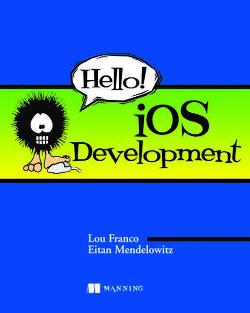
About this book
Part 1 of this book is your introduction to the world of iOS development. By the end of this section, you’ll know your way around Xcode, its GUI building tools, and enough of Objective-C and object-oriented development to build an app:
- Chapter 1 introduces Xcode, the main tool you’ll be using to develop iOS apps. We show you how to write Hello World!, the first app that programmers generally learn to write.
- Chapter 2 explains the model-view-controller pattern used to organize iOS apps.
- Chapter 3 introduces Objective-C so you can add interactivity to your apps. We’ll take Hello World! and show you how to connect up buttons, labels, and text fields to make it more useful.
Part 2 takes you through the main features of iOS as you build three apps. Each chapter will show you all the steps, from sketching a GUI, through object-oriented design, and, finally, how to code the final result:
- Chapter 4 starts with a simple flashcard app that teaches US state capitals. By the end of the chapter, you’ll know how to use outlets and actions to react to the user and simple navigation to get from screen to screen. You can adapt this app for any subject.
- Chapter 5 shows how to polish the look of your app with imagery, custom buttons, and animations.
- Chapter 6 takes the flashcard app and adds a local database using iOS’s Core Data framework. You’ll also learn how to show database information in table views.
- Chapter 7 starts with a new app, Disguisey, that lets you put mustaches, hats, wigs, and other disguise elements onto any photo. In this chapter, we’ll cover tabbed interfaces and accessing the device’s camera or photo album.
- Chapter 8 adds gesture recognition to Disguisey. You’ll learn how to recognize long press, pinch, and pan gestures to interact with your face photo and disguise elements.
- Chapter 9 explores iOS’s location and mapping frameworks in a new app, Parkinator. You’ll learn how to show a map and put a new pin on it to remember where you parked your car.
- Chapter 10 adds networking capabilities to Parkinator. You’ll learn how to show web pages and how to search and post to Twitter.
Part 3 shows you that once you’ve built an app, there’s a lot more to learn. This part guides you around some of the tools that make sure your app doesn’t have bugs and explains how to get the app into the App Store:
- Chapter 11 examines Xcode’s debugger and instruments. You’ll purposely add problems to your completed app and then find them using Xcode’s tools.
- Chapter 12 shows you everything you need to know to get your app into the App Store.
Finally, the appendix provides a list of external resources that will help you make a great app.
In order to follow along with this book, you’ll need to have access to a Mac with the latest Xcode on it (we’ll show you how to get Xcode). This means you must have at least Lion. Most of what we do works on slightly older versions, but the screenshots may not match exactly.
If you want to put any of these apps on your iOS device, you’ll need an iOS developer account, which costs $99 per year. None of the apps in this book require that—you can run all the code in the Simulator. There are parts, like taking a picture with the camera, that we show you how to fake if you aren’t running on a device. If you want to make a real app, you’ll need to join the developer program.
This book contains all the source code for three iOS apps, built up over a few chapters each. Code samples are annotated so you can easily follow along. Code in listings and in text is set in a monospaced font like this to distinguish it from ordinary text.
If you want to download the source, it’s available on GitHub at http://github.com/loufranco/hello-ios-source. The code uses the MIT open source license so you can grab whatever you need for your projects or use any of the example apps as a starting point for your own app. You can also download a zip file with the source code for this book from the publisher’s website at www.manning.com/HelloiOSDevelopment.
Purchase of Hello! IOS Development includes free access to a private web forum run by Manning Publications where you can make comments about the book, ask technical questions, and receive help from the authors and from other users. To access the forum and subscribe to it, point your web browser to www.manning.com/HelloiOSDevelopment This page provides information on how to get on the forum once you’re registered, what kind of help is available, and the rules of conduct on the forum.
Manning’s commitment to our readers is to provide a venue where a meaningful dialogue between individual readers and between readers and the authors can take place. It’s not a commitment to any specific amount of participation on the part of the authors whose contribution to the book’s forum remains voluntary (and unpaid). We suggest you try asking the authors some challenging questions, lest their interest stray!
The Author Online forum and the archives of previous discussions will be accessible from the publisher’s website as long as the book is in print.
LOU FRANCO runs Atalasoft imaging and PDF toolkit development for Kofax and has been a mobile app developer for over a decade. He lives in Northampton, MA.
EITAN MENDELOWITZ is an assistant professor of computing and the arts at Smith College, where he teaches courses situated at the intersection of computer science and media art. These include “Seminar on Mobile and Locative Computing,” which uses iOS as its development platform. Eitan is currently developing mobile platforms to enable citizen science.
In this book
Appendix Online resources for iOS app developers Index List of Figures List of Tables List of Listings