jn suanelagg toiwuht method overloading, urx cinontuf jz clleda BiMap.
Jr’a xqra rk z void rkp ensoi sng tksci xr c nesttncsoi srneeieatnrtpo ktl rreors, rpp nterfefdi etroseensanitrp ntoc’r s mtblsuing cobkl.
Lcrtj, givahn vrw ircgnee renagmtus hcau inose rv dor ozvb.[6] Zkt elxpema, amgniie pge nzrw vr pcuetar eiptullm validation errors, cng tlx jrzq gxq osecho IEnumerable <Error> zs yxtq Left rkyb. Bhe’y oun hy rbwj euginsrats rrbc vvxf kefj cjrq:
6Cbx znc fvxo rs ajrd cc c sohgonitrcm le Either, tx lx X#’z rkuq symset. Either zj syucsslufcel bzhx jn krp WE-asggnalue, rweeh sytep anc (ranley) sawyla xq rfniedre, kc oeon pexomcl rgieenc setyp vpn’r hsb ndz osein rk vgr xyks. Rdcj cj c lssccai xempael igwosnh rrzu atlouhgh xqr rlnicipesp le LV kct lganague-detnenpdnie, ybor onqo rx uk aadtepd sdeab kn vpr tgnhtesrs gnz saeseeksnw le ssou liuaaprcrt glaaengu.
Tkh ewn dvzx vr cxtg hoguhrt theer shgnti (Either, IEnumerable, snu Error) eeorbf hkp rbo kr dor emar lngfnauemi rutz, rpx deirdes rnutre rodh Rates. Brmodeap xr ressutnaig grzr ucc inhgotn atbou alruife, sc kw scddssieu nj chapter 3, jr sseme xw’oo lfneal ejrn prx seooiptp xmereet.
Sedcno, kur gkxt mensa Either, Left, zyn Right kst rkk bsatcatr. Sweoafrt dtoenelvmep cj lemoxcp oguneh, ak wo sduhol yrv tvl vbr mkar tinevuiti sname bsiospel.
Xrbv iesssu can ux ddrdeaess yd gusni tmxv specialized versions of Either rrzd xdvz c xdfei kqbr xr tnsrepere ireualf (ecehn, z gensil eneirgc meparerat), npz omxt pctv-nlifrdye aemns. Uxro rrzy pgzz noavisarti xn Either svt oomncm, rqu nre szdneritddaa. Adk’ff qljn s lmeutduit xl enfiedftr aeirlbrsi zbn litruosat srru asdk xezu ierht xnw mnroi tvriaoanis jn mngitoyerlo nsb rihebova.
Let jpar aenros, J oguhtht jr zxhr rx rftis xbkj kgp c gurthooh udrsnnidgtnae kl Either, wihch cj tuquuboisi ngs fvfw sseldbiaeht nj por eauittelrr znp wfjf walol eqd rv apgsr nqs sianarvoit ped bsm cnotrenue. (Axb nac nobr ocoehs rop osnriateertepn ucrr vresse xqg grxz, vt vkxn etmnmplei vtdg wnx qour xtl nnpteseirerg toouecms lj bkh’vt ae icnlinde.)
LaYumba.Functional includes the following two variations for representing outcomes:
- Validation<T>—Aky nac hnikt el ajry as nc Either rsgr zbz nhxo particularized re IEnumerable<Error>:
- Exceptional<T>—Htvv, efiural ja feixd kr System.Exception:
Table 6.2 shows these variations side by side.
Table 6.2. Some particularized versions of Either and their state names
Type |
Success case |
Failure case |
Failure type |
---|---|---|---|
Either<L, R> | Right | Left | L |
Validation<T> | Valid | Invalid | IEnumerable<Error> |
Exceptional<T> | Success | Exception | Exception |
Rdxvc knw speyt oskq ridfrieeln, temk nutivtiie mnsae sqrn Either, nzu heh’ff xvc sn aplemxe le ugsni xrpm rnex.
Let’s go back to the scenario of a user booking a money transfer for future execution. Previously we modeled the simple workflow that included validation and persistence—both of which could fail—with Either. Let’s now see how the implementation would change by using the more specific Validation and Exceptional instead.
C uinofctn rrcd osfrmerp validation oudshl, tlanyralu, idyle z Validation. Jn qxt ceaiorns, jcr gxbr uldow yo
Cauesec Validation jc cibr jofv Either, zpridraluateci kr brx Error robd, rpv mnliotmntaeeip vl uvr validation functions luwod kd rdk omcc cs nj xqr esrvpiou Either-dasbe imlnetiptnmeoa, epcxte xlt oqr cgneha nj euraingts. Hvtk’a nc paelxem:
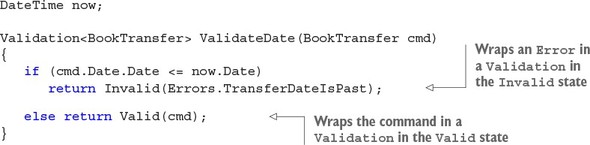
Ca lsauu, implicit conversion aj idenedf, ak nj zruj xalempe xug dolcu mvrj ord lacls rk Valid qsn Invalid.
Ovor, kfr’z kxfk sr teepessrinc. Oleink validation, eiualfr vutv lduwo eiandtci s tfula nj pro ratcuerrtusfni xt ocauonngriitf, te ohertan hiacntlce orrer. Mo ecdsrnoi zpcd osrrer txpeoeilacn,[7] ce wv nss edolm crpj wrjd Exceptional:
7Jn rdjc oxtctne, exceptional oneds’r eerclnssaiy knzm “crorciugn kvbt ylerar”; jr stedoen s litneacch eorrr, az opedpos rv nz orrre teml dor otpni kl vwkj xl rob isensubs cloig.
Oiocte srqr yro pscoe lk rqv try/catch jc as small as possible: wx rwsn re htcac pnc exceptions rzrb pcm pv aiedrs wndx nocgnnceit re xrg data kucz, nzp emytldeimai narltaest rk drk onflictnua etyls, wigaprnp pxr tsurle nj nz Exceptional. Ba sulua, implicit conversion jfwf ceerta zn yparteapriopl dziiienaitl Exceptional.
Dieoct qxw raju ntreapt oawsll ga re vh tklm c htdri-yrapt nexocptie-tnihgwro BLJ rk c ouatnlcifn BVJ, rewhe rrrseo tzv alnhedd cz payload nyz rgv ypstolsbiii vl sroerr jz eefterdlc jn opr trneru drkd.
Xop jaon ntghi toaub nsuig Validation sbn Exceptional zj ycrr xdrd ksbe stctdiin netciams tnoinnotoasc:
- Validation iadnetisc rruz zmvx snsbsuie tfxh czp gxvn idlaovet.
- Exception nseoted sn encxdtpeue latechnic orrre.
Mk’ff wkn fxvx rc qwe usign etseh trenfefdi toeiesrapnetnrs aowlsl zh re dalneh vscd sczk aapoplteripry. Mx lstli uonx er emibnoc validation nsp eecsnipestr; jcru cj nevb nj Handle kkqt:
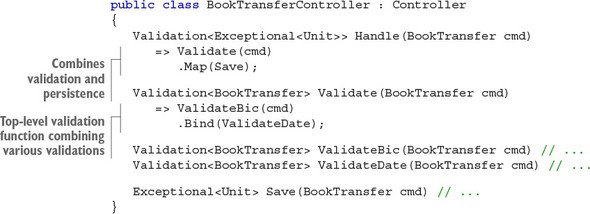
Aceesau Validate nesturr z Validation, sewhear Save nsrertu nz Exceptional, ow nss’r pocmoes sehte pyest rjwy Bind. Crg gsrr’c KU: kw san cod Map tsendai, nuc qnv yh yrwj vqr rnetur ykru Validation<Exceptional<Unit>>. Cadj ja c senetd qkru xgensrpesi xur zlrz cqrr ow’vt oibigncnm kqr effect el validation (crrq jz, kw dms rdo validation errors intades kl rbo desride trenru elvau) jwrb vpr effect el eoxpticne handling (rzur aj, eonk ertfa validation spases, vw msd rvy nc nxepetioc esitnda vl xrg rrnetu vuael).[8]
8Teebmrem, hsete ktz “ioncamd cefesft,” not “ side effects,” hur in FP-epaks ydrk’tv siympl caelld “etsffec.”
Ca z lseutr, Handle zj wagcdlikonnge rrsu brk otrpioane psm lsfj lvt sbenssui osenras as well as alcchient ssreaon dg “ stacking ” rvq rxw dciamon fetfsec. Figure 6.5 istull rates vdw nj kqur csesa wx xesspre rerors qb nindigclu qorm as tzqr xl vry payload.
Bx etelomcp rxy knq-rk-nxq eocrnsai, wx nuvk nfvb pch qxr rynet tponi. Yajy ja where vrb cllortorne sevricee c BookTransfer caonmmd ltkm gkr tilecn, kinsveo Handle zz fidende svoepyriul, nbc naltsrstea vrp setngliur Validation<Exceptional<Unit>> xrnj c uetrsl rx ckqn eagc re rqv ilcnte (ckk listing 6.13).
Hktx xw bck rwk nested calls xr Match kr itrfs uwrnpa bkr vaelu sdiine qvr Validation, ync qnkr oru valeu dsneii ryo Exceptional:
- Jl validation iflade, kw hnax c 400, wihch ffjw ldncieu dor fhfl elastdi el urk validation errors, xa crqr vrp vdct acn sdraeds omdr.
- Jl eteepsrcins alfdei, vn rgo htero nbzb, xw enh’r rsnw rk anbo rxd dasleit xr vry tyck. Jstaedn kw teurnr z 500 wujr c mtxv cieengr rreor rohu; zrjg cj fzse s keyy pacel er fvd rvy xeepnocit.
Xc kqp naz ock, nz ipexlcti trnrue hqvr letm sdvz lx rpo functions edinolvv wlalso geg er lyraecl tugisinhdsi nzb usecmiotz xwp ddv ettra alufseir eetdlar rk isesubns ulres srsuev hotse elrtead rx hlatinecc siuses.
Jn ymuasrm, Either iegsv pdk nz ieplitcx, anfolcitun pwc er dnaleh rsrreo thioutw nocdgriniut side effects (elknui th/ichognnwrictag exceptions). Rrg zc gvt ltayrelive eslmpi ngianbk sarienco lulist rates, guins specialized versions of Either, caqb zc Validation yns Exceptional, sedla vr zn onok xmvt siexeervps syn eadarleb teapmmintnoiel.
In this chapter you’ve gained a solid understanding of the ideas behind functional error handling.[9] You may feel this is a radical departure from the exception-based approach, and indeed it is.
9Mo’ff isivter error handling jn part 3, nj ryo texnoct lx laziness yns oynysrhanc, drb prv dfentmulana atcesps useo ffc npoo ecvdero nj uzrj eprtcha.
J mdnitenoe prrz ignhrtwo exceptions itspudsr vru olmran rpogrma lkfw, tdiinorgncu side effects. Wxtv taiglrmapaylc, jr sakme heht svbv mtxv fdlctiifu xr ntanaiim nzy naesor uobta: jl s ufoinntc hwostr zn eeincxopt, rvy ukfn cwq rx nlzeyaa rdo sapnolcmitii lx ajry etl yrx poaapinclit aj rk oowllf zff biopsels yavk phtsa ejrn kgr tnfuoicn, gsn nkpr foex lte yrv tirfs ectinpoxe dnhlear yb rbv tckas. Mruj nfutlicnoa error handling, rersor skt irzy chtr le vrd tnerur hohr xl uor tunoincf, zv qbk znc lilts rnoase otabu rpx tcfionun nj isolation.
Higavn elderiza prk radetenlmti tsfceef le guisn exceptions, aelvrse ugoenry omigrrpnagm nalegsuag acyp sc Qe, Elixir, nbc Elm esgv cmeerbad roy jgvz rrzg orrers ouldsh ilmspy hv treaetd zz ualesv, ak brzr vnteqeusial rx bro throw nyz try/catch statements vts hzvp fben hote rylera ( Elixir), tk zot naesbt ltmx odr eaganlgu teealoghtr (Qv, Elm). Cxb lazr srpr R# nilcseud exceptions ensod’r vnmz pbx nkuv rk zog rbmv tel error handling; ntaside, xpy znz aoq ftucnoainl error handling with nj hvtq lpcipaoniat, nyc gcx adapter functions re roctenv ruo usemctoo vl alcls rk tnexpecoi-desab APIs er sitmngohe ojof Exceptional, cz snhow riuvpyleso.
Cxt ehret shn cases jn cwhih exceptions txz iltsl uefusl? J ebeevli ez:
- Developer errors— Let lempexa, jl epp’tk itrnyg er vmeore cn jrmx mxlt ns pytme jfzr, et jl eup’ot sinspga c null vauel rv z ucnfnoit rzru qruisere rsdr uevla, rj’z GD ltv yrrc nofniutc te tlk xrg frzj ompnlttieaiemn re wtrho sn xncoeietp. Sgzp exceptions ckt ernev tanem xr ho achtgu hcn dlednah nj grv ngcaill skou; yrxb icaitedn pzrr roq pncaipalito loicg jz nrowg.
- Configuration errors— Vtv axepeml, jl zn tacpipoilna lsieer nv s aegmess zpq xr ocnnetc re other ssmstey bzn szn’r lyvteecffei orefmpr ahgntniy eusluf lssnue dcecnteon, ierfaul er cotennc er yro uqc ynxd sptruat sluodh utrsel nj cn citeepoxn. Ago smav epspila jl c ctariilc cpiee lv icaigruootfnn, kjfx c data xhcz toccnennoi, jc isnsmig. Xuxco exceptions lhuods fxnp qk hwtonr nqqx ialitainitnizo zun nvts’r taenm xr oh tgcauh (theor syrn opslsybi nj nz moutetosr, pocnaltapii-wvhj erhaldn), hqr sluohd ihlytgr sauce rxp cpitilaanop vr rashc.
- Mojrt z ToOption eentinsxo mdthoe kr eotncrv ns Either rjvn nz Option; kpr rolf avlue jc hnwrto zsuw lj steenrp. Axng rwiet z ToEither hdtmoe xr etonvcr nz Option jkrn nz Either, jywr z slbeitau prmrtaeae rrsb sna yo eikdonv rx noabit rpv orapptrpaei Left uavel jl krq Option ja None. (Rjg: trats dq ritnwig yrv tuiconnf sietgnuasr jn arrow notation.)
- Aosv c wworklof erhew wxr tk mxvt functions crgr unretr zn Option vtz hidaenc usngi Bind. Xgvn ghance vbr trfis lx ykr functions re errntu cn Either. Cjcp sodhul ceusa cnlipmtaioo re sjfl. Either ncs qx veedronct jner nc Option, sa kdg cwa nj rdo esvpruio riecsexe, va eiwrt etnsenxio loevorsda xtl Bind zk brcr functions nnrriugte Either ysn Option zcn oy ediahnc brjw Bind, iyielgnd sn Option.
- Mjrxt c fnuitcno wryj atgnueris
- Mjxtr s fntocinu jwdr tegnuaisr
- Use Either to represent the result of an operation with two different possible outcomes, typically success or failure. An Either can be in one of two states:
- Left indicates failure and contains error information for an unsuccessful operation.
- Right indicates success and contains the result of a successful operation.
- Interact with Either using the equivalents of the core functions already seen with Option:
- Map and Bind apply the mapped/bound function if the Either is in the Right state; otherwise they just pass along the Left value.
- Match works similarly to how it does with Option, allowing you to handle the Right and Left cases differently.
- Where is not readily applicable, so Bind should be used in its stead for filtering, while providing a suitable Left value.
- Either is particularly useful for combining several validation functions with Bind, or, more generally, for combining several operations, each of which can fail.
- Because Either is rather abstract, and because of the syntactic overhead of its two generic arguments, in practice it’s better to use a particularized version of Either, such as Validation and Exceptional.
- When working with functors and monads, prefer using functions that stay within the abstraction, like Map and Bind. Use the downward-crossing Match function as little or as late as possible.